Introduction to JavaServer Pages (JSP)
In the realm of web development, creating dynamic and interactive web pages is a fundamental goal. The evolution of web technologies has provided developers with various tools and frameworks to achieve this objective efficiently. One such technology that plays a crucial role in developing dynamic web pages is JavaServer Pages, commonly known as JSP. In this blog post, we will embark on a journey to understand the basics of JavaServer Pages, its benefits, and how it works to create powerful web applications.
Table of Contents
1. What are JavaServer Pages (JSP)?
1.1 Understanding Server-Side Technologies
Before delving into JavaServer Pages, it’s essential to comprehend the distinction between client-side and server-side technologies. Client-side technologies primarily involve HTML, CSS, and JavaScript, which are executed on the user’s browser. In contrast, server-side technologies operate on the web server and generate content dynamically before sending it to the client’s browser. This approach enables developers to create dynamic and data-driven web applications.
1.2 The Role of JSP in Web Development
JavaServer Pages (JSP) is a server-side technology that allows developers to embed Java code within HTML templates. This combination of Java and HTML makes it possible to create dynamic web pages that can interact with databases, process user input, and generate personalized content. JSP pages are compiled into servlets, which are Java classes responsible for generating dynamic content. This compilation process occurs automatically behind the scenes, ensuring that the web application remains efficient and responsive.
2. The Anatomy of a JSP Page
A JSP page resembles a standard HTML page with embedded Java code snippets. This amalgamation of code and markup is what empowers JSP to create dynamic content seamlessly.
2.1 Embedding Java Code in HTML
jsp <!DOCTYPE html> <html> <head> <title>JSP Example</title> </head> <body> <h1>Welcome to our website, <%= request.getParameter("username") %></h1> <p>Today is <%= new java.util.Date() %></p> </body> </html>
In this code snippet, notice the use of <%= … %> tags. These tags allow us to embed Java expressions directly into the HTML content. The request.getParameter(“username”) retrieves a parameter from the user’s request, while new java.util.Date() generates the current date and time.
2.2 The significance of .jsp Extension
JSP files are typically saved with the .jsp extension, which informs the web server to treat them as JavaServer Pages. When a user requests a JSP page, the server processes the embedded Java code, generates HTML content, and sends it back to the client’s browser.
2.3 Lifecycle of a JSP Page
Understanding the lifecycle of a JSP page is crucial for effective development. The JSP lifecycle includes stages such as translation, compilation, initialization, and service. During the translation phase, the JSP page is converted into a servlet. The compilation step involves converting the translated JSP page into bytecode that the Java Virtual Machine (JVM) can execute. Initialization includes setting up resources, and the service phase generates the dynamic content for client requests.
3. Benefits of Using JSP
JavaServer Pages offer several advantages that make them a popular choice for developing dynamic web applications.
3.1 Seamless Integration of Java Code and HTML
JSP allows developers to integrate Java code directly into HTML, promoting a clean separation of concerns while enabling dynamic content generation. This integration eliminates the need for extensive JavaScript coding to manipulate the DOM and keeps the codebase organized.
3.2 Reusable Components with Custom Tag Libraries
JSP supports custom tag libraries, which are pre-built components that encapsulate complex functionality. This promotes code reusability and helps maintain a modular architecture for the web application. The JavaServer Pages Standard Tag Library (JSTL) is a widely used tag library that simplifies tasks like iteration, conditionals, and formatting.
3.3 Support for Java EE Ecosystem
JSP is part of the Java EE (Enterprise Edition) ecosystem, which provides a suite of libraries, APIs, and tools for developing large-scale, enterprise-level applications. This ecosystem includes features like servlets, database connectivity, security mechanisms, and more, seamlessly integrating with JSP to create robust web applications.
4. Getting Started with JSP
To start developing with JSP, you’ll need a suitable development environment and an understanding of how to create and deploy JSP applications.
4.1 Setting Up a JSP Development Environment
Before diving into JSP development, ensure you have Java Development Kit (JDK) and a web server installed. Apache Tomcat is a popular choice as a Java-based web server and servlet container that supports JSP.
4.2 First JSP Example: Hello World
Let’s create a simple “Hello World” JSP example to understand the basics.
Step 1: Create a file named hello.jsp.
jsp <%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html> <html> <head> <title>Hello JSP</title> </head> <body> <h1>Hello, JSP World!</h1> </body> </html>
Step 2: Deploy the JSP to your web server.
Copy the hello.jsp file to the appropriate directory in your web server’s deployment folder.
Step 3: Access the JSP page in your browser.
Open a web browser and navigate to http://localhost:8080/your-web-app-context/hello.jsp. You should see the “Hello, JSP World!” message displayed in your browser.
4.3 Deploying JSP Applications
Deploying JSP applications involves packaging your web resources, JSP files, and other assets into a web application archive (WAR) file. This WAR file can then be deployed to your web server.
5. Dynamic Content and Expressions in JSP
One of the primary strengths of JSP is its ability to generate dynamic content through the use of Java expressions and scriptlet tags.
5.1 Using Scriptlet Tags for Java Code
Scriptlet tags (<% … %>) allow you to embed Java code directly within your JSP page. For example:
jsp <%@ page language="java" %> <!DOCTYPE html> <html> <head> <title>Dynamic Content</title> </head> <body> <% String greeting = "Hello, JSP!"; out.println(greeting); %> </body> </html>
5.2 Outputting Dynamic Data with Expressions
Expressions (<%= … %>) are used to output the result of Java expressions directly into the HTML content. For instance:
jsp <%@ page language="java" %> <!DOCTYPE html> <html> <head> <title>Dynamic Data</title> </head> <body> <p>The current year is <%= java.time.Year.now().getValue() %></p> </body> </html>
5.3 Conditional Statements and Loops in JSP
JSP allows you to incorporate Java’s control structures, such as if statements and loops, directly within your HTML markup. This enables dynamic rendering of content based on varying conditions:
jsp <%@ page language="java" %> <!DOCTYPE html> <html> <head> <title>Conditional Statements</title> </head> <body> <% int num = 5; if (num > 0) { %> <p>The number is positive.</p> <% } else { %> <p>The number is non-positive.</p> <% } %> </body> </html>
6. JSP Directives and Actions
JSP provides directives and actions that offer control over how the JSP container processes and generates the output.
6.1 Page Directives for Configuring JSP Pages
Page directives are used to provide instructions to the JSP container about how to process a specific JSP page. These directives are placed at the top of the JSP page and are enclosed within <%@ … %>. For example:
jsp <%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html> <html> <head> <title>Page Directive</title> </head> <body> <!-- Content --> </body> </html>
6.2 Include and Forward Actions
JSP includes actions like <jsp:include> and <jsp:forward> that facilitate modular development and navigation between pages.
Example of <jsp:include>:
jsp <jsp:include page="header.jsp" />
Example of <jsp:forward>:
jsp <jsp:forward page="error.jsp" />
6.3 Using JSP Standard Tag Library (JSTL)
JSP Standard Tag Library (JSTL) simplifies common tasks in JSP development, such as iteration, conditional statements, and formatting. JSTL tags are prefixed with c:.
Example of JSTL Loop Tag:
jsp <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <!DOCTYPE html> <html> <head> <title>JSTL Loop</title> </head> <body> <c:forEach var="i" begin="1" end="5"> <p>Iteration <c:out value="${i}" /></p> </c:forEach> </body> </html>
7. Maintaining State and Session Management
JSP provides mechanisms for maintaining user-specific data and managing user sessions.
7.1 Utilizing Request and Response Objects
The request and response objects in JSP enable interaction with client requests and server responses. These objects carry information such as user input and server-generated content.
7.2 Managing User Sessions with JSP
JSP enables the creation and management of user sessions, allowing data to persist across multiple requests. Session data can be stored and retrieved using the session object.
7.3 Hidden Form Fields and Cookies
Hidden form fields and cookies are commonly used techniques for maintaining state between client requests. Hidden form fields store data within the HTML form, while cookies store data on the client’s browser.
8. Integrating JSP with Databases
Integrating JSP with databases is a fundamental aspect of creating dynamic web applications that can store and retrieve information from a persistent data source.
8.1 Connecting to Databases using JDBC
Java Database Connectivity (JDBC) is a standard API for connecting Java applications to relational databases. JDBC enables JSP pages to interact with databases to perform operations such as inserting, updating, and querying data.
8.2 Executing SQL Queries within JSP
JSP can execute SQL queries to retrieve data from a database. However, it’s recommended to separate database-related logic from presentation logic for better code maintainability and security.
8.3 Displaying Database Records on Web Pages
To display database records on web pages, you can iterate through the retrieved data and generate HTML content dynamically. This can be achieved using scriptlet tags, expressions, or JSTL tags.
Conclusion
In conclusion, JavaServer Pages (JSP) is a versatile technology that empowers developers to create dynamic, interactive, and data-driven web applications. By seamlessly integrating Java code with HTML templates, JSP offers a powerful solution for building efficient and responsive web pages. From embedding Java expressions to using custom tag libraries, JSP provides a comprehensive toolkit for developing dynamic content and managing user interactions. As you continue your journey in web development, mastering JSP will undoubtedly enhance your ability to create engaging and feature-rich web applications.
Table of Contents
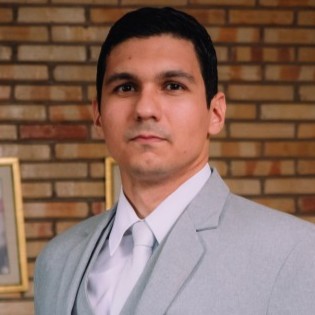
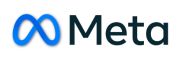