Creating Custom Controls in iOS: UIControl and UIResponder
In the world of iOS development, crafting unique and engaging user interfaces is essential for creating standout apps. While UIKit provides a plethora of pre-built UI elements, there are times when you need to create custom controls to meet specific design requirements or enhance user experience. Two fundamental classes for building custom controls in iOS are UIControl and UIResponder. In this post, we’ll dive deep into these classes, explore their functionalities, and provide practical examples to demonstrate their usage.
Understanding UIControl
UIControl serves as the base class for all controls in UIKit, including buttons, sliders, and switches. It provides essential features for handling user interactions and sending action messages in response to events like touchUpInside, valueChanged, and touchDragInside.
Key Features of UIControl
- Event Handling: UIControl manages touch events and translates them into actions using target-action mechanism.
- State Management: It manages control states such as normal, highlighted, disabled, and selected.
- Customization: Developers can customize appearance and behavior by subclassing UIControl.
Practical Example – Custom Button
Let’s create a custom button using UIControl to demonstrate its usage. Suppose we want to design a button with rounded corners and a gradient background.
import UIKit class CustomButton: UIControl { override var isEnabled: Bool { didSet { // Update appearance based on enabled state backgroundColor = isEnabled ? UIColor.blue : UIColor.lightGray } } override func layoutSubviews() { super.layoutSubviews() layer.cornerRadius = bounds.height / 2 clipsToBounds = true } }
In this example, we subclass UIControl and customize the appearance by overriding layoutSubviews() and isEnabled property.
Learn more about UIControl:
Exploring UIResponder
UIResponder is another crucial class in UIKit, serving as the superclass for objects that respond to and handle events. While UIControl is specialized for creating interactive UI elements, UIResponder is more general-purpose and forms the basis of the responder chain, facilitating event propagation and handling.
Key Features of UIResponder
- Event Handling: UIResponder objects can respond to and handle events such as touch, motion, and remote control events.
- Responder Chain: It defines a hierarchy of objects that can respond to events, enabling event propagation from the first responder to the next responder.
- Touch Handling: UIResponder provides methods like touchesBegan(_:with:) and touchesEnded(_:with:) for handling touch events.
Practical Example – Custom Text Field
Let’s create a custom text field subclassing UIResponder to handle custom text input validation.
import UIKit class CustomTextField: UIResponder { override func touchesBegan(_ touches: Set<UITouch>, with event: UIEvent?) { super.touchesBegan(touches, with: event) // Handle touch event // Example: Show custom keyboard } // Implement custom text input handling }
In this example, we subclass UIResponder to create a custom text field with specialized touch handling behavior.
Learn more about UIResponder:
Conclusion
Custom controls play a vital role in iOS app development, allowing developers to create unique and engaging user interfaces. By leveraging UIControl and UIResponder, you can design interactive and responsive UI elements tailored to your app’s requirements. Whether you’re building a custom button, text field, or any other UI component, understanding these foundational classes is essential for crafting compelling iOS applications.
Start experimenting with custom controls in your iOS projects and unleash your creativity to deliver exceptional user experiences!
External Resources:
- Custom Controls in iOS: A Comprehensive Guide
- Creating Custom UIControls in Swift
- Exploring the Responder Chain in iOS
Happy Coding!
Table of Contents
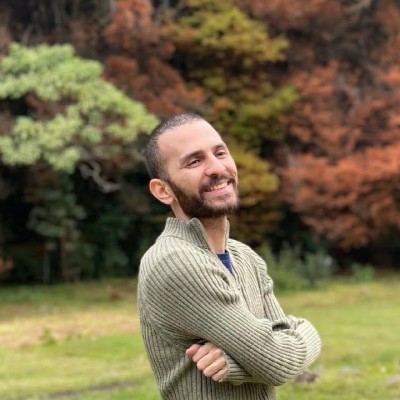
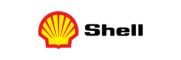