Java Data Structures: Arrays, Lists, and Maps
Java offers a variety of data structures that are essential for managing and organizing data in your programs. Among these, arrays, lists, and maps are fundamental structures that provide different ways to store and access data. This article explores how to use these data structures effectively, with practical examples and best practices.
Arrays: Fixed-Size Data Structure
Arrays in Java are a basic data structure that allows you to store a fixed-size sequence of elements of the same type. They are useful when you know the size of your data in advance and need to store elements in a contiguous block of memory.
Example: Creating and Accessing an Array
Here’s how you can create and access elements in an array:
```java public class ArrayExample { public static void main(String[] args) { // Declare and initialize an array of integers int[] numbers = {1, 2, 3, 4, 5}; // Accessing elements in the array for (int i = 0; i < numbers.length; i++) { System.out.println("Element at index " + i + ": " + numbers[i]); } } } ```
Lists: Dynamic Data Structure
Lists in Java, particularly `ArrayList` and `LinkedList`, are dynamic data structures that allow you to store elements without knowing the size in advance. Lists can grow and shrink as needed, making them more flexible than arrays.
Example: Using ArrayList
Here’s how you can create and manipulate an `ArrayList`:
```java import java.util.ArrayList; public class ListExample { public static void main(String[] args) { // Declare and initialize an ArrayList of Strings ArrayList<String> fruits = new ArrayList<>(); // Adding elements to the ArrayList fruits.add("Apple"); fruits.add("Banana"); fruits.add("Orange"); // Iterating over the ArrayList for (String fruit : fruits) { System.out.println(fruit); } } } ```
Maps: Key-Value Data Structure
Maps in Java, such as `HashMap`, `TreeMap`, and `LinkedHashMap`, allow you to store key-value pairs, where each key is unique. Maps are ideal for situations where you need to quickly retrieve, update, or delete elements based on a unique key.
Example: Using HashMap
Here’s an example of how to use a `HashMap` to store and retrieve values:
```java import java.util.HashMap; public class MapExample { public static void main(String[] args) { // Declare and initialize a HashMap HashMap<String, Integer> scores = new HashMap<>(); // Adding key-value pairs to the HashMap scores.put("Alice", 85); scores.put("Bob", 90); scores.put("Charlie", 95); // Retrieving and displaying values based on keys System.out.println("Alice's score: " + scores.get("Alice")); System.out.println("Bob's score: " + scores.get("Bob")); System.out.println("Charlie's score: " + scores.get("Charlie")); } } ```
When to Use Each Data Structure
– Arrays: Best for situations where the size of the data is fixed and you require fast access by index.
– Lists: Ideal when you need a flexible data structure that can grow and shrink dynamically, with easy insertion and deletion of elements.
– Maps: Perfect for storing and accessing data via unique keys, with quick look-up times.
Conclusion
Understanding and effectively using arrays, lists, and maps in Java is crucial for writing efficient and maintainable code. Each data structure serves different purposes, and choosing the right one can significantly impact the performance and scalability of your applications.
Further Reading:
Table of Contents
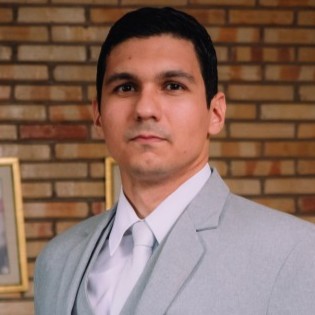
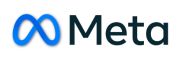