Java Native Interface (JNI): Integrating Native Code
In the ever-evolving landscape of software development, developers often encounter scenarios where they need to leverage existing native code within their Java applications or vice versa. This is where Java Native Interface (JNI) comes into play, offering a bridge between Java code and native applications written in languages like C or C++. In this comprehensive guide, we’ll delve into the intricacies of JNI, explore its benefits, potential use cases, and provide practical examples to illustrate its integration prowess.
Understanding JNI
JNI serves as a framework that enables Java code running in the Java Virtual Machine (JVM) to call and be called by native applications and libraries written in other languages. It acts as a mediator, facilitating communication between the platform-independent Java code and platform-specific native code. JNI achieves this interoperability by defining a set of conventions for mapping Java method calls to native functions and vice versa.
Benefits of Using JNI
- Performance Optimization: JNI allows developers to harness the power of native code for performance-critical tasks, such as mathematical computations or intensive data processing, while retaining the platform independence and portability of Java applications.
- Access to Existing Libraries: By integrating native libraries written in C or C++, developers can leverage a vast ecosystem of pre-existing functionality, including system-level operations, hardware interactions, and specialized algorithms.
- Legacy Code Integration: JNI facilitates the seamless integration of legacy codebases written in native languages with modern Java applications, enabling incremental migration and interoperability between different components.
Practical Examples
Example 1: Integrating a C Library with Java Application
Suppose we have a Java application that requires cryptographic functionality provided by a C library. We can use JNI to create a Java interface for invoking the cryptographic functions defined in the C library.
public class CryptoUtils { // Load native library static { System.loadLibrary("crypto"); } // Native method declaration public native byte[] encrypt(byte[] plaintext); // Native method declaration public native byte[] decrypt(byte[] ciphertext); }
In this example, encrypt and decrypt are native methods declared in the CryptoUtils class. We can then implement these methods in a C file and compile it into a shared library (libcrypto.so on Unix-like systems).
Example 2: Calling Java Code from C Application
Conversely, we might have a native application written in C that needs to invoke functionality provided by a Java class. JNI enables us to embed the JVM within the C application and call Java methods directly.
#include <jni.h> JNIEXPORT void JNICALL Java_com_example_MyClass_nativeMethod(JNIEnv *env, jobject obj) { // Access Java method from C jclass clazz = (*env)->GetObjectClass(env, obj); jmethodID method = (*env)->GetMethodID(env, clazz, "javaMethod", "()V"); (*env)->CallVoidMethod(env, obj, method); }
Here, nativeMethod is a JNI function defined in C that calls a Java method named javaMethod belonging to the MyClass class.
Conclusion
Java Native Interface (JNI) serves as a powerful tool for integrating native code with Java applications, offering developers the flexibility to leverage platform-specific functionality while maintaining the benefits of Java’s platform independence. By understanding JNI’s principles and best practices, developers can unlock new possibilities for extending the capabilities of their Java applications.
For further exploration of JNI and its applications, consider exploring the following resources:
- Official JNI Documentation
- JNI Tutorial – Tutorialspoint
- Using the Java Native Interface – IBM Developer
Whether you’re optimizing performance, accessing system resources, or integrating legacy code, JNI empowers developers to bridge the gap between Java and native environments, opening doors to a world of possibilities in software development.
Table of Contents
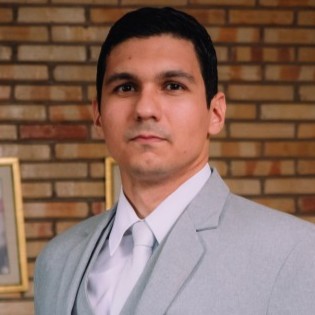
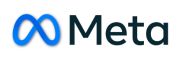