JavaScript Function Composition Libraries: A Comparative Study
Introduction to Function Composition
Function composition is a core concept in functional programming that allows developers to combine multiple functions into a single function. This technique leads to cleaner, more modular, and more maintainable code. In JavaScript, function composition can be achieved using various libraries, each offering unique features and benefits.
This article provides a comparative study of popular JavaScript function composition libraries, examining their features, performance, and ease of use. We will explore how these libraries can be leveraged to create more expressive and reusable code.
Why Function Composition Matters
Function composition helps in breaking down complex operations into smaller, reusable functions that can be combined in various ways. This leads to:
- – Modularity: Breaking down logic into small, independent functions.
- – Reusability: Reusing smaller functions across different parts of an application.
- – Readability: Creating a clear and logical flow in the codebase.
Popular JavaScript Function Composition Libraries
1. Ramda
Overview:
Ramda is a functional programming library for JavaScript that emphasizes immutability and side-effect-free functions. It offers a rich set of functions for composing functions, transforming data, and managing collections.
Features:
– Automatically curried functions.
– Point-free style enabled by default.
– Extensive support for functional programming constructs.
Example:
```javascript import { compose, map, filter } from 'ramda'; const double = x => x 2; const isEven = x => x % 2 === 0; const processNumbers = compose( map(double), filter(isEven) ); console.log(processNumbers([1, 2, 3, 4])); // [4, 8] ```
Pros:
– Strong functional programming support.
– Comprehensive and well-documented API.
Cons:
– Larger bundle size compared to more lightweight libraries.
2. Lodash/fp
Overview:
Lodash/fp is the functional programming variant of Lodash. It provides immutable, auto-curried, and composable functions, making it easy to apply functional programming principles in JavaScript.
Features:
– Curried versions of Lodash functions.
– First argument is data, allowing for easy composition.
– Familiarity for developers already using Lodash.
Example:
```javascript import { compose, map, filter } from 'lodash/fp'; const double = x => x 2; const isEven = x => x % 2 === 0; const processNumbers = compose( map(double), filter(isEven) ); console.log(processNumbers([1, 2, 3, 4])); // [4, 8] ```
Pros:
– Smaller learning curve for Lodash users.
– Lightweight compared to Ramda.
Cons:
– Less extensive than Ramda in functional programming capabilities.
3. Folktale
Overview:
Folktale is a suite of libraries for functional programming in JavaScript. It provides robust tools for handling functional programming constructs like monads, ADTs (Algebraic Data Types), and function composition.
Features:
– Monads and other FP constructs.
– Algebraic Data Types.
– Advanced function composition capabilities.
Example:
```javascript const { compose } = require('folktale/core/lambda'); const { map, filter } = require('folktale/core/fantasy-land'); const double = x => x 2; const isEven = x => x % 2 === 0; const processNumbers = compose( map(double), filter(isEven) ); console.log(processNumbers([1, 2, 3, 4])); // [4, 8] ```
Pros:
– Advanced functional programming tools.
– Strongly typed and predictable behavior.
Cons:
– Steeper learning curve for beginners.
– Larger bundle size.
Performance Considerations
When choosing a function composition library, performance is a key factor. While Ramda offers extensive features, it may introduce overhead compared to more lightweight libraries like Lodash/fp. Folktale, being feature-rich, might also impact performance depending on the complexity of the operations.
Ease of Use and Documentation
- Ramda: Comprehensive documentation, but may require a deeper understanding of functional programming concepts.
- Lodash/fp: Easier for those familiar with Lodash, with clear documentation and examples.
- Folktale: Rich documentation, but advanced features may require more time to learn.
Conclusion
Choosing the right function composition library depends on your project’s needs and your familiarity with functional programming. Ramda is ideal for those seeking a full-fledged functional programming experience, while Lodash/fp offers a more lightweight and familiar alternative. Folktale is perfect for advanced users looking for robust functional programming tools.
By understanding the strengths and weaknesses of each library, you can make an informed decision to enhance your JavaScript development with function composition.
Further Reading
Table of Contents
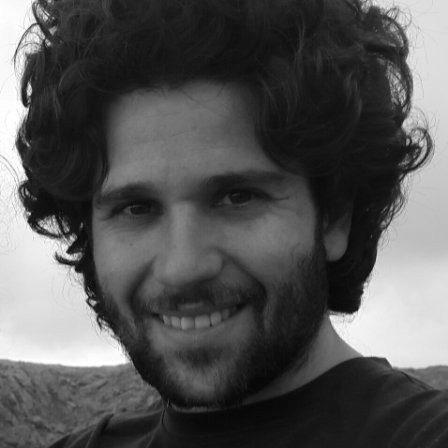
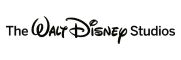