Java Database Access (JDBC): Querying and Manipulating Data
Java Database Connectivity (JDBC) is a crucial API for Java applications that need to interact with databases. It provides a standardized way to connect to relational databases, execute queries, and manipulate data. This blog explores the essentials of JDBC and offers practical examples to help you efficiently query and manage your data using Java.
Understanding JDBC
JDBC is an API that allows Java applications to connect to relational databases, execute SQL statements, and retrieve results. It abstracts the underlying database details, making it easier to write database-independent code.
Using JDBC for Database Operations
JDBC provides several core components, including the `DriverManager`, `Connection`, `Statement`, and `ResultSet` classes, which facilitate database interactions. Below are key aspects and code examples demonstrating how to use JDBC for querying and manipulating data.
1. Establishing a Database Connection
Before performing any database operations, you need to establish a connection to the database using JDBC.
Example: Connecting to a MySQL Database
```java import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; public class DatabaseConnector { public static void main(String[] args) { String url = "jdbc:mysql://localhost:3306/mydatabase"; String user = "root"; String password = "password"; try (Connection connection = DriverManager.getConnection(url, user, password)) { System.out.println("Connection established successfully!"); } catch (SQLException e) { e.printStackTrace(); } } } ```
2. Executing SQL Queries
Once connected, you can execute SQL queries to retrieve or manipulate data. JDBC provides `Statement`, `PreparedStatement`, and `CallableStatement` for executing different types of SQL queries.
Example: Querying Data with `Statement`
```java import java.sql.Connection; import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.SQLException; import java.sql.Statement; public class QueryExample { public static void main(String[] args) { String url = "jdbc:mysql://localhost:3306/mydatabase"; String user = "root"; String password = "password"; try (Connection connection = DriverManager.getConnection(url, user, password); Statement statement = connection.createStatement()) { String query = "SELECT FROM users"; ResultSet resultSet = statement.executeQuery(query); while (resultSet.next()) { int id = resultSet.getInt("id"); String name = resultSet.getString("name"); System.out.println("ID: " + id + ", Name: " + name); } } catch (SQLException e) { e.printStackTrace(); } } } ```
3. Manipulating Data
JDBC allows you to insert, update, and delete data using SQL statements. The `PreparedStatement` class is commonly used for these operations to avoid SQL injection and improve performance.
Example: Inserting Data with `PreparedStatement`
```java import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.SQLException; public class InsertData { public static void main(String[] args) { String url = "jdbc:mysql://localhost:3306/mydatabase"; String user = "root"; String password = "password"; String insertSQL = "INSERT INTO users (name, email) VALUES (?, ?)"; try (Connection connection = DriverManager.getConnection(url, user, password); PreparedStatement preparedStatement = connection.prepareStatement(insertSQL)) { preparedStatement.setString(1, "John Doe"); preparedStatement.setString(2, "john.doe@example.com"); int rowsAffected = preparedStatement.executeUpdate(); System.out.println("Rows inserted: " + rowsAffected); } catch (SQLException e) { e.printStackTrace(); } } } ```
4. Handling Transactions
JDBC supports transaction management, allowing you to commit or rollback changes based on the outcome of multiple operations.
Example: Managing Transactions
```java import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.SQLException; public class TransactionExample { public static void main(String[] args) { String url = "jdbc:mysql://localhost:3306/mydatabase"; String user = "root"; String password = "password"; String updateSQL = "UPDATE users SET email = ? WHERE name = ?"; try (Connection connection = DriverManager.getConnection(url, user, password)) { connection.setAutoCommit(false); try (PreparedStatement preparedStatement = connection.prepareStatement(updateSQL)) { preparedStatement.setString(1, "new.email@example.com"); preparedStatement.setString(2, "John Doe"); int rowsAffected = preparedStatement.executeUpdate(); System.out.println("Rows updated: " + rowsAffected); // Commit the transaction connection.commit(); } catch (SQLException e) { // Rollback in case of error connection.rollback(); e.printStackTrace(); } } catch (SQLException e) { e.printStackTrace(); } } } ```
Conclusion
JDBC is a powerful tool for Java developers needing to interact with relational databases. From establishing connections and executing queries to manipulating data and managing transactions, JDBC offers a robust framework for database operations. Mastering these capabilities will enhance your ability to develop data-driven Java applications efficiently.
Further Reading:
Table of Contents
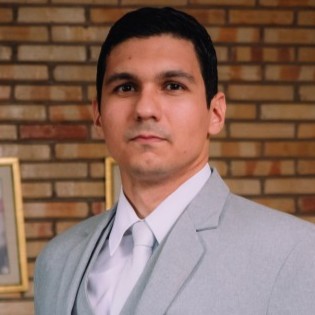
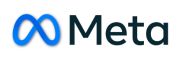