Harnessing the Power of Notifications in iOS Apps
In the ever-evolving landscape of mobile applications, user engagement remains a top priority for developers. Notifications have emerged as a powerful tool to keep users informed, engaged, and connected to their favorite apps. With the rise of iOS devices, Apple’s notification system has become a key component of app interaction. In this blog post, we will delve into the world of iOS app notifications, exploring their types, implementation techniques, and best practices.
1. Understanding the Types of Notifications
Before we dive into the implementation details, let’s first understand the different types of notifications available in iOS apps:
1.1. Local Notifications
Local notifications are triggered by the app itself and don’t require a server. They are perfect for reminding users about tasks, events, or updates within the app. Here’s how you can schedule a local notification using Swift:
swift import UIKit import UserNotifications class ViewController: UIViewController { override func viewDidLoad() { super.viewDidLoad() let content = UNMutableNotificationContent() content.title = "Don't forget!" content.body = "Your appointment is tomorrow." let triggerDate = Calendar.current.dateComponents(hour: 9, minute: 0, second: 0) let trigger = UNCalendarNotificationTrigger(dateMatching: triggerDate, repeats: false) let request = UNNotificationRequest(identifier: "reminder", content: content, trigger: trigger) UNUserNotificationCenter.current().add(request, withCompletionHandler: nil) } }
1.2. Remote Notifications (Push Notifications)
Remote notifications, commonly known as push notifications, are sent from a remote server to the user’s device. They are ideal for sending real-time updates, news, or personalized content. To enable push notifications, you need to configure your app for remote notifications and handle them appropriately:
swift import UIKit import UserNotifications @UIApplicationMain class AppDelegate: UIResponder, UIApplicationDelegate, UNUserNotificationCenterDelegate { func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool { UNUserNotificationCenter.current().delegate = self let options: UNAuthorizationOptions = [.alert, .badge, .sound] UNUserNotificationCenter.current().requestAuthorization(options: options) { (granted, error) in if granted { application.registerForRemoteNotifications() } } return true } // Handle remote notification registration func application(_ application: UIApplication, didRegisterForRemoteNotificationsWithDeviceToken deviceToken: Data) { let token = deviceToken.map { String(format: "%02.2hhx", $0) }.joined() print("Device Token: \(token)") } // Handle remote notification reception func userNotificationCenter(_ center: UNUserNotificationCenter, didReceive response: UNNotificationResponse, withCompletionHandler completionHandler: @escaping () -> Void) { // Handle the notification completionHandler() } }
2. Implementing Notification Strategies
Effective notification strategies can significantly impact user engagement and retention. Here are some strategies to consider when implementing notifications in your iOS app:
2.1. Personalization
Tailor notifications to each user’s preferences and behavior. Collect data on user interactions and preferences to send relevant and timely notifications. For example, a news app can send notifications based on the user’s favorite topics.
2.2. Frequency and Timing
Strike a balance between keeping users informed and avoiding notification fatigue. Allow users to customize the frequency and timing of notifications. Moreover, use features like “Do Not Disturb” hours to respect users’ quiet times.
2.3. Deep Linking
Utilize deep linking to direct users to specific content within your app when they interact with a notification. This enhances user experience by taking them directly to the relevant section, saving them time and effort.
2.4. A/B Testing
Experiment with different notification formats, content, and timings using A/B testing. Analyze the results to refine your notification strategy and optimize user engagement.
2.5. Clear and Concise Content
Craft notification messages that are clear, concise, and compelling. Users should immediately understand the purpose of the notification and its value.
2.6. Rich Media and Interactivity
Incorporate rich media elements such as images, GIFs, or interactive buttons to make notifications visually appealing and engaging. For instance, a messaging app could allow users to reply directly from the notification.
3. Best Practices for Implementing Notifications
Implementing notifications effectively requires following best practices to ensure a seamless user experience:
3.1. Obtain User Consent
Always ask for user consent before enabling notifications. Clearly explain the benefits of enabling notifications and how they enhance the user experience.
3.2. Respect User Preferences
Provide easy-to-access settings where users can manage their notification preferences. Allow them to opt in or out of specific notification types.
3.3. Optimize for Accessibility
Ensure that notifications are accessible to all users, including those with disabilities. Use VoiceOver compatibility and provide alternative text for images.
3.4. Test on Real Devices
Test notifications on real devices to ensure they work as intended and appear correctly across different iOS versions and devices.
3.5. Monitor Performance
Regularly monitor the performance of your notifications. Analyze metrics such as open rates, conversion rates, and user feedback to refine your strategy.
Conclusion
Notifications are a powerful tool for engaging users and keeping them connected to your iOS app. By understanding the types of notifications, implementing effective strategies, and following best practices, you can harness the full potential of notifications to enhance user experience and drive app success. Remember, a well-thought-out notification strategy strikes the right balance between information and engagement, contributing to a positive and lasting impression on your users.
Table of Contents
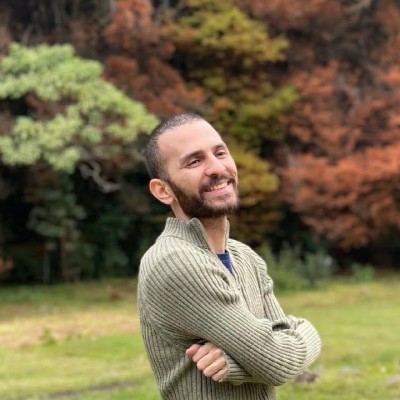
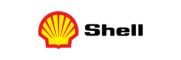