JavaFX Styling and CSS Customization
JavaFX is a powerful platform for building rich desktop applications. One of its standout features is the ability to style applications using CSS, allowing developers to create visually appealing and modern user interfaces. This article delves into the techniques for styling JavaFX applications with CSS and provides practical examples for customizing the look and feel of your JavaFX projects.
Understanding JavaFX and CSS
JavaFX allows developers to apply CSS styles to their UI components, much like how web developers style HTML elements. This makes it possible to create consistent, theme-based designs across your application.
Setting Up CSS in JavaFX
Before you can begin styling, you need to link a CSS file to your JavaFX application. This is done by adding the CSS file to your `Scene` or individual UI components.
Example: Linking a CSS File
Here’s how you can link a CSS file to a JavaFX `Scene`.
```java import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.layout.StackPane; import javafx.stage.Stage; public class Main extends Application { @Override public void start(Stage primaryStage) { StackPane root = new StackPane(); Scene scene = new Scene(root, 400, 300); // Link the CSS file scene.getStylesheets().add("styles.css"); primaryStage.setScene(scene); primaryStage.show(); } public static void main(String[] args) { launch(args); } } ```
Basic Styling with CSS
Once your CSS file is linked, you can start applying styles to your JavaFX components. You can style everything from colors and fonts to padding and margins.
Example: Styling a Button
Let’s style a simple `Button` to give it a custom look.
```css /* styles.css */ .button { -fx-background-color: #007bff; -fx-text-fill: white; -fx-font-size: 16px; -fx-padding: 10px 20px; -fx-background-radius: 5px; } .button:hover { -fx-background-color: #0056b3; } ```
This CSS will style all `Button` elements, giving them a blue background, white text, and rounded corners.
Advanced CSS Customization
JavaFX supports a range of advanced CSS features that allow for more intricate designs. These include using custom fonts, background images, and even animations.
Example: Adding a Custom Font
To use a custom font in your JavaFX application, you can define it in your CSS and apply it to specific elements.
```css /* styles.css */ @font-face { font-family: 'CustomFont'; src: url('path/to/font.ttf'); } .label { -fx-font-family: 'CustomFont'; -fx-font-size: 18px; } ```
Example: Background Images and Gradients
You can also apply background images or gradients to your UI components.
```css /* styles.css */ .stack-pane { -fx-background-image: url('background.jpg'); -fx-background-size: cover; -fx-padding: 20px; } .button { -fx-background-color: linear-gradient(to right, #ff7e5f, #feb47b); } ```
Animating CSS Properties
CSS in JavaFX supports basic animations, allowing you to create smooth transitions and effects.
Example: Button Hover Animation
Here’s how you can animate a button’s background color when it’s hovered over.
```css /* styles.css */ .button { -fx-background-color: #007bff; -fx-text-fill: white; -fx-font-size: 16px; -fx-padding: 10px 20px; -fx-background-radius: 5px; -fx-transition: background-color 0.3s ease; } .button:hover { -fx-background-color: #0056b3; } ```
This will create a smooth transition effect when the button’s background color changes on hover.
Customizing Specific Components
JavaFX’s flexibility allows you to target specific components or groups of components for customization. You can use ID selectors or class selectors to apply styles to individual elements.
Example: Styling an ID-Selected Component
You can target an individual component by assigning it an ID and using the `#` selector in your CSS.
```java Button button = new Button("Click Me"); button.setId("specialButton"); ``` ```css /* styles.css */ #specialButton { -fx-background-color: #28a745; -fx-text-fill: white; } ```
Conclusion
CSS customization in JavaFX offers developers the ability to create visually appealing applications with ease. Whether you’re aiming for a simple, clean look or a complex, animated interface, JavaFX’s CSS capabilities provide the tools you need. By mastering these techniques, you can take your JavaFX applications to the next level, creating user experiences that are both functional and beautiful.
Further Reading:
Table of Contents
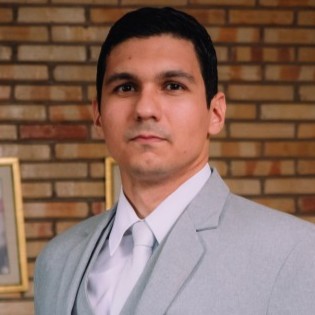
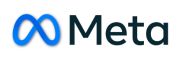