Java Reflection API: Dynamic Runtime Introspection
In the vast landscape of Java programming, there exists a powerful tool often overlooked by many developers: the Java Reflection API. This dynamic runtime introspection mechanism allows Java code to examine and manipulate the structure, behavior, and metadata of classes, interfaces, fields, and methods at runtime. In this post, we’ll delve into the intricacies of the Java Reflection API, explore its capabilities, and provide real-world examples of its usage.
Understanding Reflection in Java
Reflection in Java refers to the ability of a program to examine or “reflect” upon itself and manipulate its own structure and behavior at runtime. This powerful feature allows developers to inspect and modify classes, fields, methods, and other components dynamically, without prior knowledge of their names or types at compile time.
Key Components of the Reflection API
Class Objects
The java.lang.Class class represents classes and interfaces in Java. Through reflection, you can obtain Class objects for any class by using the .class syntax or by calling the getClass() method on an instance of that class.
Field Reflection
With reflection, you can inspect and modify the fields (variables) within a class dynamically. The java.lang.reflect.Field class provides methods to access and manipulate fields at runtime.
Method Reflection
Reflection enables you to invoke methods on objects dynamically. The java.lang.reflect.Method class facilitates the invocation of methods at runtime, including methods with various parameters.
Constructor Reflection
Reflection allows you to create new instances of classes dynamically by invoking constructors. The java.lang.reflect.Constructor class provides methods to work with constructors at runtime.
Real-World Examples
Dynamically Loading Classes
Reflection is commonly used in frameworks and libraries that rely on dynamically loading classes at runtime. For instance, frameworks like Spring and Hibernate utilize reflection to instantiate classes based on configuration files or annotations.
Serialization and Deserialization
Reflection plays a crucial role in Java’s serialization and deserialization mechanisms. The Java Object Serialization API uses reflection to examine the fields of an object and serialize them into a byte stream, enabling objects to be transmitted over networks or stored persistently.
Testing and Debugging
Reflection can be valuable in testing and debugging scenarios. Testing frameworks like JUnit utilize reflection to identify and execute test methods dynamically, based on annotations or naming conventions. Similarly, debugging tools leverage reflection to inspect the runtime state of objects and classes during debugging sessions.
Conclusion
The Java Reflection API offers developers a powerful means of introspection and dynamic manipulation of classes, fields, methods, and constructors at runtime. While reflection provides flexibility and extensibility to Java applications, it should be used judiciously due to its potential performance overhead and complexity.
By mastering the Java Reflection API, developers can unlock new possibilities for dynamic code generation, configuration, and customization in their Java projects. Whether it’s dynamically loading classes, serializing objects, or enhancing testing and debugging capabilities, reflection proves to be an invaluable tool in the Java developer’s arsenal.
External Resources:
- Oracle Java Reflection API Documentation
- Baeldung – Guide to Java Reflection API
- Java Reflection: A Practical Overview
Now armed with a deeper understanding of the Java Reflection API and its real-world applications, developers can harness its power to create more dynamic, flexible, and efficient Java applications. Happy reflecting!
Table of Contents
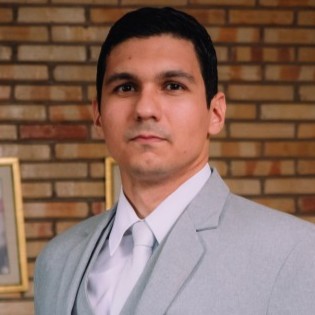
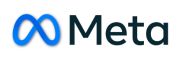