Java Design Patterns: Building Scalable Applications
In the fast-paced world of software development, building scalable applications is crucial for success. Scalability ensures that your application can handle increasing loads of users and data without compromising performance. One powerful tool in achieving scalability is the use of design patterns. In this article, we’ll explore some essential Java design patterns that can help you build scalable applications.
Introduction to Design Patterns
Design patterns are proven solutions to recurring design problems encountered in software development. They provide a blueprint for constructing robust and maintainable code by promoting best practices and encapsulating design principles.
Singleton Pattern
The Singleton pattern ensures that a class has only one instance and provides a global point of access to that instance. This pattern is particularly useful when you need to control access to shared resources or manage centralized configuration settings.
Example
Java’s Runtime class is a classic example of the Singleton pattern. It represents the runtime environment of the current Java application and ensures that only one instance exists throughout the application lifecycle.
Learn more about the Singleton pattern here.
Factory Pattern
The Factory pattern is used to create objects without specifying the exact class of the object that will be created. It promotes loose coupling by abstracting the process of object creation and allowing subclasses to alter the type of objects that will be instantiated.
Example
Consider a PaymentGatewayFactory that creates different payment gateway objects based on configuration or runtime conditions. This allows for easy integration of new payment gateways without modifying existing code.
Explore advanced Factory pattern implementations here.
Observer Pattern
The Observer pattern defines a one-to-many dependency between objects, where one object (the subject) notifies its observers of any state changes, automatically triggering updates in dependent objects. This pattern facilitates the creation of loosely coupled systems by decoupling the sender and receiver of notifications.
Example
In a stock market application, the StockExchange serves as the subject, while various traders act as observers. When the price of a stock changes, the StockExchange notifies all registered traders, enabling them to react accordingly.
Deep dive into the Observer pattern here.
Conclusion
Design patterns play a crucial role in building scalable and maintainable Java applications. By leveraging patterns like Singleton, Factory, and Observer, developers can design flexible and resilient systems capable of adapting to changing requirements and scaling gracefully with growing user demands.
Incorporating these design patterns into your Java projects not only improves code quality but also fosters a deeper understanding of software architecture principles. As you continue your journey in software development, mastering design patterns will undoubtedly elevate your skills and make you a more effective and sought-after developer.
Remember, while design patterns offer valuable guidelines and solutions, it’s essential to apply them judiciously and adapt them to suit the specific needs and constraints of your projects.
Happy coding!
Table of Contents
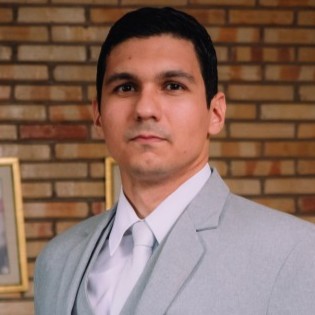
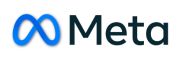