Practical Examples of JavaScript Anonymous Functions
In JavaScript, anonymous functions play a crucial role in enhancing code modularity and flexibility. Also known as “function expressions,” they allow us to define and use functions without specifying a function name. These nameless functions are particularly useful in scenarios where a function is used only once or where we need to pass functions as arguments to other functions. In this blog, we’ll explore practical examples of anonymous functions and demonstrate how they can improve your JavaScript code.
Table of Contents
1. Introduction to Anonymous Functions
In JavaScript, functions are first-class objects, meaning they can be assigned to variables, passed as arguments to other functions, and returned from functions. Anonymous functions, as the name suggests, lack an explicit name and are defined without any identifier. Instead, they are typically assigned to variables or used directly in function calls.
2. Basic Syntax of Anonymous Functions
The syntax for an anonymous function can take a couple of forms, but the most common one is using the function keyword without an identifier:
javascript const anonymousFunction = function() { // Function body };
Alternatively, with the introduction of ES6, we have the shorter syntax using arrow functions:
javascript const anonymousArrowFunction = () => { // Function body };
3. Using Anonymous Functions as Event Handlers
One of the most common use cases for anonymous functions is as event handlers. When we want to respond to a specific event, like a button click, we can define an anonymous function inline to handle the event:
html <button id="myButton">Click Me</button> <script> document.getElementById("myButton").addEventListener("click", function() { alert("Button clicked!"); }); </script>
In this example, when the button with the ID “myButton” is clicked, the anonymous function will be executed, showing an alert.
4. Self-Invoking Anonymous Functions (Immediately Invoked Function Expressions)
Self-invoking anonymous functions, also known as Immediately Invoked Function Expressions (IIFEs), are functions that execute immediately after their definition. They are often used to protect the scope of variables and avoid polluting the global scope.
4.1. Protecting Scope
Consider the following example:
html <script> for (var i = 0; i < 5; i++) { setTimeout(function() { console.log(i); }, 1000); } </script>
What do you expect this code to output? Most people would anticipate “0, 1, 2, 3, 4” with a one-second delay between each log. However, it prints “5” five times instead. This happens because the setTimeout callback functions share the same variable i, and by the time the callbacks are executed, the loop has already finished, leaving i with the value of 5.
To fix this, we can use an IIFE to create a new scope for each iteration:
html <script> for (var i = 0; i < 5; i++) { (function(index) { setTimeout(function() { console.log(index); }, 1000); })(i); } </script>
Now, the output will be the expected “0, 1, 2, 3, 4” with the one-second delay between each log.
4.2. Creating Private Variables
Another use of IIFEs is to create private variables in JavaScript. JavaScript lacks a native way of declaring private variables, but with IIFEs, we can achieve this effect:
javascript const counter = (function() { let count = 0; return { increment: function() { count++; }, get: function() { return count; } }; })(); console.log(counter.get()); // Output: 0 counter.increment(); console.log(counter.get()); // Output: 1
In this example, the variable count is enclosed within the IIFE, making it inaccessible from outside the function. However, the returned object provides public methods to interact with the private variable, allowing us to maintain its integrity.
4.3. Avoiding Global Pollution
Anonymous functions can also be utilized to prevent global scope pollution by limiting the exposure of variables:
html <script> (function() { const secretData = "Sensitive information"; // Some code that uses secretData })(); // secretData is not accessible from here </script>
By wrapping the code in an anonymous function, we ensure that secretData doesn’t leak into the global scope and remains inaccessible outside the function.
5. Callback Functions and Asynchronous Operations
Callback functions are an essential aspect of asynchronous programming in JavaScript. Anonymous functions are often used as callback functions for various asynchronous operations.
5.1. setTimeout with Anonymous Function
The setTimeout function allows us to schedule a function to be executed after a specified delay:
javascript console.log("Start"); setTimeout(function() { console.log("Delayed log after 2 seconds"); }, 2000); console.log("End");
The output will be:
mathematica Start End Delayed log after 2 seconds
Since JavaScript is single-threaded, this mechanism is crucial for non-blocking behavior, ensuring that other tasks can continue while waiting for the timeout to expire.
5.2. Array Iteration Methods
Anonymous functions are frequently employed with array iteration methods like forEach, map, filter, etc. Let’s see an example using map:
javascript const numbers = [1, 2, 3, 4, 5]; const squaredNumbers = numbers.map(function(number) { return number ** 2; }); console.log(squaredNumbers); // Output: [1, 4, 9, 16, 25]
In this case, the anonymous function is used as a mapping function to square each element in the numbers array.
6. Closures with Anonymous Functions
Closures are a powerful concept in JavaScript, and anonymous functions often come into play when dealing with closures.
Consider the following example:
javascript function outerFunction() { const message = "Hello, "; function innerFunction(name) { console.log(message + name); } return innerFunction; } const greeting = outerFunction(); greeting("John"); // Output: Hello, John
In this example, innerFunction is an anonymous function, and it forms a closure with its outer function outerFunction. The message variable in the outer function is still accessible even after outerFunction has finished executing, thanks to the closure. This allows innerFunction to access message and concatenate it with the provided name.
7. The Power of Arrow Functions
ES6 introduced arrow functions, which are a concise way to write anonymous functions. Arrow functions have a more straightforward syntax and automatically capture the surrounding context’s this value.
7.1. Concise Syntax
Compare the traditional anonymous function and the equivalent arrow function:
javascript // Traditional anonymous function const multiply = function(a, b) { return a * b; }; // Equivalent arrow function const multiplyArrow = (a, b) => a * b;
Arrow functions omit the function keyword and curly braces when the function body consists of a single expression. The result is implicitly returned, making the code more concise.
7.2. Lexical ‘this’
One of the main advantages of arrow functions is their lexical this binding. In traditional anonymous functions, this refers to the calling context, which can lead to unexpected behavior:
javascript const person = { name: "John", sayHello: function() { console.log("Hello, " + this.name); } }; person.sayHello(); // Output: Hello, John const sayHello = person.sayHello; sayHello(); // Output: Hello, undefined
In the second case, when calling sayHello as a standalone function, this is not bound to person, resulting in undefined. However, with arrow functions, this is lexically scoped, retaining the value from the surrounding context:
javascript const person = { name: "John", sayHello: function() { setTimeout(() => { console.log("Hello, " + this.name); }, 1000); } }; person.sayHello(); // Output: Hello, John
8. Benefits and Drawbacks of Anonymous Functions
Before we conclude, let’s summarize the benefits and drawbacks of using anonymous functions:
8.1. Benefits
Enhanced modularity: Anonymous functions allow for encapsulating functionality without the need for a named function declaration.
Improved code readability: They can make code more concise and expressive, especially when used as callback functions.
Scope management: IIFEs and closures with anonymous functions help control variable scope, reducing the risk of global pollution.
8.2. Drawbacks
Debugging challenges: Debugging anonymous functions can be harder since they lack a clear identifier in stack traces.
Code reusability: Anonymous functions, especially IIFEs, are not reusable as they are designed to be self-invoking or immediately executed.
Conclusion
In this blog, we’ve explored practical examples of JavaScript anonymous functions and their applications in various scenarios. Anonymous functions provide a powerful tool to enhance code organization, modularity, and maintainability. By understanding how to leverage their capabilities effectively, you can write more efficient and cleaner JavaScript code. Whether you use them as event handlers, IIFEs, or arrow functions, mastering anonymous functions will undoubtedly level up your JavaScript skills and open doors to more sophisticated programming techniques. Happy coding!
Remember, JavaScript is an ever-evolving language, and new features may have been introduced since my knowledge cutoff in September 2021. Stay curious and always keep exploring the latest updates in the world of JavaScript. Happy coding!
Table of Contents
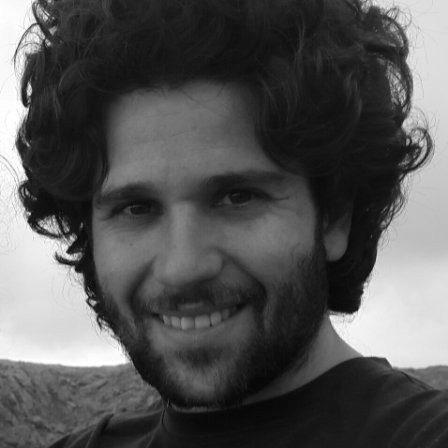
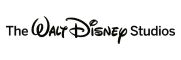