JavaScript Async Functions: Handling Asynchronous Operations
Understanding Asynchronous Programming in JavaScript
Asynchronous programming is a key feature in JavaScript, allowing developers to execute non-blocking operations. This is essential for tasks like fetching data from APIs, reading files, or handling timers without freezing the UI or blocking the event loop.
The Power of Async Functions
JavaScript introduced `async` functions in ES2017 as a modern way to handle asynchronous code. They simplify the use of promises, making asynchronous code more readable and maintainable. An `async` function returns a promise and allows the use of the `await` keyword to pause the execution until the promise is resolved or rejected.
1. Using Async and Await
The `async` and `await` keywords work together to make asynchronous code look synchronous. This makes it easier to write, read, and debug.
Example: Fetching Data from an API
```javascript async function fetchData(url) { try { let response = await fetch(url); if (!response.ok) { throw new Error('Network response was not ok'); } let data = await response.json(); console.log(data); } catch (error) { console.error('There was a problem with the fetch operation:', error); } } fetchData('https://api.example.com/data'); ```
In this example, the `fetchData` function is declared as `async`, allowing the use of `await` to handle the `fetch` promise. This way, the response is only processed after the data is fully retrieved.
2. Handling Errors in Async Functions
Error handling in async functions is straightforward using `try…catch` blocks. This ensures that errors are caught and managed within the function.
Example: Error Handling with Async/Await
```javascript async function getUserData(userId) { try { let response = await fetch(`https://api.example.com/users/${userId}`); if (!response.ok) { throw new Error('User not found'); } let user = await response.json(); return user; } catch (error) { console.error('Error fetching user data:', error); throw error; // Re-throwing the error for further handling if needed } } getUserData(1) .then(user => console.log(user)) .catch(error => console.log('Caught an error:', error)); ```
In this example, the error is caught in the `getUserData` function and re-thrown for further handling by the caller.
3. Combining Multiple Async Functions
Async functions can be composed to handle multiple asynchronous operations sequentially or concurrently.
Example: Sequential Async Operations
```javascript async function getWeatherAndNews() { try { let weather = await fetchWeather(); let news = await fetchNews(); console.log('Weather:', weather); console.log('News:', news); } catch (error) { console.error('Error fetching data:', error); } } async function fetchWeather() { let response = await fetch('https://api.example.com/weather'); return response.json(); } async function fetchNews() { let response = await fetch('https://api.example.com/news'); return response.json(); } getWeatherAndNews(); ```
In this scenario, the `getWeatherAndNews` function waits for each operation to complete before proceeding, ensuring the data is processed in the correct order.
4. Async Functions and Promises: A Comparison
Before `async/await`, developers used promises to manage asynchronous code. While promises are still valid, `async/await` provides a more straightforward syntax, reducing the need for chained `.then()` calls and nested callbacks.
Example: Promises vs. Async/Await
Using Promises:
```javascript fetch('https://api.example.com/data') .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error)); ```
Using Async/Await:
```javascript async function fetchData() { try { let response = await fetch('https://api.example.com/data'); let data = await response.json(); console.log(data); } catch (error) { console.error('Error:', error); } } fetchData(); ```
The `async/await` approach eliminates the need for chaining and provides a clearer and more linear flow.
Conclusion
JavaScript’s `async` functions have revolutionized asynchronous programming, making it easier to write clean, readable, and maintainable code. By mastering `async/await`, developers can handle asynchronous operations efficiently, leading to more responsive applications.
Further Reading:
Table of Contents
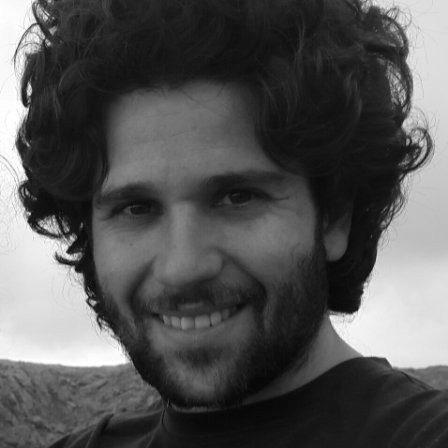
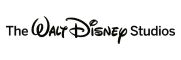