Java Concurrency: Managing Parallel Execution
In the dynamic landscape of modern software development, the need for efficient parallel execution has become paramount. With the rise of multi-core processors and distributed systems, mastering concurrency is no longer an option but a necessity. In the Java ecosystem, concurrency is a fundamental aspect that developers must grapple with to build robust and scalable applications. In this article, we delve into the intricacies of Java concurrency and explore strategies for managing parallel execution.
1. Understanding Concurrency in Java
Concurrency in Java refers to the ability of a program to execute multiple tasks simultaneously. This is achieved through the use of threads, which are lightweight processes that run within a single Java process. Threads enable developers to perform tasks concurrently, thereby leveraging the full potential of modern hardware.
2. Challenges of Concurrency
While concurrency offers significant performance benefits, it also introduces complexity and challenges. One of the primary challenges is the management of shared resources. In a concurrent environment, multiple threads may access shared data concurrently, leading to race conditions, deadlocks, and other synchronization issues. Effectively managing these challenges is crucial to ensure the correctness and reliability of concurrent Java applications.
3. Managing Parallel Execution
To effectively manage parallel execution in Java, developers employ various techniques and constructs provided by the Java concurrency framework. Let’s explore some of these strategies:
- Synchronization: Synchronization is a fundamental mechanism for coordinating access to shared resources in a multi-threaded environment. In Java, synchronization is achieved using the `synchronized` keyword or by explicitly locking objects using `Lock` interfaces. By synchronizing critical sections of code, developers can prevent data corruption and ensure thread safety.
Example: Java Synchronization Tutorial – https://www.baeldung.com/java-synchronization
- Thread Pools: Thread pools are a mechanism for managing and reusing threads to execute multiple tasks concurrently. In Java, thread pools are implemented using the `Executor` framework, which provides a high-level abstraction for managing threads. By using thread pools, developers can control resource consumption, limit the number of concurrent tasks, and improve the scalability of applications.
Example: Java ExecutorService – https://www.baeldung.com/java-executors
- Atomic Variables: Atomic variables are special types provided by the `java.util.concurrent.atomic` package that support atomic operations such as compare-and-set and get-and-increment. These variables ensure that operations on them are executed atomically, without the need for explicit synchronization. Atomic variables are particularly useful for implementing lock-free algorithms and improving performance in highly concurrent scenarios.
Example: Java Atomic Variables – https://www.baeldung.com/java-atomic-variables
- Concurrent Collections: Java provides a set of thread-safe collection classes in the `java.util.concurrent` package, such as `ConcurrentHashMap` and `ConcurrentLinkedQueue`, which are designed for concurrent access by multiple threads. These collections offer higher concurrency and performance compared to their synchronized counterparts, making them ideal for shared data structures in concurrent applications.
Example: Java Concurrent Collections – https://www.baeldung.com/java-concurrent-collections
Conclusion
Concurrency is a fundamental aspect of modern Java programming, enabling developers to harness the power of parallel execution. By understanding the challenges of concurrency and employing effective management strategies such as synchronization, thread pools, atomic variables, and concurrent collections, developers can build robust and scalable Java applications that meet the demands of today’s computing environments.
Mastering concurrency is essential for Java developers seeking to optimize the performance and scalability of their applications in a multi-core, multi-threaded world.
For further reading and exploration:
– Java Concurrency in Practice – https://jcip.net/
– Oracle Java Concurrency Tutorial – https://docs.oracle.com/javase/tutorial/essential/concurrency/
– Effective Java Concurrency – https://www.amazon.com/Effective-Java-Concurrency-Brian-Goetz/dp/0321127425
Table of Contents
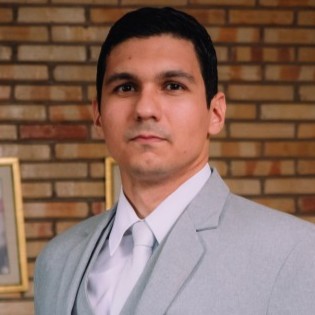
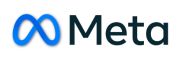