Exploring Java 9 Features: Modules and More
The Java programming language has been a cornerstone of software development for decades, consistently evolving to meet the demands of modern application development. With the release of Java 9, a host of new features were introduced, revolutionizing the way developers approach their projects. One of the most notable additions is the introduction of Modules, a groundbreaking concept that brings modularity and encapsulation to Java applications. In this article, we will dive deep into the world of Java 9 and explore its features, with a special focus on Modules, along with other significant enhancements that it brings.
Table of Contents
1. Understanding Java 9’s Significance
Java 9, released in September 2017, marked a significant shift in the Java ecosystem. While previous versions introduced incremental improvements, Java 9 brought about substantial changes that addressed some long-standing challenges in Java development. One of the major goals of Java 9 was to enhance modularity, which led to the introduction of the Java Platform Module System (JPMS).
2. Introducing Modules: A Paradigm Shift in Java Development
2.1. What are Modules?
Modules are a fundamental addition to Java 9 that enables developers to create more modular and maintainable codebases. A module is a collection of related code, resources, and data, bundled together in a single unit. This unit of encapsulation allows developers to control the accessibility of code within and between modules, reducing the risk of conflicts and making the codebase more manageable.
2.2. Advantages of Modules
2.2.1. Encapsulation and Abstraction
Modules promote encapsulation, which means that the internal implementation details of a module are hidden from other modules. This enhances abstraction and reduces tight coupling between components, making it easier to reason about the codebase and maintain its integrity.
2.2.2. Dependency Management
With modules, dependency management becomes more streamlined. You can specify the dependencies of a module explicitly, making it clear which modules rely on others. This approach prevents classpath issues and version conflicts that were common in previous Java versions.
2.2.3. Improved Readability and Maintainability
Large codebases can become convoluted and challenging to maintain. Modules encourage breaking down monolithic applications into smaller, focused units. This separation enhances code readability, facilitates debugging, and simplifies maintenance.
2.3. Creating and Using Modules
Let’s take a look at how to create and use modules in Java 9.
2.3.1. Defining a Module
To define a module, you use the module keyword followed by the module’s name. Modules are stored in directories named after the module and contain a module-info.java file.
java module com.example.mymodule { // Module declarations and exports }
2.3.2. Exporting Packages
Modules encapsulate packages, and to make a package accessible to other modules, you need to explicitly export it. This helps in controlling which parts of your module are accessible to the outside world.
java module com.example.myprovider { exports com.example.provider.api; }
2.3.3. Requiring Dependencies
A module can require other modules to use their exported packages. This forms the basis of dependency management in Java 9.
java module com.example.myconsumer { requires com.example.myprovider; }
3. Other Java 9 Features to Explore
While Modules stand out as a flagship feature of Java 9, several other enhancements contribute to making Java development more efficient and enjoyable.
3.1. JShell: Interactive Java REPL
JShell is an interactive Read-Evaluate-Print Loop (REPL) tool that allows developers to experiment with Java code snippets. It’s an excellent learning tool and also facilitates quick prototyping and testing.
java jshell> int x = 10; x ==> 10 jshell> int y = 20; y ==> 20 jshell> int sum = x + y; sum ==> 30
3.2. Private Methods in Interfaces
Java 9 introduced the ability to include private methods in interfaces. These methods can be used for common code within the interface without exposing them to the implementing classes.
java public interface MyInterface { default void publicMethod() { // Public method logic } private void privateMethod() { // Private method logic } }
3.3. Improved Streams API
Java 9 enhanced the Streams API with new methods that provide more flexibility and control over stream operations.
java List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5); List<Integer> squaredNumbers = numbers.stream() .map(n -> n * n) .collect(Collectors.toList());
4. Adopting Java 9: Considerations and Compatibility
Before fully adopting Java 9, there are some important factors to consider.
4.1. Module Migration
Migrating existing codebases to modules requires careful planning. Identifying the boundaries between modules and managing dependencies can be a complex task. However, the benefits of improved maintainability and dependency management can outweigh the initial challenges.
4.2. Library and Tool Compatibility
Before upgrading to Java 9, ensure that the libraries, frameworks, and tools you rely on are compatible with the new version. Some third-party components might need updates to function correctly with the Java 9 module system.
Conclusion
Java 9 introduced a plethora of new features, with Modules being the highlight of the release. The introduction of modularity has transformed the way Java applications are built and maintained, making them more modular, scalable, and easier to manage. Additionally, the smaller enhancements like JShell, private methods in interfaces, and improvements to the Streams API contribute to enhancing the developer experience.
As with any major update, transitioning to Java 9 requires careful consideration of the benefits and challenges it brings. However, the advantages of modularity and the other features make it a compelling choice for modern Java application development. By understanding and harnessing the power of Java 9 features, developers can take their projects to new heights of efficiency and maintainability.
Table of Contents
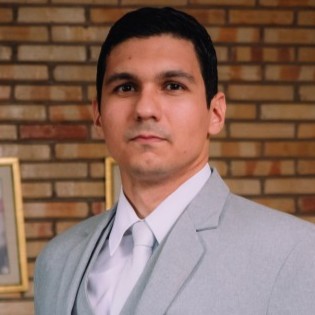
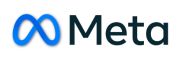