Creating Dynamic User Interfaces with UICollectionView in iOS
In the world of iOS app development, creating visually appealing and interactive user interfaces is crucial for delivering a delightful user experience. Apple’s UIKit framework provides several tools for achieving this goal, and one such powerful tool is the UICollectionView. With UICollectionView, developers can create versatile and dynamic layouts to display collections of items, making it a cornerstone for building engaging and interactive iOS apps.
Table of Contents
1. Introduction to UICollectionView
UICollectionView is a versatile class that provides a flexible and efficient way to present collections of data items using customizable layouts. It is a part of the UIKit framework and is a more advanced alternative to UITableView. UICollectionView enables developers to create complex layouts, such as grids, lists, and more, with support for horizontal and vertical scrolling, section headers and footers, and interactive cells.
2. Key Components of UICollectionView
Before diving into the details of creating dynamic user interfaces using UICollectionView, let’s understand its key components:
- UICollectionViewLayout: This defines the organization and location of all cells and supplementary views inside the collection view. iOS provides a built-in UICollectionViewFlowLayout, but you can also create custom layouts for unique designs.
- UICollectionViewCell: Cells are the building blocks of a UICollectionView. Each cell represents an individual item in the collection and can be customized to display various types of content.
- UICollectionReusableView: These are supplementary views that can be used to display headers, footers, or other custom views within sections of the collection view.
- UICollectionViewDataSource: This protocol is responsible for providing the data and content for the collection view. It defines methods to configure cells and supplementary views.
- UICollectionViewDelegate: The delegate is responsible for handling user interactions, selection, and other events related to the collection view.
3. Creating a Basic UICollectionView
Let’s start by creating a basic UICollectionView with a simple layout. In this example, we’ll build a vertical list of items using the default flow layout.
Step 1: Set up the Collection View
First, create a new project or open an existing one in Xcode. Then, navigate to the desired view controller in the storyboard and add a UICollectionView. Make sure to constrain it properly within the view.
Step 2: UICollectionViewCell Design
Design a custom UICollectionViewCell by creating a new subclass of UICollectionViewCell. This subclass will define the content and layout of each individual cell. For instance, if you’re building a photo gallery, the cell could display an image.
swift class PhotoCell: UICollectionViewCell { @IBOutlet weak var imageView: UIImageView! }
Step 3: UICollectionViewDataSource Implementation
Implement the UICollectionViewDataSource methods in your view controller to provide the data to the collection view.
swift class ViewController: UIViewController, UICollectionViewDataSource { @IBOutlet weak var collectionView: UICollectionView! let photos = ["photo1", "photo2", "photo3", "photo4"] // Names of your photo assets override func viewDidLoad() { super.viewDidLoad() collectionView.dataSource = self } func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int { return photos.count } func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell { let cell = collectionView.dequeueReusableCell(withReuseIdentifier: "PhotoCell", for: indexPath) as! PhotoCell cell.imageView.image = UIImage(named: photos[indexPath.item]) return cell } }
4. Customizing the UICollectionViewLayout
One of the powerful aspects of UICollectionView is the ability to create custom layouts. Let’s explore how to create a grid layout using a custom layout.
Step 1: Create a Custom Layout
Create a new subclass of UICollectionViewLayout to define the custom layout. In this example, we’ll create a simple grid layout.
swift class GridLayout: UICollectionViewLayout { // Implement layout logic here }
Step 2: Override Key Methods
Override the essential methods to configure your custom layout. These methods determine the size and position of cells and supplementary views.
swift class GridLayout: UICollectionViewLayout { // Other methods override func prepare() { // Perform any calculations or setup before layout } override func layoutAttributesForElements(in rect: CGRect) -> [UICollectionViewLayoutAttributes]? { // Calculate layout attributes for elements in the given rect } override func layoutAttributesForItem(at indexPath: IndexPath) -> UICollectionViewLayoutAttributes? { // Calculate layout attributes for a specific item } // Other methods }
Step 3: Integrate the Custom Layout
In your view controller, set the custom layout to your collection view.
swift class ViewController: UIViewController { @IBOutlet weak var collectionView: UICollectionView! override func viewDidLoad() { super.viewDidLoad() let layout = GridLayout() collectionView.collectionViewLayout = layout } // Other methods }
5. Handling User Interaction
UICollectionView also provides robust support for user interaction. You can handle cell selection, highlighting, and other gestures using the UICollectionViewDelegate methods.
swift class ViewController: UIViewController, UICollectionViewDelegate { // Other outlets and methods override func viewDidLoad() { super.viewDidLoad() collectionView.delegate = self } func collectionView(_ collectionView: UICollectionView, didSelectItemAt indexPath: IndexPath) { // Handle cell selection } // Other delegate methods }
Conclusion
UICollectionView is a powerful tool for creating dynamic and interactive user interfaces in iOS applications. Its flexible architecture, customizable layouts, and support for various user interactions make it a versatile choice for displaying collections of data items. By mastering the key components and techniques of UICollectionView, developers can craft engaging and visually appealing apps that provide seamless user experiences. Whether you’re building a photo gallery, a product catalog, or a social media feed, UICollectionView empowers you to design and implement intricate layouts that cater to your app’s unique requirements. So go ahead and explore the capabilities of UICollectionView to take your iOS app development skills to the next level. Happy coding!
Table of Contents
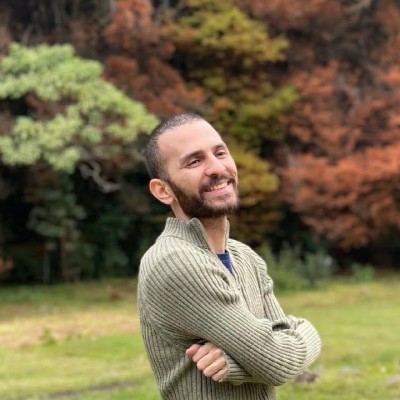
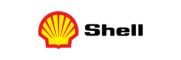