Java Web Development: Servlets, JSP, and JSTL
Java provides a robust framework for web development, allowing developers to create dynamic, scalable, and maintainable web applications. This blog explores the core components of Java web development: Servlets, JavaServer Pages (JSP), and JavaServer Pages Standard Tag Library (JSTL). We will delve into their functionalities, how they integrate, and provide practical examples to enhance your web development skills.
Understanding Servlets, JSP, and JSTL
- Servlets are Java classes that handle HTTP requests and generate responses. They form the foundation of Java web applications by managing request processing and interaction with backend logic.
- JSP (JavaServer Pages) allows developers to create dynamic web content using a mix of HTML and Java code. JSP simplifies the process of generating dynamic content and separates presentation from business logic.
- JSTL (JavaServer Pages Standard Tag Library) provides a set of custom tags for JSP that simplifies common tasks like iteration, conditionals, and formatting. JSTL enhances the functionality of JSP by offering pre-built tags for frequently used operations.
Using Servlets for Request Handling
Servlets are essential for handling HTTP requests in Java web applications. They process client requests, interact with backend systems, and generate responses. Below is an example of a simple servlet that handles GET requests.
Example: Simple Servlet for Handling GET Requests
```java import java.io.IOException; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; @WebServlet("/hello") public class HelloServlet extends HttpServlet { @Override protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { response.setContentType("text/html"); response.getWriter().println("<h1>Hello, World!</h1>"); } } ```
Creating Dynamic Web Pages with JSP
JSP allows embedding Java code into HTML pages, making it easier to generate dynamic web content. It separates the presentation layer from the business logic, promoting cleaner code and better maintainability.
Example: Basic JSP Page
```jsp <%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>JSP Example</title> </head> <body> <h1>Welcome to JSP!</h1> <p>The current time is: <%= new java.util.Date() %></p> </body> </html> ```
Utilizing JSTL for Simplified Tagging
JSTL provides a set of tags that simplify common tasks in JSP. These tags include functionalities for iteration, conditionals, and formatting, which help reduce the amount of Java code required in JSP pages.
Example: Using JSTL for Iteration
First, include JSTL library in your project. Then, use JSTL tags in your JSP page to iterate over a list of items.
```jsp <%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>JSTL Example</title> </head> <body> <h1>Item List</h1> <ul> <c:forEach var="item" items="${itemList}"> <li>${item}</li> </c:forEach> </ul> </body> </html> ```
Integrating Servlets with JSP and JSTL
Servlets, JSP, and JSTL often work together in Java web applications. Servlets handle the request processing, while JSP pages use JSTL to present dynamic content. This integration ensures a clean separation of concerns between request handling and presentation.
Example: Servlet Passing Data to JSP
```java import java.io.IOException; import java.util.ArrayList; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; @WebServlet("/items") public class ItemsServlet extends HttpServlet { @Override protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { ArrayList<String> items = new ArrayList<>(); items.add("Item 1"); items.add("Item 2"); items.add("Item 3"); request.setAttribute("itemList", items); request.getRequestDispatcher("/items.jsp").forward(request, response); } } ```
Conclusion
Java provides a powerful suite of technologies for web development, including Servlets, JSP, and JSTL. By mastering these components, you can build dynamic, maintainable, and scalable web applications. Servlets manage request processing, JSP generates dynamic content, and JSTL simplifies common tasks in JSP. Leveraging these tools effectively will lead to robust web solutions and enhanced development efficiency.
Further Reading:
Table of Contents
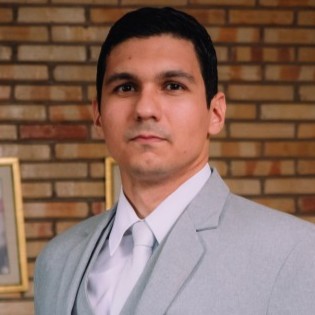
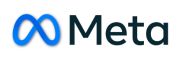