Simplifying DOM Manipulation with JavaScript Helper Functions
Modern web development heavily relies on dynamically updating web pages and creating interactive user interfaces. To achieve this, developers often need to manipulate the Document Object Model (DOM) – the structured representation of a web page’s content – using JavaScript. While DOM manipulation can be powerful, it can also be complex and error-prone, especially as projects grow in size and complexity. In this article, we’ll explore how you can simplify DOM manipulation using JavaScript helper functions, making your front-end development experience more efficient and enjoyable.
Table of Contents
1. Understanding the Challenge of DOM Manipulation
DOM manipulation involves tasks like creating, deleting, modifying, and traversing elements in a web page. While JavaScript provides native methods for these tasks, they can become cumbersome to use, especially when dealing with complex manipulations. Consider the following scenario: you want to toggle the visibility of a set of elements when a button is clicked. Using vanilla JavaScript, you might end up with code like this:
javascript const button = document.querySelector('#toggleButton'); const elements = document.querySelectorAll('.toggleElement'); button.addEventListener('click', () => { elements.forEach(element => { if (element.style.display === 'none') { element.style.display = 'block'; } else { element.style.display = 'none'; } }); });
While this code achieves the desired functionality, it’s not very readable and can be error-prone when modifying or extending. Helper functions can provide a cleaner and more maintainable solution.
2. The Power of Helper Functions
Helper functions are reusable blocks of code that perform specific tasks. They encapsulate complex logic into easy-to-use functions, enhancing code readability and reducing duplication. When it comes to DOM manipulation, creating helper functions can significantly simplify your codebase and make your development process smoother.
2.1. Creating a Helper Function for Element Selection
A common task in DOM manipulation is selecting elements by their ID, class, or tag name. Instead of repeatedly using document.querySelector or document.querySelectorAll, you can create a helper function that abstracts this process. Here’s an example:
javascript function selectElement(selector) { return document.querySelector(selector); } // Usage const button = selectElement('#toggleButton'); const elements = selectElement('.toggleElement');
By using the selectElement function, you centralize the selection logic and make it easier to update in the future.
3. Adding and Removing CSS Classes
Manipulating CSS classes is another frequent task when working with the DOM. Adding, removing, or toggling classes can become more intuitive with helper functions. Consider the following example:
javascript function addClass(element, className) { element.classList.add(className); } function removeClass(element, className) { element.classList.remove(className); } function toggleClass(element, className) { element.classList.toggle(className); } // Usage const targetElement = selectElement('.target'); addClass(targetElement, 'highlight'); setTimeout(() => { removeClass(targetElement, 'highlight'); }, 2000);
By encapsulating class manipulation within functions, you enhance the clarity of your code and reduce the risk of introducing errors.
4. Simplifying Event Handling
Handling events is an essential part of creating interactive web applications. Instead of attaching event listeners directly to elements, you can create helper functions that abstract event handling. This promotes consistency and allows for easier management of multiple events. Here’s an example:
javascript function addClickListener(element, callback) { element.addEventListener('click', callback); } // Usage const button = selectElement('#submitButton'); addClickListener(button, () => { // Perform actions on button click });
5. Building Complex Actions
As your web application grows, you’ll encounter scenarios where you need to perform more complex actions involving multiple DOM elements and interactions. Helper functions can help streamline these tasks and ensure your code remains organized.
5.1. Creating Dynamic Content
Generating dynamic content based on user input or data is a common requirement. Instead of manually creating and appending elements, you can use a helper function to streamline the process:
javascript function createAndAppendElement(parent, elementType, content) { const element = document.createElement(elementType); element.textContent = content; parent.appendChild(element); return element; } // Usage const container = selectElement('.container'); const newItem = createAndAppendElement(container, 'div', 'New Item');
This function not only creates elements but also appends them to the specified parent, saving you from repetitive code.
6. AJAX Requests Made Easier
Fetching data from a server using AJAX is an integral part of modern web development. While libraries like fetch simplify AJAX requests, helper functions can abstract even more complexity:
javascript function fetchJSON(url, options = {}) { return fetch(url, options) .then(response => response.json()) .catch(error => console.error('Error:', error)); } // Usage fetchJSON('https://api.example.com/data') .then(data => { // Handle the fetched JSON data });
The fetchJSON function encapsulates the entire process of making an AJAX request and parsing the JSON response.
7. Embracing Reusability and Readability
The true value of JavaScript helper functions lies in their reusability and improved readability. By encapsulating common DOM manipulation tasks into functions, you can build a more efficient and maintainable codebase. Here are some best practices to keep in mind:
- Modularization: Organize your helper functions into separate files based on their functionality. This makes it easier to manage and maintain your codebase as it grows.
- Documentation: Provide clear comments and documentation for your helper functions. This helps other developers (and your future self) understand how to use the functions correctly.
- Testing: Test your helper functions thoroughly to ensure they work as expected in various scenarios. This reduces the likelihood of introducing bugs into your application.
- Version Control: If you’re working on a team or open-source project, consider managing your helper functions as a separate library or package. This enables easier sharing and updating across different projects.
Conclusion
DOM manipulation is a fundamental aspect of front-end development, but it doesn’t have to be a daunting task. JavaScript helper functions offer a way to simplify and streamline your code, making it more readable, maintainable, and efficient. By creating functions that encapsulate common tasks, you’ll spend less time on repetitive chores and more time building the interactive and engaging web applications users expect.
So why not start integrating helper functions into your development workflow today? Your future self (and your fellow developers) will thank you for it as your projects continue to evolve and thrive. Happy coding!
Table of Contents
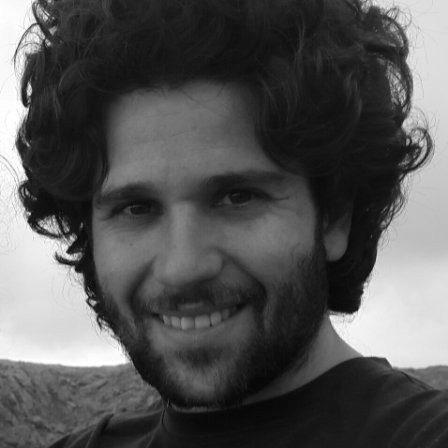
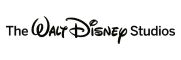