JavaScript Function Chaining Libraries: Streamlining Method Cascading
Function chaining, also known as method cascading, is a technique that allows multiple method calls to be linked together in a single statement. This approach enhances code readability and maintainability by creating a more fluent and expressive API. In JavaScript, several libraries facilitate function chaining, making it easier to perform complex operations in a clean and efficient manner. This article explores how JavaScript function chaining libraries can streamline your code and provides practical examples using popular libraries.
Understanding Function Chaining
Function chaining involves invoking multiple methods on the same object in a sequential manner. This technique reduces the need for intermediate variables and improves code clarity by creating a more natural flow of operations.
Popular JavaScript Function Chaining Libraries
Several JavaScript libraries offer robust function chaining capabilities. Here, we’ll explore three popular ones: Lodash, Underscore, and Ramda.
1. Lodash
Lodash is a widely-used utility library that provides a variety of functions for manipulating arrays, objects, and other data types. Its method chaining feature allows for elegant and expressive code.
Example: Chaining with Lodash
```javascript const _ = require('lodash'); const data = [1, 2, 3, 4, 5]; const result = _.chain(data) .map(n => n 2) .filter(n => n > 5) .reduce((sum, n) => sum + n, 0) .value(); console.log(result); // Output: 18 ```
In this example, Lodash’s `chain` method is used to create a chainable object. Methods like `map`, `filter`, and `reduce` are then applied in sequence, and `value` is called to get the final result.
2. Underscore
Underscore is another utility library similar to Lodash, offering function chaining capabilities to streamline method calls.
Example: Chaining with Underscore
```javascript const _ = require('underscore'); const data = [1, 2, 3, 4, 5]; const result = _.chain(data) .map(n => n 3) .filter(n => n < 15) .reduce((sum, n) => sum + n, 0) .value(); console.log(result); // Output: 30 ```
Underscore’s `chain` function works similarly to Lodash, allowing you to build complex chains of methods and obtain the final result with `value`.
3. Ramda
Ramda is a functional programming library that emphasizes immutability and currying. Its function chaining capabilities align well with functional programming principles.
Example: Chaining with Ramda
```javascript const R = require('ramda'); const data = [1, 2, 3, 4, 5]; const result = R.pipe( R.map(n => n 4), R.filter(n => n % 2 === 0), R.reduce((sum, n) => sum + n, 0) )(data); console.log(result); // Output: 40 ```
In this example, `R.pipe` is used to create a function pipeline, where each function is applied in sequence. Unlike method chaining, `pipe` is more aligned with functional programming practices.
Integrating Function Chaining in Projects
Function chaining libraries can be integrated into various types of JavaScript projects, including web applications, Node.js services, and more. They provide a clean and maintainable way to handle data transformations and operations.
Example: Integrating Lodash in a Web Application
```html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Function Chaining with Lodash</title> <script src="https://cdn.jsdelivr.net/npm/lodash/lodash.min.js"></script> </head> <body> <script> const data = [10, 20, 30, 40, 50]; const result = _.chain(data) .map(n => n - 5) .filter(n => n % 10 === 0) .reduce((sum, n) => sum + n, 0) .value(); console.log(result); // Output: 30 </script> </body> </html> ```
Conclusion
JavaScript function chaining libraries such as Lodash, Underscore, and Ramda offer powerful tools for streamlining method cascading and enhancing code readability. By leveraging these libraries, developers can write cleaner and more efficient code, making their applications easier to maintain and extend. Whether you’re working on a small project or a large application, incorporating function chaining can significantly improve your development experience.
Further Reading:
Table of Contents
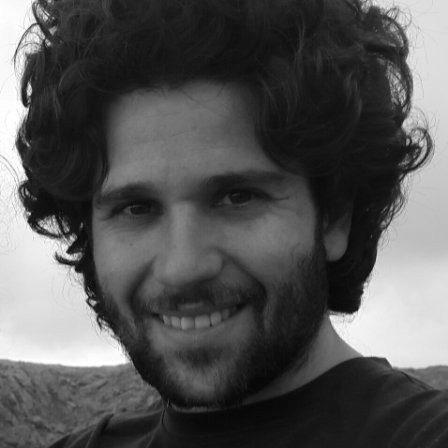
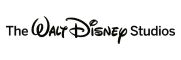