Working with Core Spotlight in iOS: Indexing App Content for Search
Mobile apps have become an integral part of our daily lives, offering a wide range of functionalities and features. One crucial aspect of a successful mobile app is its ability to provide users with a seamless and efficient way to access information within the app. This is where search functionality plays a vital role. In iOS, Core Spotlight is a powerful framework that allows you to index your app’s content and make it easily searchable. In this blog post, we will explore how to work with Core Spotlight to index app content for search, including code samples and best practices.
Table of Contents
1. Why Core Spotlight?
Before we dive into the technical details, let’s briefly understand why Core Spotlight is a valuable tool for iOS app development.
1.1. Enhanced User Experience
Implementing search functionality in your app enhances the overall user experience. Users can quickly find the content they are looking for, which increases user engagement and satisfaction.
1.2. Content Discoverability
Core Spotlight makes your app’s content more discoverable. Even if users are not familiar with the app’s navigation, they can still access specific content by searching for it.
1.3. Cross-App Integration
Core Spotlight integrates seamlessly with other iOS features like Siri and Spotlight search, providing users with a consistent search experience across the entire platform.
Now that we understand the importance of Core Spotlight, let’s dive into the steps to index your app’s content for search.
2. Getting Started with Core Spotlight
2.1. Set Up Your Xcode Project
To begin, open your Xcode project or create a new one if you haven’t already. Core Spotlight requires iOS 9.0 or later, so ensure that your project targets an appropriate iOS version.
2.2. Import Core Spotlight Framework
In your Swift or Objective-C source code file, import the Core Spotlight framework using the following line:
swift import CoreSpotlight
2.3. Create Searchable Items
To make your app’s content searchable, you need to create searchable items. These items represent individual pieces of content in your app, such as articles, products, or user profiles.
swift func createSearchableItem() { let searchableItem = CSSearchableItem(uniqueIdentifier: "article123", domainIdentifier: "com.yourapp.articles", attributeSet: searchableAttributes()) CSSearchableIndex.default().indexSearchableItems([searchableItem]) { error in if let error = error { print("Error indexing searchable item: \(error.localizedDescription)") } else { print("Searchable item indexed successfully") } } } func searchableAttributes() -> CSSearchableItemAttributeSet { let attributes = CSSearchableItemAttributeSet(itemContentType: kUTTypeText as String) attributes.title = "How to Use Core Spotlight" attributes.contentDescription = "Learn how to harness the power of Core Spotlight in iOS." attributes.keywords = ["Core Spotlight", "iOS", "Search"] // Add more attributes as needed return attributes }
In the code snippet above, we create a searchable item with a unique identifier and assign it to a specific domain identifier. The searchableAttributes function defines the attributes associated with the searchable item, such as title, content description, and keywords.
2.4. Index Searchable Items
Once you have created your searchable items, you can index them using the CSSearchableIndex class. The indexSearchableItems method allows you to index one or more searchable items, making them available for search.
2.5. Handle User Interaction
When a user interacts with a search result, you should handle the action appropriately. Typically, this involves opening the relevant view or content within your app.
swift func handleSearchResult(identifier: String) { // Retrieve the relevant content based on the identifier // Display the content to the user }
3. Advanced Usage and Best Practices
3.1. Updating and Deleting Searchable Items
Your app’s content may change over time, so it’s essential to update or delete searchable items as needed. To update an item, simply create a new searchable item with the same unique identifier, and Core Spotlight will replace the existing item with the updated one.
To delete a searchable item, use the deleteSearchableItems method of CSSearchableIndex.
3.2. Prioritize Searchable Items
You can prioritize certain searchable items to appear at the top of search results. Use the expirationDate property to specify when an item should expire and no longer appear in search results.
3.3. Implement Thorough Error Handling
When working with Core Spotlight, it’s crucial to implement error handling to address any issues that may arise during indexing or search. Proper error handling ensures that your app remains robust and reliable.
4. Integrating Core Spotlight with Your App’s Data
To make Core Spotlight truly effective, you should integrate it with your app’s data structure. Here are some additional tips:
4.1. Use a Data Model
Consider using a data model or data structure that mirrors the content in your app. This makes it easier to generate searchable items programmatically and keep them in sync with your app’s data.
4.2. Automate Indexing
Automate the process of creating and updating searchable items whenever your app’s content changes. This ensures that the search index remains up-to-date without manual intervention.
4.3. Optimize Content Descriptions
Craft informative and concise content descriptions for your searchable items. This helps users quickly understand what each search result represents.
5. Testing Core Spotlight Integration
Testing is a crucial part of any app development process. To ensure that your Core Spotlight integration works as expected, follow these testing steps:
- Manual Testing: Manually search for content within your app using the iOS device’s built-in search functionality. Verify that search results link to the correct content within your app.
- Automated Testing: Consider implementing automated UI tests that simulate user interactions with the search feature. Automated tests help catch regressions and ensure that search functionality remains reliable across app updates.
Conclusion
Core Spotlight is a powerful tool for enhancing the search capabilities of your iOS app. By indexing your app’s content with Core Spotlight, you improve user experience and content discoverability. Remember to follow best practices, automate indexing, and test thoroughly to ensure a seamless search experience for your app’s users. With Core Spotlight, your app can stand out in the competitive world of mobile applications.
Incorporating search functionality using Core Spotlight can be a game-changer for your iOS app. So, start harnessing the power of Core Spotlight today and make your app’s content easily accessible to users.
Table of Contents
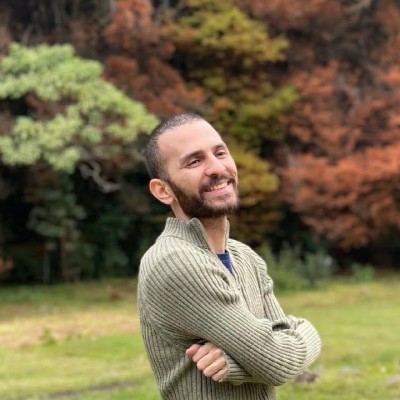
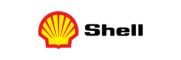