From Basics to Mastery: An In-Depth and Practical Guide to Java Programming
Java, a programming language known for its ‘write once, run anywhere’ principle, has maintained a consistent and commanding presence in the world of programming since its inception in the mid-90s. For many, Java is the gateway into the vast realm of software development, and for others, it’s the backbone of their career, powering their high-impact software solutions. This article will guide you through a comprehensive journey of mastering Java, accompanied by concrete examples to foster your understanding.
1. Introduction to Java
Java is an object-oriented, high-level programming language. Developed by Sun Microsystems (now owned by Oracle), it is designed to have as few implementation dependencies as possible. This platform independence is a key reason why many companies choose to hire Java developers – the same Java code can run on different platforms like Windows, Linux, and Mac, without any modifications. This broad compatibility reduces the complexities in software development, making Java an appealing choice for both developers and the organizations that hire them.
2. Understanding Java Basics
2.1 Variables and Data Types
Java has several data types, including integers (int), floating-point numbers (float and double), characters (char), and booleans (boolean). Let’s declare some variables.
```java int number = 10; double decimal = 5.6; char letter = 'A'; boolean isJavaFun = true; ```
2.2 Operators
Java also includes a variety of operators for performing operations on these variables. Here are a few:
```java int sum = 5 + 10; // addition int difference = 10 - 5; // subtraction int product = 5 * 10; // multiplication int quotient = 10 / 5; // division ```
2.3 Control Structures
Java uses control structures like if-else statements and loops for controlling the flow of execution. Below is an example of an if-else statement and a for loop.
```java int score = 85; // If-else statement if (score >= 90) { System.out.println("A grade"); } else if (score >= 80) { System.out.println("B grade"); } else { System.out.println("C grade"); } // For loop for (int i = 0; i < 5; i++) { System.out.println(i); } ```
3. Object-Oriented Programming in Java
Object-oriented programming (OOP) is at the heart of Java. The fundamental units of OOP are classes and objects.
3.1 Classes and Objects
A class is a blueprint from which we can create individual objects. Objects are instances of classes, containing variables and methods. Let’s define a simple class.
```java public class Car { // Class variables, also known as fields String model; int year; double price; // Class method void drive() { System.out.println("The car is driving!"); } } // Creating an object of the Car class Car car = new Car(); car.model = "Toyota"; car.year = 2022; car.price = 20000.0; // Calling the drive method car.drive(); ```
3.2 Inheritance
Inheritance allows us to define a class based on another class. It promotes code reusability and hierarchical classifications. Let’s extend the Car class with a SportsCar class.
```java public class SportsCar extends Car { // Additional field boolean isConvertible; // Additional method void race() { System.out.println("The sports car is racing!"); } } // Creating an object of the SportsCar class SportsCar sportsCar = new SportsCar(); sportsCar.model = "Ferrari"; sportsCar.year = 2023; sportsCar.price = 50000.0; sportsCar.isConvertible = true; // Calling the race method sportsCar.race(); ```
4. Exception Handling in Java
Exception handling is crucial for developing robust applications. Java uses try-catch blocks for exception handling.
```java try { int result = 10 / 0; // This will throw an ArithmeticException } catch (ArithmeticException e) { System.out.println("Caught an exception: " + e.getMessage()); } ```
5. Java’s Standard API
Java comes with a large standard library, or API (Application Programming Interface). The Java API provides a wealth of pre-written classes for performing common tasks.
5.1 Collections Framework
The Java Collections Framework provides classes for handling and manipulating groups of data as single units, like lists and sets.
```java // Creating an ArrayList List<String> list = new ArrayList<>(); list.add("Apple"); list.add("Banana"); list.add("Cherry"); // Iterating over the list for (String fruit : list) { System.out.println(fruit); } ```
5.2 JDBC API
The JDBC (Java Database Connectivity) API provides methods for querying and updating data in a database.
```java // Connecting to a database Connection conn = DriverManager.getConnection("jdbc:mysql://localhost/test", "username", "password"); // Creating a Statement Statement stmt = conn.createStatement(); // Executing a query ResultSet rs = stmt.executeQuery("SELECT * FROM fruits"); // Processing the result while (rs.next()) { System.out.println(rs.getString("name")); } ```
Concluding Remarks
Mastering Java opens up a world of possibilities in the field of software development. From creating simple command-line applications to intricate web systems, the power of Java is limitless. Companies often hire Java developers due to the language’s robustness and versatility. The examples provided in this article offer a glimpse into the language’s capabilities that a proficient Java developer should master. Yet, there’s so much more to learn and explore. Whether you’re looking to boost your skills or aiming to hire Java developers, a comprehensive understanding of the language is crucial. So, keep practicing, keep exploring, and you will indeed master Java. Happy coding!
Table of Contents
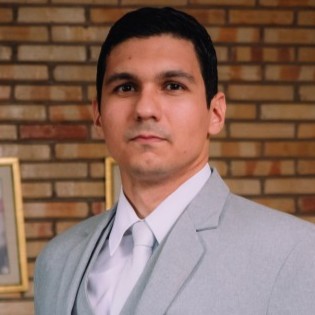
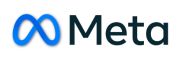