Working with Core Graphics in iOS: Creating Custom Views
When it comes to building engaging and visually appealing iOS applications, having the ability to create custom views is a valuable skill. Apple’s Core Graphics framework provides developers with a powerful set of tools to draw and manipulate graphics at a low level. In this article, we will explore the fascinating world of Core Graphics and learn how to create custom views that can truly elevate your app’s user interface.
1. Understanding Core Graphics
Core Graphics, also known as Quartz 2D, is a powerful framework in iOS that allows developers to work with 2D graphics. It’s used to render images, shapes, and text, and it provides a wide range of capabilities for creating complex and dynamic visual elements. Core Graphics operates at a low level, giving you fine-grained control over every pixel on the screen.
2. Benefits of Custom Views
Creating custom views using Core Graphics offers several benefits. By crafting your own visual elements, you can achieve designs that might be challenging or impossible with pre-built components. Custom views also provide a way to optimize performance by drawing only the necessary elements, resulting in smoother animations and interactions. Moreover, they can encapsulate complex drawing code, promoting reusability and maintainability across your project.
3. Getting Started: Setting Up the Project
To begin working with Core Graphics, create a new Xcode project or open an existing one. For this tutorial, let’s assume we’re building a simple drawing app where users can sketch using their fingers.
3.1 Create a New Custom View Class:
Start by creating a new Swift file in your Xcode project and name it SketchView.swift. In this file, define your custom view class by subclassing UIView. This will be the canvas where users can draw.
swift import UIKit class SketchView: UIView { // We'll implement the drawing code here } Override the draw(_:) Method:
Inside the SketchView class, override the draw(_:) method to provide the drawing implementation. This method is automatically called whenever the view needs to be redrawn, such as when it’s first displayed or when you explicitly call setNeedsDisplay().
swift override func draw(_ rect: CGRect) { super.draw(rect) // Implement your drawing code here }
4. Creating Basic Drawings
Let’s start by drawing some basic shapes on our custom view.
4.1 Drawing a Line:
To draw a line, you can use the UIBezierPath class to define the path of the line and then stroke it. Add the following code inside the draw(_:) method:
swift override func draw(_ rect: CGRect) { super.draw(rect) if let context = UIGraphicsGetCurrentContext() { context.setStrokeColor(UIColor.blue.cgColor) context.setLineWidth(2.0) context.move(to: CGPoint(x: 50, y: 50)) context.addLine(to: CGPoint(x: 200, y: 200)) context.strokePath() } }
4.2 Drawing a Circle:
Drawing a circle follows a similar approach. Use the UIBezierPath class to create a circular path and then stroke or fill it.
swift override func draw(_ rect: CGRect) { super.draw(rect) if let context = UIGraphicsGetCurrentContext() { context.setFillColor(UIColor.red.cgColor) let circlePath = UIBezierPath( arcCenter: CGPoint(x: 150, y: 150), radius: 50, startAngle: 0, endAngle: CGFloat.pi * 2, clockwise: true ) context.addPath(circlePath.cgPath) context.fillPath() } }
5. Implementing User Interaction
Our drawing app won’t be complete without allowing users to actually draw on the canvas. To achieve this, we’ll use touch events to capture the user’s input and render it on the screen.
5.1 Tracking Touch Events:
Override the touchesBegan(_:with:), touchesMoved(_:with:), and touchesEnded(_:with:) methods in the SketchView class to track user touch events.
swift class SketchView: UIView { private var path = UIBezierPath() override func touchesBegan(_ touches: Set<UITouch>, with event: UIEvent?) { if let touch = touches.first { path.move(to: touch.location(in: self)) } } override func touchesMoved(_ touches: Set<UITouch>, with event: UIEvent?) { if let touch = touches.first { path.addLine(to: touch.location(in: self)) setNeedsDisplay() } } override func touchesEnded(_ touches: Set<UITouch>, with event: UIEvent?) { if let touch = touches.first { path.addLine(to: touch.location(in: self)) setNeedsDisplay() } } // The draw(_:) method remains the same }
6. Customizing the Drawing Experience
You can enhance the drawing experience by allowing users to choose different colors, brush sizes, and more. Here’s a basic implementation:
6.1 Adding Color and Brush Size:
Add properties to the SketchView class for the current drawing color and brush size. Update the touch event methods to apply these properties to the drawing context.
swift class SketchView: UIView { private var path = UIBezierPath() var strokeColor: UIColor = UIColor.black var strokeWidth: CGFloat = 2.0 override func draw(_ rect: CGRect) { super.draw(rect) if let context = UIGraphicsGetCurrentContext() { context.setStrokeColor(strokeColor.cgColor) context.setLineWidth(strokeWidth) // Rest of the drawing code remains the same } } // Touch event methods remain the same }
6.2 Adding Clear Functionality:
Allow users to clear the canvas by adding a clear button. In your view controller, you can connect a button action to the clearCanvas method in your SketchView instance.
swift @IBAction func clearButtonTapped(_ sender: UIButton) { sketchView.clearCanvas() } Inside the SketchView class: swift Copy code func clearCanvas() { path.removeAllPoints() setNeedsDisplay() }
Conclusion
Core Graphics provides a powerful toolkit for creating custom views and implementing complex graphics in your iOS applications. By understanding the fundamentals of drawing paths, using touch events, and customizing the drawing experience, you can create engaging and interactive user interfaces that stand out. As you continue to explore Core Graphics, you’ll discover even more possibilities for turning your app’s visual design ideas into reality. So go ahead and experiment with custom views and unleash your creativity in the world of iOS development!
Table of Contents
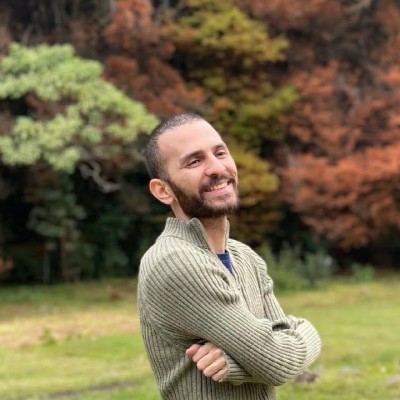
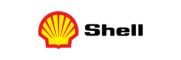