Empowering Your Code: A Deep Dive into JavaScript Arrow Functions
JavaScript, an ubiquitous and versatile language of the web, has evolved greatly since its inception, continually refining its syntax and introducing new features. One such transformative feature is the Arrow Function. When you hire JavaScript developers, you expect them to be proficient with these modern aspects of the language. Introduced with ECMAScript 6 (ES6) in 2015, Arrow Functions have proven to be a game-changer, providing not just a shorter, cleaner syntax, but also lexical scoping of `this`. Understanding and implementing this powerful feature is essential in supercharging your JavaScript code. Let’s dive into it and explore its potential.
Understanding Traditional Functions
Before delving into arrow functions, it’s crucial to comprehend traditional JavaScript function syntax. Here’s a basic example of a traditional function:
```javascript function addNumbers(a, b) { return a + b; } console.log(addNumbers(2, 3)); // Outputs: 5 ```
Here, `addNumbers` is a function that takes two arguments, `a` and `b`, and returns their sum. Although quite simple and functional, the syntax is verbose.
Introduction to Arrow Functions
In contrast, the arrow function syntax offers a leaner, more streamlined alternative. The equivalent of the previous traditional function in arrow function form is:
```javascript let addNumbers = (a, b) => a + b; console.log(addNumbers(2, 3)); // Outputs: 5 ```
Note the transformation: the `function` keyword is replaced by an arrow (`=>`) placed between the argument list and the function body. If the function body consists of just a single statement, you can omit the brackets and the `return` keyword.
Single Argument Arrow Functions
Arrow functions simplify further with single argument functions. For such functions, parentheses around the parameter can be omitted. This makes arrow functions perfect for many array methods like `map()`, `filter()`, etc.
```javascript let numbers = [1, 2, 3, 4, 5]; // ES5 syntax let squaresES5 = numbers.map(function (n) { return n * n; }); console.log(squaresES5); // Outputs: [1, 4, 9, 16, 25] // ES6 syntax let squaresES6 = numbers.map(n => n * n); console.log(squaresES6); // Outputs: [1, 4, 9, 16, 25] ```
In the above example, the `map()` function in ES6 is much cleaner and easier to understand.
Arrow Functions with No Arguments
If your function doesn’t take any arguments, you need to include an empty pair of parentheses before the arrow:
```javascript let getGreeting = () => "Hello, world!"; console.log(getGreeting()); // Outputs: Hello, world! ```
Multi-line Arrow Functions
If an arrow function has a more complicated body, it can be expanded to include multiple lines, encapsulated within `{}` brackets. In this case, you must explicitly use the `return` statement if your function needs to return a value:
```javascript let divideNumbers = (a, b) => { if (b === 0) { return 'Cannot divide by zero!'; } return a / b; }; console.log(divideNumbers(20, 5)); // Outputs: 4 console.log(divideNumbers(20, 0)); // Outputs: Cannot divide by zero! ```
Lexical Scoping of `this` with Arrow Functions
Arrow functions handle `this` differently from traditional functions. In a traditional function, `this` is determined by how the function is called (its execution context). This behavior can lead to unexpected results.
```javascript function Timer() { this.seconds = 0; setInterval(function() { this.seconds++; }, 1000); } let timer = new Timer(); setTimeout(function() { console.log(timer.seconds); }, 3100); // Outputs: 0 ```
In the example above, `this` in `this.seconds++` does not refer to the Timer instance but the `window` object (or `undefined` in strict mode), leading to unexpected output. This issue is often resolved by hacks like `_this = this` or `.bind(this)`.
On the other hand, arrow functions resolve `this` lexically. That means they inherit `this` from the surrounding (parent) code where they are defined. The above code, re-written using an arrow function, works as expected:
```javascript function Timer() { this.seconds = 0; setInterval(() => { this.seconds++; }, 1000); } let timer = new Timer(); setTimeout(() => { console.log(timer.seconds); }, 3100); // Outputs: 3 ```
Here, `this` inside the arrow function refers to the Timer instance as expected. This lexical scoping of `this` is one of the most powerful features of arrow functions.
Conclusion
Arrow functions are a potent tool in modern JavaScript, providing more than just a shorter syntax. They simplify the function scope and make the handling of `this` more predictable. This feature, combined with others introduced with ES6, aids in writing cleaner, more maintainable, and robust JavaScript code. Thus, when you hire JavaScript developers, ensure they are adept at leveraging these features. Supercharge your JavaScript with arrow functions and enjoy the elevated coding experience!
Table of Contents
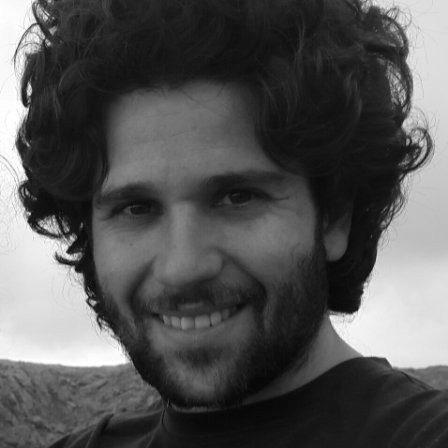
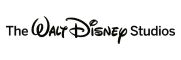