JavaScript Functions in ES6: Exploring New Syntax and Features
JavaScript’s ES6 (ECMAScript 2015) update brought significant enhancements to the language, especially in how functions are written and used. These new features make JavaScript code more concise, readable, and powerful. This article explores the key improvements in ES6 for JavaScript functions, providing practical examples to help you understand and apply these modern techniques.
Understanding ES6 Function Syntax
ES6 introduced several new features for functions, including arrow functions, default parameters, rest parameters, and more. These enhancements improve code clarity and reduce the potential for errors.
1. Arrow Functions
Arrow functions are a shorthand way to write functions in JavaScript. They are particularly useful for simplifying function expressions and improving the readability of your code.
Example: Simplifying a Function with Arrow Syntax
Here’s how you can transform a regular function into an arrow function:
```javascript // Traditional function expression const add = function(a, b) { return a + b; }; // Arrow function expression const add = (a, b) => a + b; ```
Arrow functions have a more concise syntax, and they also have the added benefit of lexical `this` binding, meaning they inherit `this` from the surrounding code context.
2. Default Parameters
Default parameters allow you to set default values for function arguments, which are used if no argument is provided or if `undefined` is passed.
Example: Using Default Parameters
```javascript function greet(name = 'Guest') { return `Hello, ${name}!`; } console.log(greet()); // Output: Hello, Guest! console.log(greet('Alice')); // Output: Hello, Alice! ```
This feature helps avoid undefined values and makes functions more flexible.
3. Rest Parameters
Rest parameters allow you to represent an indefinite number of arguments as an array. This is especially useful when working with functions that require a variable number of arguments.
Example: Collecting Arguments with Rest Parameters
```javascript function sum(...numbers) { return numbers.reduce((acc, num) => acc + num, 0); } console.log(sum(1, 2, 3)); // Output: 6 console.log(sum(10, 20, 30, 40)); // Output: 100 ```
Rest parameters make it easier to handle multiple arguments without needing to use the `arguments` object.
4. The `spread` Operator in Function Calls
The spread operator (`…`) allows you to expand an array into individual elements, which can be passed as arguments to a function.
Example: Expanding Arrays with the Spread Operator
```javascript const numbers = [1, 2, 3]; function multiply(a, b, c) { return a b c; } console.log(multiply(...numbers)); // Output: 6 ```
This feature simplifies the process of passing array elements as function arguments.
5. Enhanced Object Literals for Methods
ES6 allows for a more concise syntax when defining methods in object literals, eliminating the need for the `function` keyword.
Example: Defining Methods in Objects
```javascript const calculator = { add(a, b) { return a + b; }, multiply(a, b) { return a b; } }; console.log(calculator.add(2, 3)); // Output: 5 console.log(calculator.multiply(4, 5)); // Output: 20 ```
This feature makes objects with methods more concise and easier to read.
Conclusion
The introduction of new syntax and features for functions in ES6 has significantly improved the way JavaScript developers write code. From arrow functions to rest parameters, these enhancements offer greater flexibility, readability, and power. By mastering these features, you can write more efficient and maintainable JavaScript code, taking full advantage of modern JavaScript.
Further Reading
Table of Contents
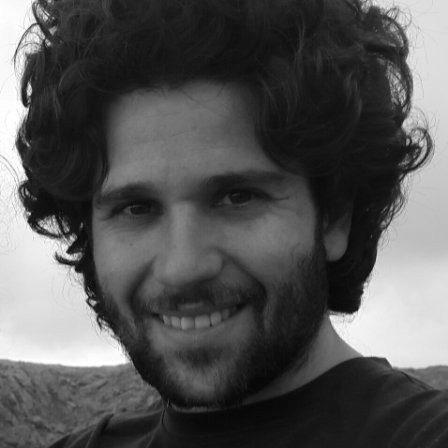
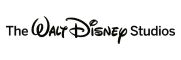