JavaScript Functions for Data Validation: Ensuring Data Integrity
Data validation is a critical aspect of maintaining data integrity in applications. Ensuring that the data entered into your system meets specific criteria helps prevent errors and inconsistencies. JavaScript, with its versatile capabilities, offers a range of functions and techniques for effective data validation. This article explores how JavaScript can be utilized for data validation and provides practical examples to ensure the integrity of your data.
Understanding Data Validation
Data validation involves checking the accuracy and quality of data before it is processed or stored. Effective validation ensures that data conforms to expected formats, ranges, and constraints, preventing issues that could arise from invalid or incomplete data.
Using JavaScript for Data Validation
JavaScript provides numerous methods and libraries to handle data validation tasks. Below are some key aspects and code examples demonstrating how JavaScript can be employed for effective data validation.
1. Validating User Input
The first step in data validation is to ensure that user input meets specific criteria. JavaScript can be used to validate form inputs, such as checking for required fields, proper email formats, and acceptable ranges.
Example: Validating an Email Address
Here’s a simple function to validate an email address using a regular expression.
```javascript function validateEmail(email) { const emailPattern = /^[^\s@]+@[^\s@]+\.[^\s@]+$/; return emailPattern.test(email); } // Example usage const email = 'user@example.com'; console.log(validateEmail(email)); // true or false ```
2. Checking Data Ranges
Ensuring that numerical data falls within a specified range is another crucial aspect of validation. JavaScript functions can be used to verify that numbers meet certain criteria, such as being within a minimum and maximum value.
Example: Checking if a Number is Within Range
Here’s a function to check if a number falls within a given range.
```javascript function isInRange(value, min, max) { return value >= min && value <= max; } // Example usage const age = 25; console.log(isInRange(age, 18, 65)); // true or false ```
3. Validating Dates
Date validation ensures that date inputs are in the correct format and fall within valid ranges. JavaScript provides date functions to help with these tasks.
Example: Validating a Date Format
This function checks if a date string is in the format `YYYY-MM-DD`.
```javascript function validateDate(dateString) { const datePattern = /^\d{4}-\d{2}-\d{2}$/; return datePattern.test(dateString) && !isNaN(new Date(dateString).getTime()); } // Example usage const date = '2024-08-15'; console.log(validateDate(date)); // true or false ```
4. Custom Validation Rules
For more complex validation needs, custom rules can be implemented using JavaScript functions. This allows for the creation of tailored validation logic that meets specific requirements.
Example: Custom Validation for a Password
Here’s a function to validate a password based on specific criteria: minimum length, inclusion of numbers, and special characters.
```javascript function validatePassword(password) { const minLength = 8; const hasNumber = /\d/; const hasSpecialChar = /[!@#$%^&]/; return password.length >= minLength && hasNumber.test(password) && hasSpecialChar.test(password); } // Example usage const password = 'SecureP@ss123'; console.log(validatePassword(password)); // true or false ```
5. Integrating with Form Validation Libraries
JavaScript libraries such as `validator.js` can simplify and enhance data validation processes. These libraries offer a range of pre-built validation functions and can be easily integrated into your application.
Example: Using validator.js for Validation
Here’s how you might use the `validator.js` library to validate an email address.
```javascript const validator = require('validator'); const email = 'user@example.com'; console.log(validator.isEmail(email)); // true or false ```
Conclusion
JavaScript offers a powerful set of tools and functions for data validation. From checking user input and data ranges to validating dates and passwords, JavaScript can help ensure the integrity of your data. By leveraging these capabilities, you can build more reliable and robust applications.
Further Reading:
Table of Contents
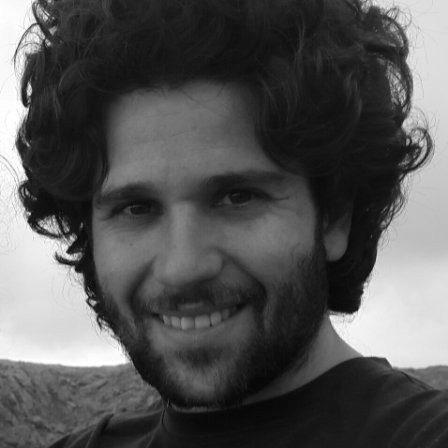
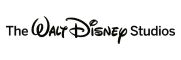