Introduction to Core Animation in iOS: Layer-Based Animations
If you’ve ever marveled at the fluidity of animations in iOS apps, chances are you’ve encountered Core Animation at work. Core Animation is the framework behind the smooth, visually appealing animations that bring life to user interfaces on Apple devices. In this guide, we’ll dive into the fundamentals of Core Animation, focusing specifically on layer-based animations in iOS development.
Understanding Core Animation
At its core, Core Animation is a graphics rendering and animation infrastructure that powers the visual elements of iOS and macOS applications. It operates at a lower level than UIKit, Apple’s primary framework for building user interfaces, providing high-performance rendering capabilities that leverage hardware acceleration for optimal efficiency.
Key Concepts of Core Animation
- Layers: In Core Animation, everything revolves around layers. Layers are lightweight objects representing visual content that can be rendered onscreen. Each UIView in iOS is backed by a layer (CALayer), making it the foundation for building interactive and visually appealing user interfaces.
- Properties and Animatable Attributes: Core Animation enables developers to animate various properties of layers, such as position, size, opacity, and rotation. These properties are animatable by default, allowing for smooth transitions and transformations.
- Implicit and Explicit Animations: Core Animation supports two types of animations: implicit and explicit. Implicit animations are triggered by changes to animatable properties, while explicit animations are explicitly defined by developers using animation objects.
- Timing Functions: Core Animation provides timing functions to control the pacing and acceleration of animations. From linear to ease-in-out curves, developers can customize the timing behavior to achieve the desired animation effects.
Getting Started with Layer-Based Animations
To demonstrate the power of Core Animation, let’s dive into a practical example: animating the position and opacity of a CALayer.
- Creating a CALayer: Start by creating a CALayer instance and adding it to the view’s layer hierarchy.
swift let animatedLayer = CALayer() animatedLayer.frame = CGRect(x: 50, y: 50, width: 100, height: 100) animatedLayer.backgroundColor = UIColor.blue.cgColor view.layer.addSublayer(animatedLayer)
- Animating Properties: Next, define the desired animations using Core Animation APIs. For this example, let’s animate the layer’s position and opacity.
let positionAnimation = CABasicAnimation(keyPath: "position") positionAnimation.fromValue = animatedLayer.position positionAnimation.toValue = CGPoint(x: 200, y: 200) let opacityAnimation = CABasicAnimation(keyPath: "opacity") opacityAnimation.fromValue = 1.0 opacityAnimation.toValue = 0.0 let animationGroup = CAAnimationGroup() animationGroup.animations = [positionAnimation, opacityAnimation] animationGroup.duration = 2.0 animatedLayer.add(animationGroup, forKey: "animation")
- Handling Animation Completion: Optionally, you can implement the animation delegate methods to perform actions upon animation completion.
class AnimationDelegate: NSObject, CAAnimationDelegate { func animationDidStop(_ anim: CAAnimation, finished flag: Bool) { if flag { // Animation completed successfully } } } let delegate = AnimationDelegate() animationGroup.delegate = delegate
Conclusion
Core Animation is a powerful framework for creating fluid and engaging user interfaces in iOS applications. By leveraging layer-based animations, developers can bring their apps to life with smooth transitions and visually appealing effects. Understanding the key concepts and APIs of Core Animation opens up a world of possibilities for crafting immersive user experiences on Apple devices.
Now that you’ve got a taste of Core Animation, dive deeper into its capabilities and explore advanced techniques to take your iOS development skills to the next level.
External Resources
Table of Contents
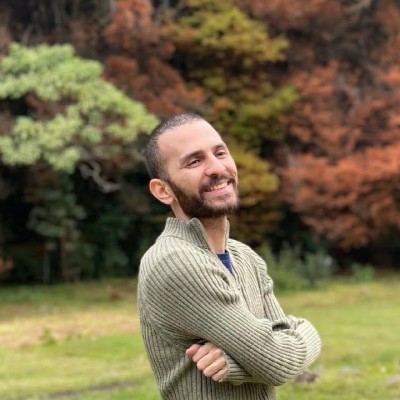
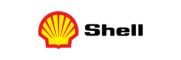