JavaScript Function Debugging: Tips and Techniques for Efficient Troubleshooting
Debugging is an essential skill for any JavaScript developer. Whether you’re building complex web applications or working on smaller projects, being able to efficiently troubleshoot and fix issues in your code can save you a lot of time and frustration. In this article, we’ll explore various techniques and tools that can help you debug JavaScript functions more effectively.
Understanding JavaScript Debugging
Debugging is the process of identifying and fixing errors or bugs in your code. In JavaScript, this often involves stepping through your code line by line, examining variables, and understanding how the code is executed. Effective debugging allows you to quickly locate the source of issues and implement fixes without disrupting the overall flow of your application.
Using `console.log()` for Quick Debugging
One of the most common and straightforward methods of debugging in JavaScript is using `console.log()`. By inserting `console.log()` statements into your code, you can output the values of variables, the results of expressions, or messages to track the flow of execution.
Example: Debugging with `console.log()`
```javascript function calculateSum(a, b) { console.log('calculateSum called with:', a, b); const sum = a + b; console.log('Sum:', sum); return sum; } calculateSum(5, 10); ```
In this example, `console.log()` helps you verify that the function is receiving the expected arguments and producing the correct output.
Using Breakpoints in Browser Developer Tools
While `console.log()` is helpful, it can clutter your code. For more controlled debugging, browser developer tools offer breakpoints, which allow you to pause code execution at specific lines and inspect the state of your application.
Example: Setting Breakpoints in Chrome DevTools
- Open your web application in Google Chrome.
- Right-click on the line number where you want to set a breakpoint and select “Add breakpoint.”
- Reload the page or trigger the function, and the execution will pause at your breakpoint.
- Use the “Step over,” “Step into,” and “Step out” controls to navigate through your code line by line.
Inspecting Variables with the Watch Panel
The Watch panel in browser developer tools allows you to keep an eye on specific variables and expressions as you debug your code. This feature is particularly useful when you need to monitor the value of a variable as it changes throughout the execution of your program.
Example: Using the Watch Panel
- Open the developer tools in your browser.
- Navigate to the “Sources” tab.
- Add variables or expressions to the Watch panel by clicking the “+” button and typing the variable name.
- As you step through your code, the Watch panel will update with the current values.
Using `debugger` Statements
The `debugger` statement is another powerful tool for debugging JavaScript functions. When the JavaScript engine encounters a `debugger` statement, it will pause execution and bring up the debugging tools, allowing you to inspect the current state of your code.
Example: Using the `debugger` Statement
```javascript function calculateProduct(a, b) { debugger; // Execution will pause here const product = a * b; return product; } calculateProduct(4, 7); ```
In this example, placing the `debugger` statement inside the `calculateProduct` function will automatically trigger a breakpoint when the function is called.
Analyzing Call Stacks and Understanding Function Execution Flow
When debugging complex applications, understanding the call stack is crucial. The call stack shows the order in which functions are called, helping you trace the execution flow and pinpoint where errors occur.
Example: Analyzing the Call Stack
- Set a breakpoint in a function.
- Trigger the function to pause execution.
- Navigate to the “Call Stack” panel in your browser’s developer tools.
- Examine the list of functions in the call stack to understand the order of execution.
Using Error Handling for Better Debugging
Implementing error handling in your code can also aid in debugging. By using `try-catch` blocks, you can catch and handle errors gracefully, preventing your application from crashing and making it easier to identify the source of the issue.
Example: Using `try-catch` for Error Handling
```javascript function divide(a, b) { try { if (b === 0) { throw new Error('Cannot divide by zero'); } return a / b; } catch (error) { console.error('Error:', error.message); } } divide(10, 0); ```
In this example, the `try-catch` block catches the error and logs it to the console, allowing you to debug the issue without the application crashing.
Conclusion
Debugging JavaScript functions efficiently is key to maintaining smooth and functional code. Whether you use `console.log()`, browser developer tools, `debugger` statements, or error handling, these techniques will help you troubleshoot problems more effectively. By mastering these tools and techniques, you can save time, reduce frustration, and improve the overall quality of your code.
Further Reading
Table of Contents
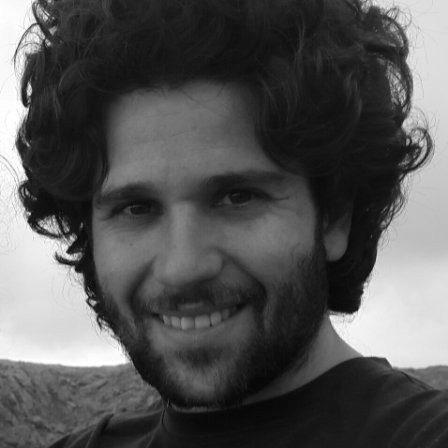
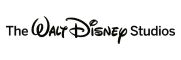