Understanding File Operations in Java: Working with Files using java.io and java.nio
Java, renowned for its robust capabilities in handling Input and Output (I/O) operations and interacting with file systems, is an excellent programming language for a wide array of applications. This makes it an essential skill set to look for when you aim to hire Java developers. In this article, we delve into the various methods Java developers use to read from and write to files, leveraging both traditional and newer APIs like `java.io` and `java.nio`. Understanding these functionalities can give you a clearer perspective on what to expect when you hire Java developers for your projects.
1. Traditional I/O with java.io
Java’s `java.io` package offers the `File` class, which gives us interfaces to interact with file systems. Additionally, it provides various stream classes for both reading and writing.
1.1. Reading a File
Here’s a simple example of reading a file using `FileReader` and `BufferedReader`:
```java import java.io.*; public class ReadFile { public static void main(String[] args) { try { File file = new File("example.txt"); FileReader fileReader = new FileReader(file); BufferedReader bufferedReader = new BufferedReader(fileReader); String line; while ((line = bufferedReader.readLine()) != null) { System.out.println(line); } bufferedReader.close(); } catch (IOException e) { e.printStackTrace(); } } } ```
In this snippet, we’ve created an instance of `File` to represent our `example.txt` file. We then use a `FileReader` and `BufferedReader` to read the file line by line.
1.2. Writing to a File
Writing to a file can be achieved using `FileWriter` and `BufferedWriter`. Let’s see how to do that:
```java import java.io.*; public class WriteFile { public static void main(String[] args) { try { File file = new File("example.txt"); FileWriter fileWriter = new FileWriter(file); BufferedWriter bufferedWriter = new BufferedWriter(fileWriter); bufferedWriter.write("Hello, World!"); bufferedWriter.close(); } catch (IOException e) { e.printStackTrace(); } } } ```
In this code snippet, we’re writing the string “Hello, World!” to the `example.txt` file.
2. New I/O with java.nio
The `java.nio` package, also known as NIO (New Input/Output), provides more powerful features for I/O operations, and is generally more efficient than the traditional `java.io` package.
2.1. Reading a File
The `Files` and `Paths` classes offer methods for performing file operations. Here’s an example of reading a file using `java.nio`:
```java import java.nio.file.*; import java.io.*; public class NIOReadFile { public static void main(String[] args) { try { Path path = Paths.get("example.txt"); byte[] bytes = Files.readAllBytes(path); String content = new String(bytes); System.out.println(content); } catch (IOException e) { e.printStackTrace(); } } } ```
In this example, we’re using the `Paths.get()` method to get a `Path` instance representing our file. Then we’re reading all bytes from the file with `Files.readAllBytes()`, converting them to a `String`, and printing the content.
2.2. Writing to a File
Writing to a file is also simple using `Files` and `Paths`. Let’s look at an example:
```java import java.nio.file.*; import java.io.*; public class NIOWriteFile { public static void main(String[] args) { try { Path path = Paths.get("example.txt"); byte[] bytes = "Hello, World!".getBytes(); Files.write(path, bytes); } catch (IOException e) { e.printStackTrace(); } } } ```
In this code snippet, we’re writing the byte representation of the string “Hello, World!” to the `example.txt` file.
3. Stream-Based I/O with java.nio
Java 8 introduced a new way of performing I/O operations using streams, particularly useful when handling large files.
3.1. Reading a File
Here’s an example of reading a file with streams:
```java import java.nio.file.*; import java.io.*; public class StreamReadFile { public static void main(String[] args) { try (Stream<String> lines = Files.lines(Paths.get("example.txt"))) { lines.forEach(System.out::println); } catch (IOException e) { e.printStackTrace(); } } } ```
In this example, we’re reading the lines of a file as a stream and then printing each line to the console.
3.2. Writing to a File
Writing to a file using streams is a bit trickier, as `Files` does not provide a direct method to do so. We have to use a `BufferedWriter` from a `FileWriter`:
```java import java.nio.file.*; import java.io.*; public class StreamWriteFile { public static void main(String[] args) { try (BufferedWriter writer = Files.newBufferedWriter(Paths.get("example.txt"))) { writer.write("Hello, World!"); } catch (IOException e) { e.printStackTrace(); } } } ```
Conclusion
In this post, we explored the various ways to handle file I/O in Java, a skill that is crucial when looking to hire Java developers. By using both the traditional `java.io` and the more modern `java.nio` libraries, we demonstrated the diverse ways Java developers can read and write files, including using streams. Java offers a wide range of options for interacting with file systems, showcasing the versatile capabilities that Java developers possess. For those seeking to hire Java developers, it is important to understand these different tools at your disposal, enabling you to identify skilled professionals who can choose the most appropriate method based on your project’s specific needs.
Table of Contents
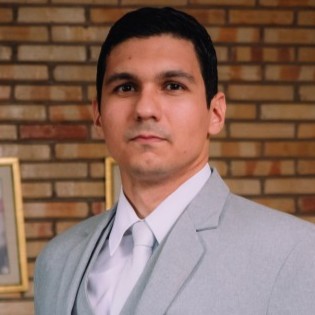
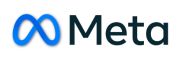