Implementing Touch Gestures in iOS: A Practical Guide
In the world of mobile app development, user experience reigns supreme. Users expect intuitive and responsive interactions, and touch gestures play a crucial role in meeting those expectations. Whether it’s swiping, pinching, tapping, or rotating, touch gestures allow users to engage with your app in a natural and enjoyable way. In this guide, we’ll explore the ins and outs of implementing touch gestures in your iOS app, complete with practical examples and code samples to help you get started.
Table of Contents
1. Understanding the Basics of Touch Gestures
Before we dive into the code, let’s briefly understand the core concepts behind touch gestures. In the context of iOS development, a touch gesture refers to a specific interaction made by the user on the screen of a device. These interactions can be as simple as tapping a button or as complex as swiping multiple fingers to zoom in on an image.
2. Types of Touch Gestures
iOS offers a variety of predefined touch gestures, each serving a distinct purpose:
Tap Gesture: Recognizes a single quick touch on the screen. It’s commonly used for buttons, links, and other UI elements that trigger actions.
swift let tapGesture = UITapGestureRecognizer(target: self, action: #selector(handleTap(_:))) view.addGestureRecognizer(tapGesture)
Swipe Gesture: Detects horizontal or vertical swipes. You can use it to navigate between different views or trigger actions based on the direction of the swipe.
swift let swipeGesture = UISwipeGestureRecognizer(target: self, action: #selector(handleSwipe(_:))) swipeGesture.direction = .right view.addGestureRecognizer(swipeGesture)
Pinch Gesture: Tracks the pinching or zooming motion made by the user. This gesture is useful for implementing features like image zooming.
swift let pinchGesture = UIPinchGestureRecognizer(target: self, action: #selector(handlePinch(_:))) imageView.addGestureRecognizer(pinchGesture)
Rotation Gesture: Detects rotational gestures made by the user. You can utilize this gesture for applications involving rotation-based interactions.
swift let rotationGesture = UIRotationGestureRecognizer(target: self, action: #selector(handleRotation(_:))) view.addGestureRecognizer(rotationGesture)
Pan Gesture: Recognizes dragging or panning motions. It’s ideal for implementing draggable elements within your app.
swift let panGesture = UIPanGestureRecognizer(target: self, action: #selector(handlePan(_:))) draggableView.addGestureRecognizer(panGesture)
3. Implementing Touch Gestures Step by Step
Now that we have a grasp of the various touch gestures, let’s walk through the process of implementing them in your iOS app.
Step 1: Adding Gesture Recognizers
To enable touch gesture recognition, you need to add gesture recognizers to your views. This is typically done in the viewDidLoad method of your view controller.
swift override func viewDidLoad() { super.viewDidLoad() let tapGesture = UITapGestureRecognizer(target: self, action: #selector(handleTap(_:))) view.addGestureRecognizer(tapGesture) let swipeGesture = UISwipeGestureRecognizer(target: self, action: #selector(handleSwipe(_:))) swipeGesture.direction = .right view.addGestureRecognizer(swipeGesture) let pinchGesture = UIPinchGestureRecognizer(target: self, action: #selector(handlePinch(_:))) imageView.addGestureRecognizer(pinchGesture) let rotationGesture = UIRotationGestureRecognizer(target: self, action: #selector(handleRotation(_:))) view.addGestureRecognizer(rotationGesture) let panGesture = UIPanGestureRecognizer(target: self, action: #selector(handlePan(_:))) draggableView.addGestureRecognizer(panGesture) }
In this example, we’re adding different gesture recognizers to the view and an imageView. Make sure to replace handleTap(_:), handleSwipe(_:), etc., with your actual handler methods.
Step 2: Implementing Gesture Handlers
Each gesture recognizer needs a corresponding handler method to perform actions when the gesture is recognized. Here’s a template for implementing gesture handlers:
swift @objc func handleTap(_ gesture: UITapGestureRecognizer) { // Handle tap gesture } @objc func handleSwipe(_ gesture: UISwipeGestureRecognizer) { // Handle swipe gesture } @objc func handlePinch(_ gesture: UIPinchGestureRecognizer) { // Handle pinch gesture } @objc func handleRotation(_ gesture: UIRotationGestureRecognizer) { // Handle rotation gesture } @objc func handlePan(_ gesture: UIPanGestureRecognizer) { // Handle pan gesture }
Step 3: Accessing Gesture Information
Each gesture handler provides access to gesture-specific information. For example, in the pinch gesture handler, you can access the scale property to determine how much the user is pinching:
swift @objc func handlePinch(_ gesture: UIPinchGestureRecognizer) { let scale = gesture.scale // Use the scale value to update your UI }
Step 4: Responding to Gesture States
Gestures have different states, such as began, changed, ended, and failed. You can utilize these states to create smooth and responsive interactions. For instance, in a pan gesture, you can move an object along with the user’s finger:
swift @objc func handlePan(_ gesture: UIPanGestureRecognizer) { let translation = gesture.translation(in: view) switch gesture.state { case .began: // Gesture started, initialize your logic case .changed: // Update UI based on translation draggableView.center.x += translation.x draggableView.center.y += translation.y gesture.setTranslation(.zero, in: view) case .ended: // Gesture ended, finalize your logic default: break } }
4. Fine-Tuning Gesture Recognition
While adding touch gestures is straightforward, ensuring their accuracy and responsiveness might require some fine-tuning. Here are a few tips to consider:
- Gesture Delegate Methods: Gesture recognizers often come with delegate methods that allow you to control their behavior more precisely. For example, you can use the shouldRecognizeSimultaneouslyWith method to handle multiple gestures simultaneously.
- Gesture Thresholds: You can set thresholds for gestures to prevent unintended recognition. For instance, in a pan gesture, you might want to start moving an object only when the user’s finger has moved a certain distance.
- Feedback and Visuals: Provide visual feedback to users when a gesture is recognized. Change the color of a button on tap, or animate an element when it’s being rotated.
- Testing on Real Devices: While simulators are useful, testing your touch gestures on actual devices is crucial to ensure they work as intended.
Conclusion
Incorporating touch gestures into your iOS app can significantly enhance user experience and engagement. By understanding the types of gestures available, implementing gesture recognizers, and handling gesture states, you can create interactive and user-friendly applications. Remember to experiment, test thoroughly, and iterate based on user feedback to provide seamless touch interactions that keep your users coming back for more. Happy coding!
Table of Contents
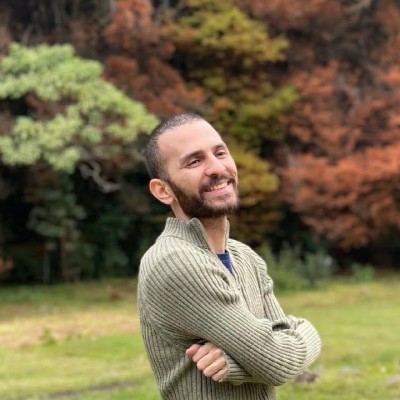
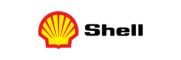