Java Functional Interfaces: Leveraging Lambda Expressions
Functional programming has become an integral part of modern Java development. With the introduction of lambda expressions in Java 8, developers gained the ability to write more concise and readable code. This article explores the concept of functional interfaces in Java, demonstrates how to leverage lambda expressions, and provides practical examples to showcase their power.
Understanding Functional Interfaces
A functional interface in Java is an interface that contains exactly one abstract method. This single abstract method defines the functional contract, which can be implemented using a lambda expression, method reference, or an anonymous class. Functional interfaces are the backbone of functional programming in Java.
Example: Defining a Custom Functional Interface
Here’s how you can define a custom functional interface:
```java @FunctionalInterface public interface MathOperation { int operate(int a, int b); } ```
This interface has a single abstract method `operate`, making it a functional interface. You can now implement this interface using a lambda expression.
Leveraging Lambda Expressions
Lambda expressions are a way to provide a concise implementation of a functional interface. They are particularly useful for writing inline code that doesn’t require a full class definition.
Example: Using a Lambda Expression with MathOperation
```java public class Main { public static void main(String[] args) { MathOperation addition = (a, b) -> a + b; MathOperation multiplication = (a, b) -> a * b; System.out.println("Addition: " + addition.operate(5, 3)); System.out.println("Multiplication: " + multiplication.operate(5, 3)); } } ```
In this example, the `MathOperation` interface is implemented using lambda expressions, providing a clear and concise way to define operations.
Built-in Functional Interfaces in Java
Java provides several built-in functional interfaces in the `java.util.function` package, such as `Predicate`, `Function`, `Consumer`, and `Supplier`. These interfaces simplify common coding patterns and promote a functional style of programming.
Example: Using the Predicate Interface
The `Predicate` interface represents a single argument function that returns a boolean value. It’s often used for filtering data.
```java import java.util.function.Predicate; public class Main { public static void main(String[] args) { Predicate<String> isLongerThanFive = s -> s.length() > 5; System.out.println(isLongerThanFive.test("Hello")); // false System.out.println(isLongerThanFive.test("Hello World")); // true } } ```
This example demonstrates how the `Predicate` interface can be used to check if a string is longer than five characters.
Method References and Lambda Expressions
Method references are another concise way to implement functional interfaces. They allow you to refer to methods by their names directly, without having to invoke them.
Example: Using a Method Reference
```java import java.util.function.Function; public class Main { public static void main(String[] args) { Function<String, Integer> stringLength = String::length; System.out.println(stringLength.apply("Hello World")); // 11 } } ```
Here, the `String::length` method reference replaces a lambda expression, making the code cleaner and more readable.
Higher-Order Functions and Composition
Functional interfaces in Java can be composed to create higher-order functions—functions that operate on or return other functions. This allows for more flexible and reusable code.
Example: Composing Functions
```java import java.util.function.Function; public class Main { public static void main(String[] args) { Function<Integer, Integer> multiplyByTwo = x -> x * 2; Function<Integer, Integer> square = x -> x * x; Function<Integer, Integer> multiplyThenSquare = multiplyByTwo.andThen(square); System.out.println(multiplyThenSquare.apply(3)); // 36 } } ```
In this example, the `andThen` method is used to compose two functions, first multiplying by two and then squaring the result.
Conclusion
Java’s functional interfaces and lambda expressions offer a powerful way to write cleaner, more maintainable code. By understanding and leveraging these concepts, you can create more flexible and efficient applications. Whether you are defining custom functional interfaces or using Java’s built-in ones, lambda expressions and method references provide the tools you need to embrace functional programming in Java.
Further Reading:
Table of Contents
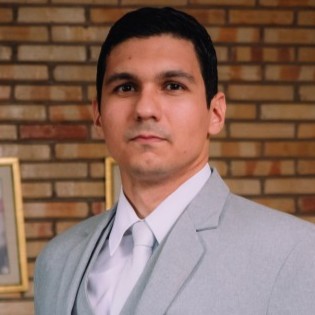
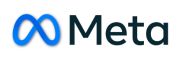