Implementing Core Spotlight in iOS: Making Content Discoverable
In the rapidly evolving world of mobile apps, user engagement and retention are paramount. To achieve these goals, you need to ensure that your app’s content is easily discoverable. This is where Core Spotlight, a powerful iOS framework, comes into play. In this comprehensive guide, we’ll explore how to implement Core Spotlight in your iOS app, allowing users to find relevant content quickly and efficiently.
Table of Contents
1. Why Core Spotlight Matters
Before diving into the technical details, let’s understand why Core Spotlight is essential for your iOS app:
1.1. Improved User Experience
Imagine your app has a vast library of articles, products, or multimedia content. Without an efficient search mechanism, users may struggle to find what they’re looking for. Core Spotlight enables you to provide a seamless and user-friendly search experience.
1.2. Enhanced Engagement
When users can easily access relevant content, they’re more likely to engage with your app regularly. This increased engagement can lead to higher user retention rates, boosting your app’s success.
1.3. Spotlight Integration
Core Spotlight integrates seamlessly with iOS’s built-in Spotlight search, making your app’s content accessible not only within the app but also from the device’s home screen. This can significantly expand your app’s visibility.
Now that we understand the significance of Core Spotlight let’s get into the implementation details.
2. Getting Started with Core Spotlight
To implement Core Spotlight in your iOS app, follow these steps:
2.1. Import the Core Spotlight Framework
Open your Xcode project and ensure that the Core Spotlight framework is imported. If it’s not already imported, you can do this by selecting your project in the Project Navigator, navigating to the “Build Phases” tab, and adding Core Spotlight to the “Link Binary With Libraries” section.
2.2. Create Core Spotlight Items
Core Spotlight relies on the creation of searchable items, each representing a piece of content within your app. These items should include metadata that helps users find what they’re looking for. Here’s a basic example of how to create a Core Spotlight item:
swift import CoreSpotlight import MobileCoreServices func createCoreSpotlightItem(for article: Article) { let attributeSet = CSSearchableItemAttributeSet(itemContentType: kUTTypeText as String) attributeSet.title = article.title attributeSet.contentDescription = article.summary attributeSet.thumbnailData = article.thumbnailImageData let searchableItem = CSSearchableItem(uniqueIdentifier: article.identifier, domainIdentifier: "com.yourapp.articles", attributeSet: attributeSet) CSSearchableIndex.default().indexSearchableItems([searchableItem]) { error in if let error = error { print("Error indexing item: \(error.localizedDescription)") } else { print("Item indexed successfully") } } }
In this example, we create a Core Spotlight item for an article in your app. We set the title, content description, and thumbnail data to provide users with a clear understanding of the article.
2.3. Handling User Interaction
When a user interacts with a Core Spotlight search result, your app should respond appropriately. You can achieve this by implementing the UIApplicationDelegate method application(_:continue:restorationHandler:). Here’s how you can handle user interactions with Core Spotlight items:
swift import UIKit import CoreSpotlight import MobileCoreServices @UIApplicationMain class AppDelegate: UIResponder, UIApplicationDelegate { func application(_ application: UIApplication, continue userActivity: NSUserActivity, restorationHandler: @escaping ([UIUserActivityRestoring]?) -> Void) -> Bool { if userActivity.activityType == CSSearchableItemActionType { if let identifier = userActivity.userInfo?[CSSearchableItemActivityIdentifier] as? String { // Handle the user's interaction with the Core Spotlight item identified by 'identifier'. // You can navigate the user to the relevant content in your app. return true } } return false } }
In this method, you check if the user’s interaction is related to a Core Spotlight item. If it is, you can extract the identifier and use it to navigate the user to the corresponding content in your app.
3. Advanced Usage of Core Spotlight
Now that you have the basics covered, let’s explore some advanced techniques to make the most of Core Spotlight in your iOS app:
3.1. Customizable Searchable Item Attributes
You can customize the searchable item attributes to include additional information that helps users find content more effectively. For instance, you can add keywords, dates, or author information to your Core Spotlight items.
swift attributeSet.keywords = ["iOS", "Swift", "Development"] attributeSet.addedDate = article.publishDate attributeSet.author = article.author
3.2. Updating and Deleting Items
Core Spotlight allows you to update or delete items as your app’s content changes. This ensures that the search index stays up-to-date with the latest content.
To update an item, simply create a new searchable item with the same unique identifier and updated attributes, then call indexSearchableItems with the updated item.
To delete an item, use the deleteSearchableItems(withIdentifiers:) method with the unique identifier of the item you want to remove.
3.3. Grouping Searchable Items
If your app has content organized into categories or sections, you can group searchable items for a more organized search experience. Use the groupIdentifier property of CSSearchableItemAttributeSet to achieve this.
swift attributeSet.groupIdentifier = "Articles"
This groups all items with the same groupIdentifier together in the search results.
4. Best Practices for Core Spotlight Implementation
To ensure a successful Core Spotlight implementation, consider these best practices:
4.1. Optimize Metadata
Make sure that the metadata associated with your searchable items is accurate and descriptive. This will help users find what they’re looking for quickly.
4.2. Keep the Index Updated
Regularly update and delete items to reflect changes in your app’s content. An outdated search index can lead to a frustrating user experience.
4.3. Test Thoroughly
Test your Core Spotlight implementation thoroughly to ensure that it works seamlessly with different types of content and user interactions.
4.4. Monitor Performance
Keep an eye on your app’s performance when using Core Spotlight, especially if you have a large number of searchable items. Performance issues can negatively impact the user experience.
Conclusion
Implementing Core Spotlight in your iOS app is a powerful way to make your content easily discoverable, ultimately leading to improved user engagement and retention. By following the steps outlined in this guide and adhering to best practices, you can enhance your app’s search capabilities and provide a seamless user experience.
Remember that Core Spotlight is just one tool in your arsenal for improving user engagement. Combine it with other techniques, such as intelligent recommendation algorithms and a user-friendly interface, to create an app that users can’t resist.
Incorporating Core Spotlight into your iOS app takes some effort, but the benefits in terms of user satisfaction and app success are well worth it. So, get started today and make your app’s content shine in the spotlight!
Table of Contents
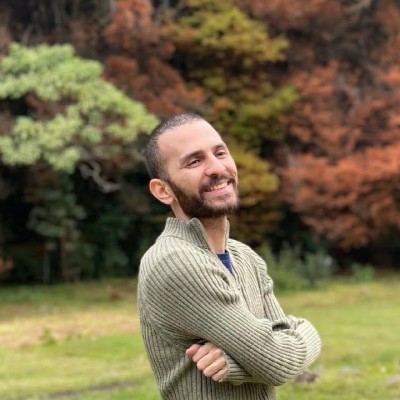
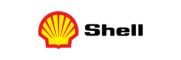