JavaScript Promises: Simplifying Asynchronous Functionality
As web applications and JavaScript codebases grow in complexity, so does the need to handle asynchronous tasks effectively. Traditionally, handling asynchronous operations in JavaScript was a challenge, leading to callback hell, where deeply nested callbacks made the code hard to read and maintain. However, with the introduction of JavaScript Promises, asynchronous programming has been simplified and made more manageable. In this blog post, we will explore JavaScript Promises and how they revolutionized asynchronous functionality, making it easier to handle complex tasks.
Table of Contents
Understanding Asynchronous Programming
Before diving into JavaScript Promises, let’s briefly review what asynchronous programming is and why it’s essential. JavaScript, as a single-threaded language, executes code sequentially, one operation at a time. However, certain operations can take longer to complete, such as making API calls, reading files, or querying a database. In synchronous code, the application would have to wait for these time-consuming tasks to finish before moving on to the next operation, causing the entire application to freeze.
Asynchronous programming allows the JavaScript engine to perform other tasks while waiting for non-blocking operations to complete. This way, the application remains responsive and can handle multiple operations simultaneously.
Traditionally, developers used callbacks to manage asynchronous code. A callback is a function passed as an argument to another function, and it is executed when the asynchronous operation completes. While callbacks work, chaining multiple callbacks for sequential tasks led to the callback hell problem, as shown in the example below:
javascript getDataFromAPI(function (data) { processData(data, function (result) { saveData(result, function (response) { // More nested callbacks... }); }); });
As you can see, the nested structure quickly becomes unwieldy, making the code difficult to read and maintain. This is where JavaScript Promises come to the rescue!
Introducing JavaScript Promises
A Promise in JavaScript represents a value that may not be available yet but will be resolved at some point in the future. Promises can have three states:
- Pending: The initial state. The Promise is still in progress and hasn’t been fulfilled or rejected yet.
- Fulfilled: The asynchronous operation was successful, and the Promise is resolved with a value.
- Rejected: The asynchronous operation encountered an error, and the Promise is rejected with a reason for the failure.
Using Promises, we can create more structured and readable asynchronous code without resorting to deeply nested callbacks. Let’s take a look at the same example as before, but this time using Promises:
javascript getDataFromAPI() .then(processData) .then(saveData) .then(response => { // Handle the final response }) .catch(error => { // Handle errors });
Isn’t this a significant improvement? Promises allow us to chain asynchronous operations together, making the code flow more linear and easier to understand. Each .then() block represents a step in the asynchronous process, and we can use a final .catch() block to handle any errors that might occur during the process.
Creating Promises
To create a Promise in JavaScript, we use the Promise constructor, which takes a function as an argument. This function, called the “executor,” has two arguments: resolve and reject. These are functions that are used to fulfill or reject the Promise, respectively.
Here’s a simple example of creating a Promise:
javascript const delay = (ms) => new Promise((resolve) => setTimeout(resolve, ms)); delay(2000) .then(() => console.log("Two seconds have passed!")) .catch((error) => console.error("Error:", error));
In this example, we define a function delay that returns a Promise that resolves after a given number of milliseconds. The resolve function is called after the specified delay, and we log a message to the console. If any error occurs during the Promise execution, it will be caught and logged using the catch block.
Chaining Promises
One of the most powerful features of Promises is the ability to chain them together. Each then block in the chain receives the result of the previous Promise and returns a new Promise. This allows us to perform sequential operations with ease.
javascript const fetchUser = () => { return new Promise((resolve, reject) => { // Simulate fetching user data from an API setTimeout(() => { const user = { id: 1, name: "John Doe" }; resolve(user); }, 1000); }); }; const fetchUserPosts = (user) => { return new Promise((resolve, reject) => { // Simulate fetching user posts from an API using the user object setTimeout(() => { const posts = ["Post 1", "Post 2", "Post 3"]; resolve({ user, posts }); }, 1500); }); }; fetchUser() .then(fetchUserPosts) .then(({ user, posts }) => console.log(`${user.name} has ${posts.length} posts.`)) .catch((error) => console.error("Error:", error));
In this example, we have two functions: fetchUser and fetchUserPosts, each returning a Promise that resolves with data fetched from an API (simulated by a setTimeout function). We use .then() to chain these functions together, accessing the user data in the second .then() block and logging the result to the console.
Handling Errors with Promises
Handling errors is essential in any application. With Promises, error handling becomes more straightforward using the .catch() block at the end of the chain.
javascript const fetchUserData = () => { return new Promise((resolve, reject) => { // Simulate fetching user data from an API setTimeout(() => { const error = new Error("Failed to fetch user data."); reject(error); }, 1000); }); }; fetchUserData() .then((data) => console.log(data)) .catch((error) => console.error("Error:", error.message));
In this example, the fetchUserData function returns a Promise that immediately rejects with an error. The error is caught in the .catch() block, and the error message is logged to the console.
Running Promises in Parallel
Promises are not limited to sequential execution. In many cases, we might want to run multiple asynchronous operations simultaneously and wait for all of them to complete. JavaScript provides a utility function called Promise.all(), which takes an array of Promises and returns a new Promise that resolves when all Promises in the array are fulfilled, or rejects if any of the Promises are rejected.
javascript const fetchUserData = () => { return new Promise((resolve) => { // Simulate fetching user data from an API setTimeout(() => { const user = { id: 1, name: "John Doe" }; resolve(user); }, 1000); }); }; const fetchUserPosts = () => { return new Promise((resolve) => { // Simulate fetching user posts from an API setTimeout(() => { const posts = ["Post 1", "Post 2", "Post 3"]; resolve(posts); }, 1500); }); }; Promise.all([fetchUserData(), fetchUserPosts()]) .then(([user, posts]) => console.log(`${user.name} has ${posts.length} posts.`)) .catch((error) => console.error("Error:", error));
In this example, we have two separate functions that return Promises: fetchUserData and fetchUserPosts. We use Promise.all() to run both functions simultaneously and handle the results in the .then() block once both Promises are fulfilled.
Conclusion
JavaScript Promises have significantly simplified asynchronous programming in JavaScript, enabling developers to write cleaner and more maintainable code. By eliminating callback hell and offering a more structured way to handle asynchronous tasks, Promises have become an essential part of modern JavaScript development. As you continue to build complex web applications, mastering Promises will empower you to create more efficient and reliable asynchronous functionality.
In this blog post, we’ve only scratched the surface of what Promises can do. To further enhance your skills, consider exploring other Promise methods, such as Promise.race(), async/await, and how to handle errors more effectively. Happy coding!
Table of Contents
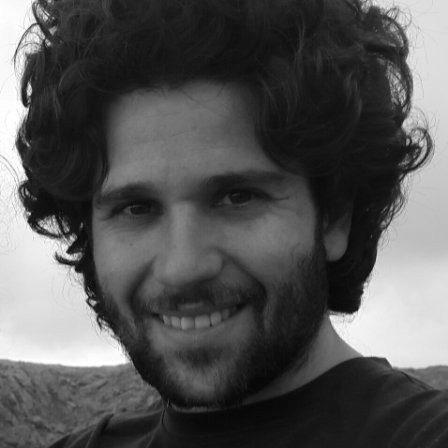
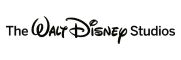