Java Unit Testing Frameworks: JUnit and TestNG
Unit testing is a crucial part of software development, ensuring that individual components of an application work as intended. In the Java ecosystem, JUnit and TestNG are two widely used frameworks for unit testing. This article explores the key features, benefits, and differences between JUnit and TestNG, along with practical examples to help you choose the right tool for your testing needs.
Understanding Unit Testing in Java
Unit testing involves testing individual components of an application in isolation to verify that they work correctly. It is a fundamental practice that helps developers catch bugs early in the development process, ensuring higher code quality and maintainability.
JUnit: The Classic Java Testing Framework
JUnit is one of the most popular unit testing frameworks in the Java community. It is simple, easy to use, and integrates well with many development environments. JUnit is often the first choice for developers who need a straightforward solution for testing Java applications.
Example: Writing a Simple JUnit Test
Here’s a basic example of a JUnit test case that checks if a method returns the expected result.
```java import org.junit.Test; import static org.junit.Assert.assertEquals; public class CalculatorTest { @Test public void testAdd() { Calculator calculator = new Calculator(); int result = calculator.add(2, 3); assertEquals(5, result); } } ```
In this example, the `testAdd` method tests the `add` method of a `Calculator` class, verifying that it returns the correct sum.
TestNG: A Flexible Alternative
TestNG is another powerful testing framework that extends the capabilities of JUnit. It offers more flexibility, such as support for parallel test execution, dependency testing, and advanced configuration options. TestNG is often preferred in complex projects where additional features are required.
Example: Writing a Simple TestNG Test
Here’s how you can write a similar test using TestNG:
```java import org.testng.annotations.Test; import static org.testng.Assert.assertEquals; public class CalculatorTest { @Test public void testAdd() { Calculator calculator = new Calculator(); int result = calculator.add(2, 3); assertEquals(result, 5); } } ```
TestNG tests are similar to JUnit tests, but TestNG offers additional annotations and configurations that allow for more sophisticated testing scenarios.
Comparing JUnit and TestNG
Both JUnit and TestNG are excellent choices for Java unit testing, but they have some key differences that may influence your decision:
– Annotations: TestNG offers a broader set of annotations, such as `@BeforeSuite`, `@AfterSuite`, `@BeforeTest`, and `@AfterTest`, which provide greater control over the test execution lifecycle.
– Parallel Execution: TestNG supports parallel execution of test methods, classes, and suites, which can significantly speed up test execution in large projects.
– Dependency Testing: TestNG allows tests to be dependent on other tests, enabling more complex test scenarios. JUnit does not support this feature natively.
– Parameterized Tests: Both frameworks support parameterized tests, but the implementation differs. JUnit uses `@ParameterizedTest` while TestNG uses the `@DataProvider` annotation for more flexible data-driven testing.
Example: Parameterized Tests in JUnit and TestNG
# JUnit Parameterized Test Example ```java import org.junit.Test; import org.junit.runner.RunWith; import org.junit.runners.Parameterized; import java.util.Arrays; import java.util.Collection; import static org.junit.Assert.assertEquals; @RunWith(Parameterized.class) public class CalculatorParameterizedTest { private int input1; private int input2; private int expected; public CalculatorParameterizedTest(int input1, int input2, int expected) { this.input1 = input1; this.input2 = input2; this.expected = expected; } @Parameterized.Parameters public static Collection<Object[]> data() { return Arrays.asList(new Object[][] { { 1, 1, 2 }, { 2, 2, 4 }, { 3, 3, 6 } }); } @Test public void testAdd() { Calculator calculator = new Calculator(); assertEquals(expected, calculator.add(input1, input2)); } } ``` # TestNG Parameterized Test Example ```java import org.testng.annotations.DataProvider; import org.testng.annotations.Test; import static org.testng.Assert.assertEquals; public class CalculatorTest { @DataProvider(name = "addData") public Object[][] createAddData() { return new Object[][] { { 1, 1, 2 }, { 2, 2, 4 }, { 3, 3, 6 } }; } @Test(dataProvider = "addData") public void testAdd(int input1, int input2, int expected) { Calculator calculator = new Calculator(); assertEquals(calculator.add(input1, input2), expected); } } ```
Conclusion
Both JUnit and TestNG are powerful frameworks for unit testing in Java, and the choice between them depends on your specific needs. JUnit is a great option for straightforward testing, while TestNG provides additional flexibility for more complex test scenarios. Understanding the strengths and features of each framework will help you make an informed decision and enhance the quality of your Java applications.
Further Reading:
Table of Contents
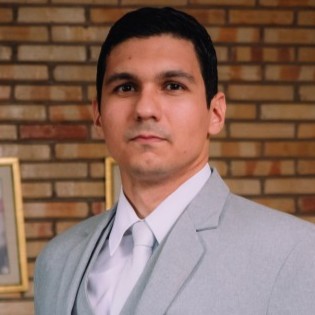
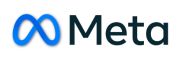