Java Performance Optimization: Improving Speed and Efficiency
In the realm of software development, Java stands tall as one of the most versatile and widely-used programming languages. Its cross-platform compatibility, robustness, and extensive libraries make it a top choice for building a wide range of applications, from enterprise systems to mobile apps. However, with great power comes great responsibility, and ensuring optimal performance is crucial for delivering a seamless user experience. In this article, we’ll explore strategies for optimizing Java performance to enhance speed and efficiency.
1. Understanding the Importance of Performance Optimization
In today’s fast-paced digital landscape, users demand applications that are not only feature-rich but also lightning-fast. Whether it’s a web application loading content in milliseconds or a mobile app responding swiftly to user interactions, performance plays a pivotal role in user satisfaction and retention. By optimizing Java performance, developers can minimize resource consumption, reduce response times, and ultimately deliver a superior user experience.
2. Key Areas for Java Performance Optimization
2.1. Memory Management
Java’s automatic memory management through garbage collection simplifies memory allocation and deallocation, but inefficient memory usage can still lead to performance bottlenecks. To optimize memory usage:
– Use Object Pooling: Reusing objects instead of creating new ones can reduce memory overhead and garbage collection overhead. Libraries like Apache Commons Pool provide efficient object pooling mechanisms.
– Optimize Data Structures: Choose appropriate data structures and collections based on usage patterns to minimize memory footprint. For example, prefer ArrayList over LinkedList for random access operations to reduce memory overhead.
– Tune Garbage Collection: Adjust garbage collection settings such as heap size, garbage collector algorithm, and generation sizes based on application requirements and workload characteristics.
2.2. Multithreading and Concurrency
Java’s support for multithreading enables parallel execution of tasks, but improper synchronization and contention can degrade performance. To optimize concurrency:
– Use Thread Pools: Instead of creating new threads for each task, utilize thread pools to manage a fixed number of reusable threads, reducing thread creation overhead.
– Synchronize Strategically: Minimize synchronized blocks and use finer-grained locking mechanisms like ReadWriteLock to reduce contention and increase throughput.
– Explore Java Concurrency Utilities: Leverage high-level concurrency utilities like Executor Framework, Concurrent Collections, and CompletableFuture for efficient concurrent programming.
2.3. Performance Profiling and Tuning
Identifying performance bottlenecks and fine-tuning critical components is essential for achieving optimal Java performance. To profile and tune performance:
– Use Profiling Tools: Utilize tools like Java Mission Control, VisualVM, and YourKit Profiler to analyze CPU usage, memory allocation, and thread activity to pinpoint performance hotspots.
– Optimize Critical Code Paths: Focus on optimizing critical code paths identified through profiling by employing techniques like algorithmic improvements, caching, and JIT compiler hints.
– Benchmark and Iterate: Continuously benchmark application performance after optimizations to validate improvements and identify further optimization opportunities.
3. Real-World Examples of Java Performance Optimization
- Netflix: Netflix, a pioneer in streaming technology, heavily relies on Java for its backend infrastructure. By optimizing Java performance, Netflix ensures smooth streaming experiences for millions of users worldwide. They employ techniques like thread pool tuning, memory optimization, and asynchronous processing to achieve high throughput and low latency.
Read more about Netflix’s Java optimization strategies – https://netflixtechblog.com/java-at-netflix-episode-1-3f39007d4c49
- Twitter: Twitter, a social media giant handling billions of tweets daily, leverages Java for its microservices architecture. To maintain real-time responsiveness and scalability, Twitter optimizes Java performance through efficient thread management, caching, and request batching.
Explore Twitter’s approach to Java performance optimization – https://blog.twitter.com/engineering/en_us/topics/infrastructure/2019/java-collection-batching.html
- Google: Google relies on Java for a myriad of its services, including Android OS and backend systems. Through meticulous performance tuning and optimization, Google ensures snappy user experiences across its diverse range of products, from search to cloud services.
Learn about Google’s best practices for Java performance optimization – https://cloud.google.com/blog/products/gcp/google-cloud-performance-at-speed-and-scale
Conclusion
Optimizing Java performance is a multifaceted endeavor that involves meticulous attention to memory management, concurrency, profiling, and tuning. By adopting best practices and learning from real-world examples, developers can unleash the full potential of Java and deliver high-performance applications that delight users.
Remember, performance optimization is not a one-time task but an ongoing journey of refinement and improvement to stay ahead in today’s competitive landscape. So, roll up your sleeves, dive into the code, and embark on the quest for Java performance excellence!
Table of Contents
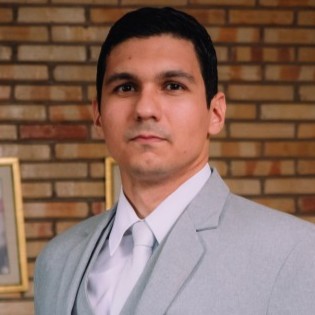
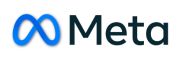