The Role of APIs in iOS Development: Backend Integration Techniques
In the dynamic world of mobile development, a modern application is incomplete without a powerful, reliable backend. From providing real-time updates to managing databases, backend services have become the backbone of mobile applications. In iOS development, Application Programming Interfaces (APIs) serve as the conduit to this backend magic. Understanding this interplay is crucial, especially if you’re looking to hire an iOS developer with adept knowledge in these areas. This blog post will elucidate how to work with APIs in iOS, specifically focusing on integrating backend services, which is a key competency for any iOS developer you aim to hire.
1. What is an API?
An API or Application Programming Interface is a set of protocols, routines, and tools for building software applications. In the context of mobile applications, APIs function as a bridge between the frontend (user interface) and the backend (server or database). They enable applications to communicate with each other and transfer data seamlessly.
When it comes to iOS, there are numerous APIs available. These can be categorized into:
- Apple APIs: These are APIs provided by Apple for developers to access iOS system functionalities. Examples include Core Location for location services, UIKit for UI components, or MapKit for map services.
- Third-Party APIs: These are APIs developed by external companies, allowing access to their data or services. Examples include the Twitter API for accessing Twitter data, Google Maps API for integrating Google Maps, or the OpenWeatherMap API for retrieving weather data.
- Custom APIs: These are APIs created by developers for their specific needs, usually to interact with their own backend services.
This post will concentrate on how to use custom and third-party APIs in an iOS application.
2. Communicating with APIs: HTTP and REST
Most APIs communicate using the HTTP (Hypertext Transfer Protocol) protocol, based on the request-response model. Four common types of HTTP requests are:
– GET: Fetch data from the server
– POST: Send new data to the server
– PUT/PATCH: Update existing data on the server
– DELETE: Remove data from the server
Furthermore, many APIs adhere to the principles of REST (Representational State Transfer). RESTful APIs are stateless, meaning each request from client to server must contain all the information needed to understand and process the request.
3. Integrate APIs in iOS
Now, let’s dive into the practical part of integrating APIs into an iOS app. We’ll use Swift and URLSession, a popular networking API provided by Apple, to perform HTTP requests.
3.1 Making a GET Request
Firstly, let’s see how to make a GET request to retrieve data from a RESTful API. For this, we will use the JSONPlaceholder API which provides dummy data for testing and prototyping.
```swift let url = URL(string: "https://jsonplaceholder.typicode.com/posts")! let task = URLSession.shared.dataTask(with: url) {(data, response, error) in guard let data = data else { return } do { let json = try JSONSerialization.jsonObject(with: data, options: []) print(json) } catch { print("JSON error: \(error.localizedDescription)") } } task.resume() ```
In this example, we create a URL object, then use URLSession’s dataTask method to send a GET request. When the response arrives, it is converted from JSON to a Swift object using JSONSerialization.
3.2 Making a POST Request
Next, let’s create a new record using a POST request.
```swift let url = URL(string: "https://jsonplaceholder.typicode.com/posts")! var request = URLRequest(url: url) request.httpMethod = "POST" let newPost: [String: Any] = ["title": "foo", "body": "bar", "userId": 1] request.httpBody = try? JSONSerialization.data(withJSONObject: newPost, options: []) let task = URLSession.shared.dataTask(with: request) { (data, response, error) in guard let data = data else { return } do { let json = try JSONSerialization.jsonObject(with: data, options: []) print(json) } catch { print("JSON error: \(error.localizedDescription)") } } task.resume() ```
Here, we set the httpMethod of our request to “POST” and assign the JSON-encoded post to httpBody.
3.3 Error Handling
Error handling in network requests is vital. In both the GET and POST examples, we use a guard statement to handle the scenario where we do not receive any data. If there’s an error in the JSON deserialization process, it’s caught and handled by the catch block.
4. Using Third-Party Libraries
While URLSession is powerful and sufficient for many scenarios, third-party libraries like Alamofire and Moya can make working with APIs more manageable and efficient.
4.1 Alamofire
Alamofire is a Swift-based HTTP networking library that simplifies a lot of common networking tasks.
To make a GET request with Alamofire, you can do something like:
```swift AF.request("https://jsonplaceholder.typicode.com/posts").responseJSON { response in if let json = response.value { print("JSON: \(json)") } } ```
As you can see, Alamofire takes care of a lot of the boilerplate code, making the request simpler and more readable.
4.2 Moya
Moya is another popular library built on top of Alamofire. It abstracts the details of networking and provides a more declarative and clean interface. This is particularly useful in large projects or when you have to deal with many different endpoints.
Conclusion
Backend services significantly augment the functionalities of an iOS application, and APIs act as a bridge to these services. They can range from being system-provided to being custom-built or third-party. Swift and its vast ecosystem of libraries provide efficient ways to communicate with these APIs, thereby making it a breeze to integrate robust backend services into your application. This is an essential skill when you plan to hire an iOS developer as it allows for creating more dynamic and interactive applications.
Remember, understanding how to work with APIs is a fundamental skill for any iOS developer. With practice, you will become proficient in leveraging their power to create more feature-rich applications. This proficiency is particularly crucial if you’re in the market to hire an iOS developer.
Hopefully, this article helped you understand how to integrate APIs into your iOS applications and what to look for when you’re ready to hire an iOS developer. Happy coding!
Table of Contents
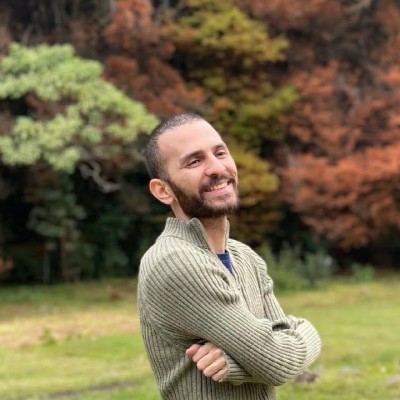
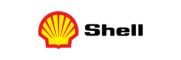