iOS Q & A
What is the best way to organize code in an iOS project?
Organizing code effectively is crucial for maintaining a clean, maintainable, and scalable codebase in iOS app development. Here are some best practices for organizing code in an iOS project:
- Model-View-Controller (MVC) Architecture:
-
-
- Adopt the MVC architectural pattern to separate the application’s concerns into distinct layers: models (data), views (user interface), and controllers (application logic).
- Organize your code into model classes for data representation, view classes for user interface components, and controller classes for business logic and interaction between models and views.
-
- Group by Features or Modules:
Group your code files and resources by features or modules to keep related code together and maintain a clear separation of concerns.
- Create directories or folders for each feature or module in your project structure.
- Place related view controllers, models, views, and other supporting files within the corresponding feature folder.
- Use namespaces or prefixes to prevent naming conflicts between different modules.
- Separate Concerns:
-
-
- Follow the Single Responsibility Principle (SRP) to ensure that each class or component has a single responsibility and does one thing well.
- Split large classes or view controllers into smaller, more manageable components that handle specific tasks or functionalities.
- Delegate responsibilities to specialized classes or objects, such as data managers, networking clients, or view models.
-
- Use Extensions and Categories:
-
-
- Extend existing classes using Swift extensions or Objective-C categories to add additional functionality or behavior.
- Group related methods or computed properties together in extensions to improve code organization and readability.
- Keep extensions and categories organized by feature or functionality to maintain clarity and avoid clutter.
-
- Follow Naming Conventions:
-
-
- Adopt consistent naming conventions for classes, methods, variables, and other identifiers throughout your codebase.
- Use meaningful and descriptive names that accurately reflect the purpose and functionality of each component.
- Follow the Swift or Objective-C naming conventions recommended by Apple and the community for consistency and readability.
-
- Use Modular Design:
-
-
- Break your app into smaller, reusable components or libraries that encapsulate specific functionality or features.
- Use frameworks, packages, or dynamic libraries to create modular, decoupled modules that can be developed, tested, and maintained independently.
- Define clear interfaces and contracts between modules to facilitate communication and integration.
-
- Documentation and Comments:
-
-
- Document your code effectively using inline comments, documentation comments, or README files to provide context, usage instructions, and explanations for other developers.
- Use documentation generators like Javadoc, Doxygen, or SwiftDoc to generate API documentation from your source code comments automatically.
-
- Version Control and Branching Strategy:
-
- Use version control systems like Git and establish a clear branching strategy for managing code changes, feature development, and bug fixes.
- Create feature branches for new development, bug fix branches for addressing issues, and release branches for preparing and deploying releases.
- Follow best practices for committing, merging, and reviewing code changes to maintain code quality and collaboration.
By following these best practices and principles, you can organize your iOS project’s codebase effectively, making it easier to understand, maintain, and scale as your app evolves over time.
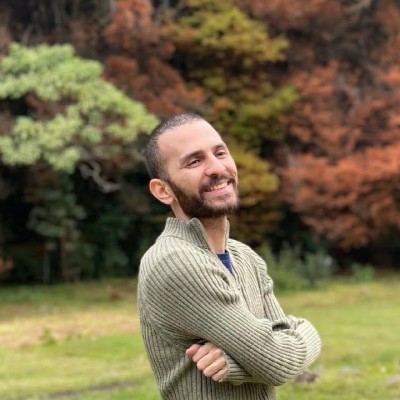
Previously at
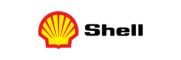
Skilled iOS Engineer with extensive experience developing cutting-edge mobile solutions. Over 7 years in iOS development.