JavaScript Currying: Enhancing Function Reusability
JavaScript is a versatile and powerful language that allows developers to create dynamic and interactive web applications. One of the essential aspects of writing clean and maintainable code is reusability. Reusing code not only saves time but also makes the codebase more organized and easier to manage. In this blog, we will explore a powerful technique called “JavaScript currying” that enhances function reusability and makes our code more flexible.
Table of Contents
1. What is Currying?
Definition and Explanation
Currying is a functional programming technique that involves transforming a function that takes multiple arguments into a series of functions that take one argument each. The process of currying allows us to create new functions by fixing one or more arguments of the original function. This concept is named after the mathematician Haskell Curry, who contributed significantly to combinatory logic.
The resulting curried functions become more composable building blocks, and they can be chained together to create powerful and flexible code structures. By using currying, we can achieve a higher level of abstraction and create specialized functions with ease.
Example of Currying
Let’s illustrate currying with a simple example. Consider a function that calculates the total cost of an item based on its price, tax rate, and discount.
javascript // Non-curried function function calculateTotal(price, taxRate, discount) { return price + (price * taxRate) - discount; } // Curried function function curriedCalculateTotal(price) { return function (taxRate) { return function (discount) { return price + (price * taxRate) - discount; }; }; } // Usage of the curried function const calculateTotalCurried = curriedCalculateTotal(100); const taxRate = 0.1; const discount = 20; const totalCost = calculateTotalCurried(taxRate)(discount); console.log(totalCost); // Output: 90
In the non-curried version, we pass all three arguments at once. In contrast, the curried version breaks down the function into three nested functions, each taking a single argument. This enables us to create a partially applied function calculateTotalCurried that already knows the price argument and allows us to supply the remaining arguments (taxRate and discount) separately.
2. How Currying Works?
Partial Application
Currying and partial application are related concepts, but they are not the same. While currying transforms a function into a series of unary (single-argument) functions, partial application fixes a specific number of arguments of a function, creating a new function with reduced arity. Currying can be seen as a form of partial application, but currying creates a chain of functions, whereas partial application directly produces a new function with fewer arguments.
In the previous example, we partially applied the price argument to the curriedCalculateTotal function, resulting in the calculateTotalCurried function, which is waiting for the remaining arguments.
Currying vs. Partial Application
To clarify the difference, let’s see an example of partial application:
javascript // Original function function greet(greeting, name) { return `${greeting}, ${name}!`; } // Partially applied function const greetHello = greet.bind(null, 'Hello'); console.log(greetHello('John')); // Output: Hello, John! console.log(greetHello('Alice')); // Output: Hello, Alice!
In this example, we use Function.prototype.bind to create the greetHello function, which is a partially applied version of the greet function. We fix the first argument as ‘Hello’, and the resulting function is ready to receive only the name argument.
3. Benefits of Currying
Function Composition
Currying is a powerful technique for function composition. It allows us to build complex functionalities by combining simpler functions. With currying, we can chain functions together, creating a functional pipeline. This approach enhances the readability and maintainability of the code, as each function is focused on a specific task.
Reusability and Flexibility
Currying promotes code reusability by breaking down functions into smaller, composable parts. Once we create a curried function with fixed arguments, we can reuse it in different contexts by supplying the remaining arguments. This eliminates the need to rewrite similar functions with minor variations, leading to more efficient and maintainable code.
The flexibility offered by currying allows us to customize and modify functions easily. We can create specialized versions of a function by fixing specific arguments, tailoring it to specific use cases. This adaptability is particularly useful when dealing with APIs that require different configurations.
Customization and Specialization
Consider an example of a function that calculates the area of various shapes:
javascript // Non-curried function function calculateArea(shape, ...args) { switch (shape) { case 'rectangle': return args[0] * args[1]; case 'circle': return Math.PI * args[0] * args[0]; // More shape calculations... } } console.log(calculateArea('rectangle', 4, 5)); // Output: 20 console.log(calculateArea('circle', 3)); // Output: 28.274333882308138
In this example, the calculateArea function determines the shape and calculates the area based on the provided arguments. However, this function can quickly become unwieldy as we add more shapes. With currying, we can improve its flexibility and reusability:
javascript // Curried function for calculating areas function curriedCalculateArea(shape) { switch (shape) { case 'rectangle': return function (length) { return function (width) { return length * width; }; }; case 'circle': return function (radius) { return Math.PI * radius * radius; }; // More curried functions for other shapes... } } // Usage of curried functions const rectangleArea = curriedCalculateArea('rectangle')(4)(5); const circleArea = curriedCalculateArea('circle')(3); console.log(rectangleArea); // Output: 20 console.log(circleArea); // Output: 28.274333882308138
By currying the calculateArea function, we create specialized functions for each shape, making the code more modular and maintainable.
4. Implementing Currying in JavaScript
Manual Currying
We’ve already seen manual currying in the previous example. While it’s instructive to understand the concept, manually currying functions can be cumbersome, especially for complex functions. However, understanding the underlying principle is crucial for grasping more advanced currying techniques and how libraries implement them.
Using Libraries (Lodash, Ramda)
Several JavaScript libraries, such as Lodash and Ramda, provide built-in functions for currying and partial application. These libraries make it easier to work with curried functions, and they handle various edge cases, ensuring better performance and functionality.
Let’s take a look at how Lodash handles currying:
javascript // Using Lodash to create a curried function const lodashCurriedCalculateTotal = _.curry((price, taxRate, discount) => { return price + (price * taxRate) - discount; }); // Usage of the curried function const calculateTotalCurriedWithLodash = lodashCurriedCalculateTotal(100); const lodashTotalCost = calculateTotalCurriedWithLodash(0.1)(20); console.log(lodashTotalCost); // Output: 90
Lodash’s _.curry function takes a function as its argument and returns a new curried version of that function. It’s worth noting that Ramda’s curry function behaves similarly.
5. Real-World Use Cases
Event Handling
Currying can be especially useful in event handling scenarios. Suppose we have multiple buttons on a webpage, each with a unique action:
javascript const button1 = document.getElementById('button1'); const button2 = document.getElementById('button2'); function onButtonClick(action, event) { // Perform the action based on the button clicked } // Adding event listeners button1.addEventListener('click', (event) => onButtonClick('action1', event)); button2.addEventListener('click', (event) => onButtonClick('action2', event));
By currying the onButtonClick function, we can create specialized event handlers for each button:
javascript const button1 = document.getElementById('button1'); const button2 = document.getElementById('button2'); function curriedOnButtonClick(action) { return function (event) { // Perform the action based on the button clicked }; } // Adding event listeners using curried functions button1.addEventListener('click', curriedOnButtonClick('action1')); button2.addEventListener('click', curriedOnButtonClick('action2'));
This approach results in cleaner and more organized code, making it easier to manage various button actions.
Functional Pipelines
Currying can be an integral part of functional pipelines, which are sequences of functions applied to data to produce desired results. Each function in the pipeline is curried, allowing them to accept one argument at a time and chain together seamlessly:
javascript const add = (a, b) => a + b; const square = (x) => x * x; const subtract = (a, b) => a - b; const pipeline = (value) => subtract(square(add(value, 2)), 5); console.log(pipeline(3)); // Output: 6
In this example, each function in the pipeline is curried, enabling the creation of a concise and expressive data transformation process.
Configurable Functionality
Currying is ideal for creating configurable functions, where certain parameters can be set upfront, and other parameters can be passed later. This technique is prevalent in functional programming.
Consider a data filtering function:
javascript function filterData(criteria, data) { // Filter data based on criteria } const filterWithCriteria = filterData.bind(null, 'active'); const filteredData = filterWithCriteria(data);
With currying, we can create a more elegant solution:
javascript function curriedFilterData(criteria) { return function (data) { // Filter data based on criteria }; } const filterActiveData = curriedFilterData('active'); const filteredData = filterActiveData(data);
This enables us to create reusable filtering functions for various criteria effortlessly.
Conclusion
JavaScript currying is a powerful technique that enhances function reusability and flexibility. By breaking down functions into composable building blocks, currying allows us to create functional pipelines, improve code organization, and build configurable and specialized functions.
While manual currying is possible, using libraries like Lodash or Ramda simplifies the process and handles edge cases more effectively. Currying, in combination with functional programming principles, can significantly improve the maintainability and readability of your code, making it an essential tool for any JavaScript developer.
By employing currying judiciously in your projects, you can enhance your codebase’s modularity, reusability, and composability, leading to more efficient and maintainable applications. Happy currying!
Table of Contents
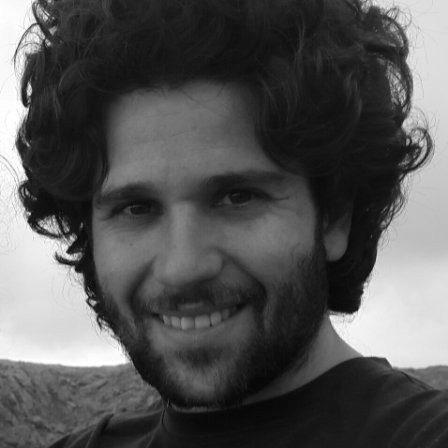
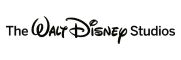