Building Custom JavaScript Array Functions for Enhanced Manipulation
Arrays are a fundamental data structure in JavaScript, and they play a crucial role in various programming tasks. From storing collections of data to performing operations like filtering, mapping, and reducing, arrays are a versatile tool. JavaScript provides a rich set of built-in array methods like forEach, map, filter, and reduce, but sometimes, your specific requirements demand custom solutions. In this guide, we’ll explore the process of building custom JavaScript array functions to enhance your array manipulation capabilities.
Table of Contents
Why Custom Array Functions?
JavaScript’s built-in array methods are incredibly powerful and cover a wide range of use cases. However, there are times when you might need to perform specialized operations or apply a custom logic to your arrays. Creating custom array functions allows you to encapsulate your unique requirements and make your code more readable, reusable, and maintainable.
Getting Started
To begin building custom array functions, you’ll need a solid understanding of basic JavaScript concepts, including functions, loops, and the structure of arrays. Additionally, a grasp of higher-order functions and callback functions will be beneficial.
Let’s start by creating a simple custom array function: a function that squares each element of an array.
javascript function squareArrayElements(arr) { const squaredArray = []; for (let i = 0; i < arr.length; i++) { squaredArray.push(arr[i] * arr[i]); } return squaredArray; } const numbers = [1, 2, 3, 4, 5]; const squaredNumbers = squareArrayElements(numbers); console.log(squaredNumbers); // Output: [1, 4, 9, 16, 25]
Anatomy of a Custom Array Function
Let’s break down the key components of the squareArrayElements function:
- Function Name: Choose a descriptive name that reflects the purpose of the custom function.
- Parameters: The function takes an array as its parameter (arr in this case).
- Logic: Inside the function, we iterate over each element of the input array using a loop and perform the desired manipulation. In this case, we’re squaring each element.
- Return Value: The manipulated array is stored in the squaredArray variable, which is then returned from the function.
Making Custom Functions More Dynamic
The example above demonstrated a simple custom array function. However, to create truly versatile and dynamic functions, we need to introduce parameters that allow us to customize the behavior.
Let’s create a more dynamic version of the custom function that accepts a callback function as a parameter. This will enable us to apply any manipulation to the array elements.
javascript function manipulateArray(arr, callback) { const manipulatedArray = []; for (let i = 0; i < arr.length; i++) { manipulatedArray.push(callback(arr[i])); } return manipulatedArray; } const numbers = [1, 2, 3, 4, 5]; // Create a callback function to square a number function square(num) { return num * num; } const squaredNumbers = manipulateArray(numbers, square); console.log(squaredNumbers); // Output: [1, 4, 9, 16, 25] // Create a callback function to calculate the cube of a number function cube(num) { return num * num * num; } const cubedNumbers = manipulateArray(numbers, cube); console.log(cubedNumbers); // Output: [1, 8, 27, 64, 125]
By accepting a callback function as a parameter, the manipulateArray function becomes highly flexible. You can now apply various manipulations to the input array, making your code more adaptable.
Implementing Common Array Operations
Let’s delve into building custom functions for common array operations: filtering and mapping.
Custom Filter Function
The built-in filter method creates a new array with all elements that pass a given test. Let’s create a custom filter function that mimics this behavior.
javascript function customFilter(arr, callback) { const filteredArray = []; for (let i = 0; i < arr.length; i++) { if (callback(arr[i])) { filteredArray.push(arr[i]); } } return filteredArray; } const numbers = [1, 2, 3, 4, 5]; // Create a callback function to filter even numbers function isEven(num) { return num % 2 === 0; } const evenNumbers = customFilter(numbers, isEven); console.log(evenNumbers); // Output: [2, 4]
Custom Map Function
The map method creates a new array populated with the results of calling a provided function on every element in the calling array. Let’s build a custom map function that achieves the same outcome.
javascript function customMap(arr, callback) { const mappedArray = []; for (let i = 0; i < arr.length; i++) { mappedArray.push(callback(arr[i])); } return mappedArray; } const numbers = [1, 2, 3, 4, 5]; // Create a callback function to double a number function double(num) { return num * 2; } const doubledNumbers = customMap(numbers, double); console.log(doubledNumbers); // Output: [2, 4, 6, 8, 10]
Going Beyond: Custom Reducer Function
The reduce method applies a function against an accumulator and each element in the array to reduce it to a single value. Creating a custom reducer function involves a slightly more complex process.
javascript function customReduce(arr, callback, initialValue) { let accumulator = initialValue === undefined ? arr[0] : initialValue; for (let i = initialValue === undefined ? 1 : 0; i < arr.length; i++) { accumulator = callback(accumulator, arr[i]); } return accumulator; } const numbers = [1, 2, 3, 4, 5]; // Create a callback function to calculate the sum function sum(a, b) { return a + b; } const totalSum = customReduce(numbers, sum, 0); console.log(totalSum); // Output: 15
Conclusion
Building custom JavaScript array functions empowers you to tailor your code to specific requirements, resulting in more efficient and readable code. By encapsulating array manipulation logic within custom functions, you enhance the reusability and maintainability of your codebase. Whether you’re working on small scripts or large-scale applications, custom array functions are an essential tool in your JavaScript toolkit. With the knowledge gained from this guide, you’re ready to dive deeper into the world of custom array functions and supercharge your JavaScript programming skills.
Table of Contents
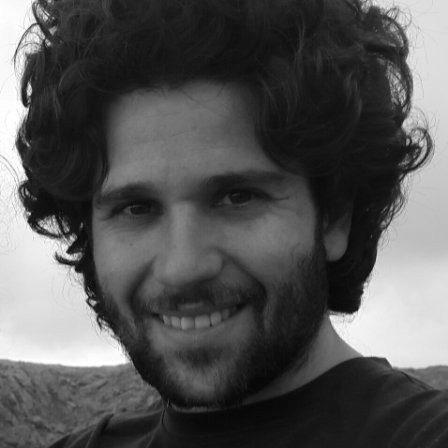
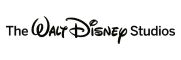