Mastering JavaScript Callback Hell: Strategies for Clean Code
In the world of JavaScript programming, dealing with asynchronous operations is a common challenge. Callbacks have long been the traditional way to handle these operations, but they can quickly lead to a tangled mess of nested functions known as “callback hell.” As your codebase grows, managing callback hell becomes increasingly challenging, making code readability, maintainability, and debugging a nightmare.
Table of Contents
Fear not! In this article, we’ll dive deep into the concept of callback hell, understand why it happens, and explore effective strategies to tame this beast and produce clean, manageable code. We’ll cover various techniques, including the use of named functions, modularization, Promises, and async/await. By the end of this guide, you’ll be equipped with the tools to conquer callback hell and write code that’s not only functional but also elegant and maintainable.
1. Understanding Callback Hell
Before we delve into the solutions, let’s understand why callback hell occurs. Callback hell arises due to the nesting of asynchronous functions within one another, often resulting in deeply indented code that’s hard to read and maintain. This can lead to a multitude of issues, such as:
- Readability: Deeply nested code becomes difficult to understand, making it challenging for you and your team to grasp the logic behind the implementation.
- Debugging: Identifying errors and tracing their sources becomes a tedious task when you’re dealing with a convoluted structure of callbacks.
- Maintainability: As your project grows, maintaining callback-heavy code becomes increasingly complex. Adding new features or modifying existing ones becomes risky and error-prone.
- Scalability: Callback hell can hinder the scalability of your application. It becomes harder to refactor and adapt your codebase to accommodate changes and enhancements.
2. Strategies for Clean Code
2.1. Named Functions
One effective way to tackle callback hell is by using named functions. Instead of providing anonymous functions as callbacks, define named functions and pass them as references. This approach enhances code readability and encourages modularization.
javascript function fetchData(url, onSuccess, onError) { // Simulate fetching data const data = { /* fetched data */ }; if (data) { onSuccess(data); } else { onError("Error fetching data"); } } function displayData(data) { // Display data on the UI } function handleError(error) { // Handle and display errors } fetchData("https://example.com/api/data", displayData, handleError);
By employing named functions, the code structure becomes cleaner, and each function’s responsibility is well-defined, making it easier to manage.
2.2. Modularization
Dividing your code into smaller, modular functions can significantly alleviate callback hell. Break down complex tasks into separate functions and connect them through callbacks. This not only simplifies your code but also promotes reusability and maintainability.
javascript function fetchUserData(userId, onSuccess, onError) { // Fetch user data } function fetchUserPosts(userId, onSuccess, onError) { // Fetch user's posts } function displayUserInfo(userData) { // Display user data on the UI } function displayUserPosts(posts) { // Display user's posts on the UI } const userId = "123"; fetchUserData(userId, (userData) => { displayUserInfo(userData); fetchUserPosts(userId, displayUserPosts, handleError); }, handleError);
By compartmentalizing functionality into modular functions, you can concentrate on individual tasks, making the codebase more comprehensible and adaptable.
2.3. Promises
ES6 introduced Promises as a more structured and readable way to handle asynchronous operations. Promises allow you to chain asynchronous calls, eliminating the need for deep nesting and indentation.
javascript function fetchData(url) { return new Promise((resolve, reject) => { // Simulate fetching data const data = { /* fetched data */ }; if (data) { resolve(data); } else { reject("Error fetching data"); } }); } fetchData("https://example.com/api/data") .then((data) => { // Process data }) .catch((error) => { // Handle errors });
Promises enhance code readability by structuring the flow of asynchronous operations sequentially. They also offer the advantage of handling errors in a centralized manner using the .catch() block.
2.4. Async/Await
The async/await syntax builds on top of Promises and provides a more synchronous-like way to write asynchronous code. It combines the power of Promises with a cleaner and more intuitive syntax.
javascript async function fetchData(url) { try { // Simulate fetching data const data = { /* fetched data */ }; return data; } catch (error) { throw new Error("Error fetching data"); } } async function processAsyncOperations() { try { const data = await fetchData("https://example.com/api/data"); // Process data } catch (error) { // Handle errors } } processAsyncOperations();
Using async/await, you can write asynchronous code that resembles synchronous code, making it easier to follow the logical flow of your program.
Conclusion
Callback hell is a notorious pitfall in JavaScript development, but armed with the right strategies, you can conquer it and write clean, maintainable, and efficient code. By embracing techniques like named functions, modularization, Promises, and async/await, you can transform complex asynchronous operations into elegant solutions that are easier to understand, debug, and maintain. Remember, writing code is not just about functionality—it’s about crafting a masterpiece of logic that’s both powerful and readable. So go forth, and master the art of taming callback hell!
As you embark on your journey to cleaner code, keep practicing and exploring advanced topics. The JavaScript landscape is constantly evolving, and your commitment to enhancing your skills will set you on the path to becoming a proficient and sought-after JavaScript developer.
Table of Contents
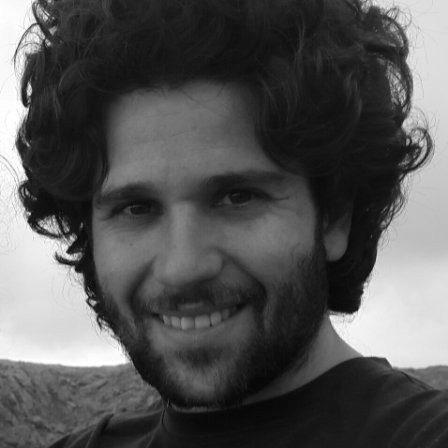
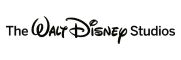