Securing User Data in iOS Apps: Keychain and Data Encryption
In today’s digital landscape, user data privacy and security are paramount concerns for both users and developers. Mobile apps, particularly those running on iOS devices, often handle sensitive user information such as passwords, personal details, and financial data. As a result, implementing robust security measures is crucial to prevent unauthorized access and protect user data from potential breaches.
Table of Contents
1. The Need for Robust Data Security
With the increasing dependency on mobile apps for various tasks, users are becoming more aware of the potential risks associated with sharing personal information online. They expect their data to be handled with the utmost care and security. For app developers, gaining user trust by ensuring the safety of their data is not only a legal and ethical responsibility but also a competitive advantage.
Data breaches can have severe consequences, including financial losses, legal issues, and reputational damage. To mitigate these risks, developers must employ advanced security techniques to safeguard user data. Two fundamental approaches for achieving this in iOS apps are utilizing the Keychain and implementing data encryption.
2. Understanding the Keychain
The iOS Keychain is a specialized secure storage solution provided by Apple. It allows apps to securely store sensitive information such as passwords, encryption keys, and certificates. The Keychain ensures that this information is encrypted and only accessible to the app that originally stored it. Even if the device is compromised, the data stored in the Keychain remains relatively safe.
3. Benefits of Using the Keychain
- Encryption: The Keychain automatically encrypts the stored data using hardware-based encryption mechanisms, ensuring that even if an unauthorized person gains access to the device’s storage, the data remains unreadable.
- Isolation: Each app has its own isolated Keychain, preventing one app from accessing another app’s Keychain data. This isolation enhances security and prevents data leakage between apps.
- Touch ID/Face ID Integration: The Keychain can be unlocked using biometric authentication methods like Touch ID or Face ID, adding an extra layer of protection beyond just a password.
4. Storing Data in the Keychain
Here’s a simple example of how you can store and retrieve data in the Keychain using Swift:
swift import Security // Data to be stored in the Keychain let secretData = "mySecretPassword".data(using: .utf8)! // Define the query for adding data to the Keychain let addQuery: [String: Any] = [ kSecClass as String: kSecClassGenericPassword, kSecAttrAccount as String: "myAccount", kSecValueData as String: secretData ] // Add data to the Keychain let status = SecItemAdd(addQuery as CFDictionary, nil) if status == errSecSuccess { print("Data added to Keychain successfully.") } else { print("Error adding data to Keychain: \(status)") } // Define the query for retrieving data from the Keychain let getQuery: [String: Any] = [ kSecClass as String: kSecClassGenericPassword, kSecAttrAccount as String: "myAccount", kSecMatchLimit as String: kSecMatchLimitOne, kSecReturnData as String: kCFBooleanTrue! ] // Retrieve data from the Keychain var retrievedData: AnyObject? let getStatus = SecItemCopyMatching(getQuery as CFDictionary, &retrievedData) if getStatus == errSecSuccess, let data = retrievedData as? Data { let password = String(data: data, encoding: .utf8) print("Retrieved password: \(password ?? "N/A")") } else { print("Error retrieving data from Keychain: \(getStatus)") }
5. Data Encryption Techniques
Apart from utilizing the Keychain, another vital aspect of securing user data is data encryption. Encryption involves transforming data into a format that is unreadable without a decryption key. This ensures that even if a malicious entity gains access to the encrypted data, they would not be able to decipher it without the proper decryption key.
5.1. Symmetric Encryption
Symmetric encryption uses a single key both for encryption and decryption. While it’s efficient, the challenge lies in securely sharing the encryption key between the sender and the recipient. Here’s a simplified example of symmetric encryption using the CommonCrypto library:
swift import CommonCrypto // The data to be encrypted let originalData = "Sensitive information".data(using: .utf8)! // A 256-bit encryption key let encryptionKey: [UInt8] = [/* Populate with key bytes */] // Initialize buffers for encrypted data var encryptedData = Data(count: originalData.count + kCCBlockSizeAES128) var bytesEncrypted: size_t = 0 // Perform encryption let encryptionStatus = originalData.withUnsafeBytes { dataPointer in encryptedData.withUnsafeMutableBytes { encryptedBytesPointer in CCCrypt( CCOperation(kCCEncrypt), CCAlgorithm(kCCAlgorithmAES), CCOptions(kCCOptionPKCS7Padding), encryptionKey, encryptionKey.count, nil, dataPointer.baseAddress, originalData.count, encryptedBytesPointer.baseAddress, encryptedData.count, &bytesEncrypted ) } } if encryptionStatus == kCCSuccess { encryptedData.count = bytesEncrypted print("Data encrypted successfully: \(encryptedData)") } else { print("Encryption error: \(encryptionStatus)") }
5.2. Asymmetric Encryption
Asymmetric encryption uses a pair of keys: a public key for encryption and a private key for decryption. This eliminates the need to share a single secret key, making it ideal for secure data transmission. Here’s a simplified example using the Security framework:
swift import Security // The data to be encrypted let originalData = "Confidential information".data(using: .utf8)! // Generate a key pair var publicKey, privateKey: SecKey? let keyPairAttributes: [String: Any] = [ kSecAttrKeyType as String: kSecAttrKeyTypeRSA, kSecAttrKeySizeInBits as String: 2048 ] SecKeyGeneratePair(keyPairAttributes as CFDictionary, &publicKey, &privateKey) // Encrypt using the public key var encryptedData: Data? let encryptionStatus = SecKeyEncrypt( publicKey!, .PKCS1, originalData, [], &encryptedData ) if encryptionStatus == errSecSuccess, let encryptedData = encryptedData { print("Data encrypted successfully: \(encryptedData)") } else { print("Encryption error: \(encryptionStatus)") }
Conclusion
In a world where cyber threats are ever-evolving, securing user data in iOS apps is not an option but a necessity. The Keychain and data encryption are powerful tools that developers can leverage to enhance the security of their applications. By implementing these techniques effectively, you can ensure that user data remains confidential and protected from unauthorized access.
Remember, the examples provided in this article are simplified for illustrative purposes. In a real-world scenario, it’s essential to follow best practices, keep up with the latest security updates, and undergo thorough testing to identify and rectify potential vulnerabilities. By prioritizing data security, you contribute to a safer and more trustworthy app ecosystem for users worldwide.
Table of Contents
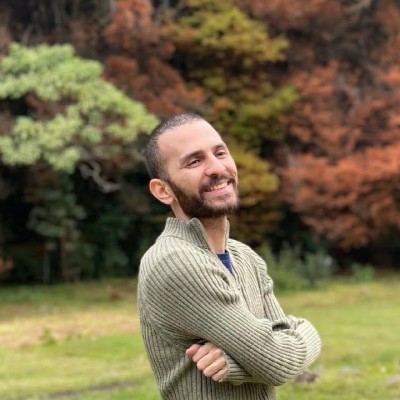
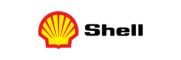