JavaScript Error Handling: Best Practices for Function Exception Handling
In the world of software development, errors are inevitable. No matter how well we write our code, there will always be scenarios where unexpected situations arise, leading to errors. JavaScript, being a widely-used programming language in web development, is no exception to this reality. However, the key to creating robust and reliable applications lies in how effectively we handle these errors.
Table of Contents
In this blog post, we’ll explore the best practices for JavaScript error handling in functions. We’ll cover various techniques and strategies that can help you identify, catch, and handle exceptions gracefully, ensuring smoother application flow and enhanced user experience. So, let’s dive in!
Understanding JavaScript Errors
Before we delve into error handling techniques, it’s crucial to understand the different types of errors that can occur in JavaScript. Common errors include:
- Syntax Errors: These occur when there are mistakes in the code syntax, such as missing semicolons or parentheses. The code fails to compile or interpret due to these errors.
- Runtime Errors: Also known as exceptions, these errors occur during the execution of the code. They often result from logical mistakes, improper data types, or accessing properties of undefined or null variables.
- Logical Errors: These are the most challenging errors to detect as they don’t throw any exceptions. Instead, they cause unintended behavior in the program, leading to incorrect results.
The try-catch Statement
One of the fundamental constructs for error handling in JavaScript is the try-catch statement. It allows us to attempt a risky piece of code within the try block, and if an error occurs, the catch block is executed, enabling us to handle the exception gracefully.
javascript function divide(a, b) { try { if (b === 0) { throw new Error("Division by zero is not allowed."); } return a / b; } catch (error) { console.error("Error: ", error.message); return NaN; } } console.log(divide(10, 2)); // Output: 5 console.log(divide(10, 0)); // Output: Error: Division by zero is not allowed. NaN
In the above example, we have a divide function that attempts to divide two numbers. If b is equal to zero, we manually throw an Error using the throw statement. The catch block catches this error, logs the error message, and returns NaN to indicate the operation’s failure.
Properly Logging Errors
While the catch block in the previous example does log the error message, it’s essential to have a robust error logging mechanism in place, especially in production environments. Proper error logging allows developers to identify and address issues swiftly, leading to better maintenance and debugging.
There are several ways to log errors, depending on the environment:
1. Browser Console:
In web applications, we can log errors to the browser console using console.error() or console.log(). However, keep in mind that logs in the browser console are visible to end-users, so avoid logging sensitive information.
javascript function fetchUserData(userId) { // Simulate an API call setTimeout(() => { const user = { id: userId, name: "John Doe" }; if (!user.name) { console.error("User name not found."); } else { console.log("User data:", user); } }, 1000); } fetchUserData(123);
In the above example, the fetchUserData function fetches user data and logs the result or an error message to the console, based on whether the user’s name exists.
2. Server-side Logging:
In server-side environments, such as Node.js, you can use various logging libraries like Winston, Bunyan, or Morgan to log errors to files or external logging services.
javascript const winston = require("winston"); // Create a logger const logger = winston.createLogger({ level: "error", format: winston.format.json(), transports: [ new winston.transports.File({ filename: "error.log" }), ], }); function processOrder(order) { try { // Process the order if (!order.isValid()) { throw new Error("Invalid order data."); } } catch (error) { logger.error(error.message); } }
In this example, we use Winston to create a logger and then log the error message in the processOrder function.
3. Remote Logging Services:
For real-time monitoring and analysis of errors in production, you can integrate remote logging services like Loggly, Sentry, or LogRocket.
Graceful Degradation with Default Values
In certain scenarios, you can handle errors gracefully by providing default values or fallbacks. This technique is especially useful when dealing with external data or user inputs, where the data’s integrity cannot be guaranteed.
javascript function findUser(username) { const users = { john_doe: "John Doe", alice_wonderland: "Alice Wonderland" }; return users[username] || "User not found"; } console.log(findUser("john_doe")); // Output: John Doe console.log(findUser("unknown_user")); // Output: User not found
In the above example, the findUser function attempts to retrieve the user’s name based on the provided username. If the username doesn’t exist in the users object, the function returns a default value, “User not found,” instead of throwing an error.
Specific Error Handling
When dealing with multiple types of errors in a function, it’s beneficial to handle each error specifically. This approach allows you to provide customized responses or actions for different error scenarios.
javascript function readJSON(jsonString) { try { const data = JSON.parse(jsonString); if (!data || typeof data !== "object") { throw new TypeError("Invalid JSON data."); } return data; } catch (error) { if (error instanceof SyntaxError) { console.error("SyntaxError: Malformed JSON."); } else if (error instanceof TypeError) { console.error("TypeError: Invalid JSON data."); } else { console.error("Unknown error occurred:", error.message); } return null; } }
In this example, the readJSON function attempts to parse a JSON string. If the string is not valid JSON or not an object, specific errors are thrown. The catch block then handles each error type separately, providing relevant error messages.
Propagating Errors
In certain situations, you may want to handle an error at a higher level rather than dealing with it immediately. In such cases, you can propagate the error to a higher level by rethrowing it or throwing a new error.
javascript function processOrder(order) { try { if (!order.isValid()) { throw new Error("Invalid order data."); } // Process the order } catch (error) { // Log the error and rethrow it console.error("Error processing order:", error.message); throw error; } } try { const order = getOrderFromAPI(); processOrder(order); } catch (error) { console.error("Error occurred while processing the order:", error.message); }
In this example, the processOrder function throws an error if the order data is invalid. The error is logged, and then rethrown, so it can be caught and handled at a higher level, outside the function.
Using Promises for Error Handling
Asynchronous JavaScript operations, such as API calls or file I/O, often return Promises. When dealing with asynchronous code, using try-catch blocks alone may not suffice, as errors inside a Promise’s then block cannot be caught with regular catch statements.
In such cases, it’s essential to use the .catch() method on the Promise to handle errors.
javascript function fetchDataFromAPI(url) { return fetch(url) .then((response) => { if (!response.ok) { throw new Error("Failed to fetch data from the API."); } return response.json(); }) .catch((error) => { console.error("Error fetching data:", error.message); throw error; }); } fetchDataFromAPI("https://api.example.com/data") .then((data) => { console.log("Data fetched successfully:", data); }) .catch((error) => { console.error("Error occurred while fetching data:", error.message); });
In this example, the fetchDataFromAPI function fetches data from an API using the fetch function. If the API request is unsuccessful, an error is thrown inside the then block. The error is then caught using the .catch() method to handle the error and propagate it further.
Conclusion
Effective error handling is an essential aspect of writing reliable and robust JavaScript code. By following the best practices discussed in this blog post, you can enhance your function’s exception handling, leading to better application stability and improved user experience. Remember to use the try-catch statement for synchronous code, log errors properly, and handle errors at appropriate levels.
Always strive to provide meaningful error messages and graceful fallbacks to ensure your application gracefully handles unexpected situations. Additionally, when working with asynchronous operations, make sure to use Promises and the .catch() method to handle errors effectively.
By adopting these best practices, you’ll be well-equipped to write more resilient and error-tolerant JavaScript functions, making your applications more dependable and easier to maintain in the long run. Happy coding!
Table of Contents
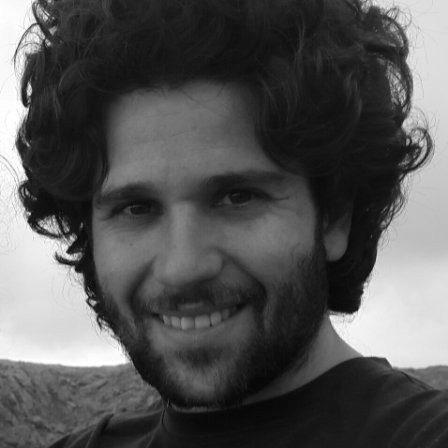
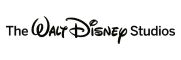