Building Music Playback in iOS: AVFoundation and Media Player Frameworks
Are you an iOS developer looking to integrate music playback into your app? Whether you’re creating a music streaming service, a podcast player, or simply adding background music to your application, understanding how to implement music playback is crucial. In this guide, we’ll explore two key frameworks for handling audio in iOS: AVFoundation and Media Player.
AVFoundation Framework
AVFoundation is a powerful framework provided by Apple for working with audiovisual media in iOS and macOS applications. It offers a wide range of functionalities, including playback, recording, editing, and exporting of audio and video content.
Steps to Integrate Music Playback with AVFoundation:
- Import AVFoundation: Start by importing the AVFoundation framework into your Xcode project.
import AVFoundation
- Set Up Audio Player: Create an instance of AVAudioPlayer to handle the playback of audio files.
var audioPlayer: AVAudioPlayer? func setupAudioPlayer() { guard let soundURL = Bundle.main.url(forResource: "music", withExtension: "mp3") else { return } do { audioPlayer = try AVAudioPlayer(contentsOf: soundURL) audioPlayer?.prepareToPlay() } catch { print("Error loading audio file: \(error.localizedDescription)") } }
- Play Audio: Implement methods to play, pause, and stop the audio playback.
func play() { audioPlayer?.play() } func pause() { audioPlayer?.pause() } func stop() { audioPlayer?.stop() audioPlayer?.currentTime = 0 }
- Handle Playback Events: Utilize AVAudioPlayerDelegate methods to handle playback events such as playback completion or errors.
extension YourViewController: AVAudioPlayerDelegate { func audioPlayerDidFinishPlaying(_ player: AVAudioPlayer, successfully flag: Bool) { // Handle playback completion } func audioPlayerDecodeErrorDidOccur(_ player: AVAudioPlayer, error: Error?) { // Handle decoding errors } }
- Control Playback: Implement user interface controls to allow users to play, pause, and stop the audio playback.
@IBAction func playButtonTapped(_ sender: UIButton) { play() } @IBAction func pauseButtonTapped(_ sender: UIButton) { pause() } @IBAction func stopButtonTapped(_ sender: UIButton) { stop() }
Media Player Framework
The Media Player framework provides a higher-level interface for managing and playing audiovisual media. It offers built-in controls for playback, making it ideal for applications that require standard media playback features.
Steps to Integrate Music Playback with Media Player:
- Import MediaPlayer: Import the MediaPlayer framework into your Xcode project.
import MediaPlayer
- Set Up Media Player: Create an instance of MPMusicPlayerController to handle the playback of media items.
let musicPlayer = MPMusicPlayerController.systemMusicPlayer
- Request Authorization: Request authorization from the user to access their music library.
MPMediaLibrary.requestAuthorization { status in if status == .authorized { // Access granted, proceed with playback } else { // Access denied, handle accordingly } }
- Queue Music: Set the queue of media items to be played by the music player.
func queueMusic() { let mediaQuery = MPMediaQuery.songs() guard let items = mediaQuery.items else { return } musicPlayer.setQueue(with: MPMediaItemCollection(items: items)) }
- Control Playback: Utilize methods provided by MPMusicPlayerController to control playback.
func play() { musicPlayer.play() } func pause() { musicPlayer.pause() } func stop() { musicPlayer.stop() }
Conclusion
In conclusion, integrating music playback into your iOS app is made easy with the AVFoundation and Media Player frameworks provided by Apple. By following the steps outlined in this guide, you can create seamless audio experiences for your users, whether they’re listening to music, podcasts, or any other audio content.
External Resources
For further reading and resources on audio development in iOS, check out the following links:
- AVFoundation Framework Documentation
- Media Player Framework Documentation
- Ray Wenderlich’s Guide to AVFoundation
Happy coding, and may your app’s soundtrack be as delightful as your users’ experiences!
Table of Contents
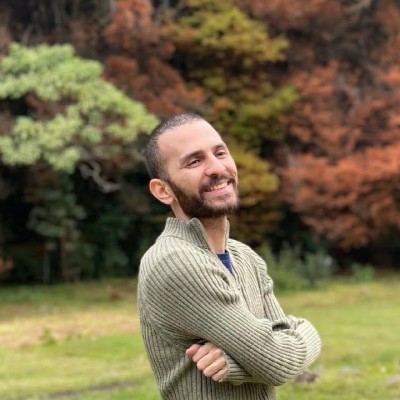
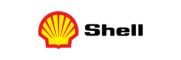