Working with Core Image in iOS: Image Filtering and Processing
Are you looking to enhance your iOS app’s visual appeal by incorporating stunning image filtering and processing capabilities? Look no further than Core Image, Apple’s powerful framework for manipulating images and videos. With Core Image, you can apply a wide range of filters and effects to images with ease, giving your app a professional and polished look. In this guide, we’ll explore how to leverage Core Image to add image filtering and processing features to your iOS app.
Understanding Core Image
Core Image is a high-level framework provided by Apple for processing and manipulating images and videos. It offers a wide range of built-in filters and effects that can be applied to images, as well as support for custom filter creation. Core Image takes advantage of the GPU to perform image processing tasks efficiently, ensuring smooth performance even when applying complex filters to high-resolution images.
Getting Started with Core Image
To start using Core Image in your iOS app, you’ll first need to import the Core Image framework into your project. You can do this by adding the following import statement to your source files:
import CoreImage
Once you’ve imported the framework, you can create instances of the CIImage class to represent images and apply filters to them using the CIFilter class. Let’s take a look at a simple example of how to apply a filter to an image:
guard let image = UIImage(named: "example.jpg"), let ciImage = CIImage(image: image) else { return } let filter = CIFilter(name: "CIPhotoEffectMono") filter?.setValue(ciImage, forKey: kCIInputImageKey) if let outputImage = filter?.outputImage { let filteredImage = UIImage(ciImage: outputImage) // Display or use the filteredImage as needed }
In this example, we load an image named “example.jpg” from the app’s bundle and create a CIImage instance from it. We then create a CIFilter instance with the desired filter name (“CIPhotoEffectMono” in this case) and set the input image using the kCIInputImageKey key. Finally, we retrieve the output image from the filter and create a UIImage instance from it, which can be displayed or used in the app.
Applying Filters and Effects
Core Image provides a wide range of built-in filters and effects that you can apply to images. These filters cover various categories such as color adjustments, blurs, distortions, and stylized effects. Here are a few examples of commonly used filters:
- CIColorControls: Adjusts the brightness, contrast, and saturation of an image.
let filter = CIFilter(name: "CIColorControls") filter?.setValue(ciImage, forKey: kCIInputImageKey) filter?.setValue(1.2, forKey: kCIInputBrightnessKey) filter?.setValue(1.0, forKey: kCIInputContrastKey) filter?.setValue(0.8, forKey: kCIInputSaturationKey)
- CIGaussianBlur: Applies a Gaussian blur effect to the image.
let filter = CIFilter(name: "CIGaussianBlur") filter?.setValue(ciImage, forKey: kCIInputImageKey) filter?.setValue(10.0, forKey: kCIInputRadiusKey)
- CIPhotoEffectChrome: Applies a chrome effect to the image.
let filter = CIFilter(name: "CIPhotoEffectChrome") filter?.setValue(ciImage, forKey: kCIInputImageKey)
Creating Custom Filters
In addition to the built-in filters provided by Core Image, you can also create custom filters using the Core Image Kernel Language. This language allows you to define image processing operations using a syntax similar to C, giving you full control over the filtering process. Custom filters can be created by subclassing the CIFilter class and implementing the necessary kernel function. Check out Apple’s documentation on Core Image Filter Reference for more information on creating custom filters.
Conclusion
In this guide, we’ve only scratched the surface of what’s possible with Core Image in iOS. By leveraging Core Image’s powerful capabilities, you can enhance your app’s user experience with stunning image filtering and processing effects. Experiment with different filters and effects to find the perfect combination for your app’s visual style. With Core Image, the possibilities are endless!
Now that you have a basic understanding of Core Image, why not dive deeper into the world of iOS image processing? Check out these additional resources for further exploration:
- Apple’s Core Image Programming Guide
- Ray Wenderlich’s Core Image Tutorial for iOS
- GitHub – CoreImageFilters
Happy coding, and may your images always be pixel-perfect!
Table of Contents
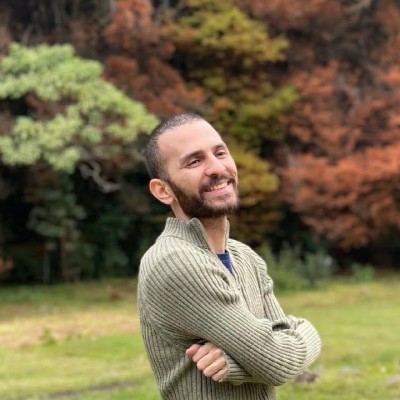
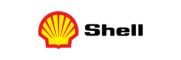