JavaScript Event Handlers: Building Interactive Functionality
In the realm of web development, interactivity is the key to engaging user experiences. A static webpage can only do so much to captivate visitors, but with the help of JavaScript event handlers, developers can breathe life into their creations. Event handlers are an essential part of JavaScript that allow us to respond to user actions, such as mouse clicks, keyboard inputs, or touch events, enabling us to build dynamic and interactive functionality. In this blog, we will delve into the world of JavaScript event handlers, exploring their capabilities and learning how to harness their power to create interactive web pages.
Table of Contents
1. Understanding Event Handlers
1.1 What are Event Handlers?
Event handlers are blocks of code that allow JavaScript to “listen” and respond to various events triggered by users interacting with a webpage. These events can be mouse clicks, keyboard inputs, form submissions, or even page loading. With event handlers, we can execute specific actions or functions when the corresponding event occurs.
1.2 How Do Event Handlers Work?
At the core of event handling in JavaScript is the EventListener interface. This interface represents an object that can handle an event dispatched by the DOM (Document Object Model) or other interfaces. By associating event handlers with specific events, developers can instruct the browser to execute particular code when those events take place.
2. Event Types and Common Use Cases
2.1 Click Events
Click events are one of the most common types of events used in web development. They occur when a user clicks on an HTML element, such as a button or a link. Let’s see an example of a simple click event handler:
html <!DOCTYPE html> <html> <head> <title>Click Event Example</title> </head> <body> <button id="myButton">Click Me!</button> <script> const button = document.getElementById('myButton'); button.addEventListener('click', () => { alert('Button clicked!'); }); </script> </body> </html>
2.2 Key Events
Key events allow developers to capture keyboard inputs from users. They are useful for implementing features like search functionality, keyboard shortcuts, or validating user inputs in forms.
html <!DOCTYPE html> <html> <head> <title>Key Event Example</title> </head> <body> <input type="text" id="searchInput"> <script> const searchInput = document.getElementById('searchInput'); searchInput.addEventListener('keyup', (event) => { console.log(`Key "${event.key}" was pressed.`); }); </script> </body> </html>
2.3 Form Events
Form events are relevant when working with HTML forms, such as capturing data when the user submits a form or validating input fields.
html <!DOCTYPE html> <html> <head> <title>Form Event Example</title> </head> <body> <form id="myForm"> <input type="text" name="username" required> <button type="submit">Submit</button> </form> <script> const form = document.getElementById('myForm'); form.addEventListener('submit', (event) => { event.preventDefault(); const formData = new FormData(form); const username = formData.get('username'); alert(`Hello, ${username}! Form submitted successfully.`); }); </script> </body> </html>
2.4 Mouse Events
Mouse events are used to track user interactions with the mouse, such as hovering over an element or dragging and dropping.
html <!DOCTYPE html> <html> <head> <title>Mouse Event Example</title> </head> <body> <div id="myDiv" style="width: 200px; height: 100px; background-color: lightblue;"></div> <script> const myDiv = document.getElementById('myDiv'); myDiv.addEventListener('mouseover', () => { myDiv.style.backgroundColor = 'orange'; }); myDiv.addEventListener('mouseout', () => { myDiv.style.backgroundColor = 'lightblue'; }); </script> </body> </html>
2.5 Touch Events
Touch events are specifically designed for touchscreen devices and are essential for building mobile-friendly web applications.
html <!DOCTYPE html> <html> <head> <title>Touch Event Example</title> </head> <body> <div id="touchDiv" style="width: 200px; height: 100px; background-color: lightgreen;"></div> <script> const touchDiv = document.getElementById('touchDiv'); touchDiv.addEventListener('touchstart', () => { touchDiv.style.backgroundColor = 'yellow'; }); touchDiv.addEventListener('touchend', () => { touchDiv.style.backgroundColor = 'lightgreen'; }); </script> </body> </html>
3. Registering Event Handlers
3.1 Inline Event Handlers
One way to register event handlers is by using inline event attributes directly in HTML elements. Though easy to implement, this approach can lead to code clutter and maintenance issues.
html <!DOCTYPE html> <html> <head> <title>Inline Event Handler Example</title> </head> <body> <button onclick="alert('Hello from inline event!')">Click Me!</button> </body> </html>
3.2 Using DOM Properties
We can also attach event handlers using DOM properties. Although this approach is better than inline event handlers, it still presents limitations when dealing with multiple events.
html <!DOCTYPE html> <html> <head> <title>DOM Properties Event Handler Example</title> </head> <body> <button id="myButton">Click Me!</button> <script> const button = document.getElementById('myButton'); button.onclick = () => { alert('Hello from DOM property event!'); }; </script> </body> </html>
3.3 addEventListener Method
The preferred and most flexible way to register event handlers is by using the addEventListener method. This method allows you to attach multiple event listeners to a single element and easily remove them when no longer needed.
html <!DOCTYPE html> <html> <head> <title>addEventListener Example</title> </head> <body> <button id="myButton">Click Me!</button> <script> const button = document.getElementById('myButton'); button.addEventListener('click', () => { alert('Hello from addEventListener!'); }); </script> </body> </html>
4. Event Object and Event Propagation
4.1 Accessing Event Object
When an event occurs, the browser creates an event object that holds information about the event. This object can be accessed inside event handlers and provides valuable data related to the event.
html <!DOCTYPE html> <html> <head> <title>Event Object Example</title> </head> <body> <button id="myButton">Click Me!</button> <script> const button = document.getElementById('myButton'); button.addEventListener('click', (event) => { console.log(event); }); </script> </body> </html>
4.2 Event Propagation: Capturing and Bubbling
Event propagation refers to the order in which events are handled when an element has nested elements with attached event handlers. There are two phases in event propagation: capturing and bubbling.
When an event occurs on a deeply nested element, the event first enters the capturing phase, triggering event handlers attached to the ancestor elements. After reaching the target element, the event enters the bubbling phase, triggering event handlers attached to ancestor elements in reverse order.
html <!DOCTYPE html> <html> <head> <title>Event Propagation Example</title> </head> <body> <div id="outer"> <div id="middle"> <div id="inner">Click Me!</div> </div> </div> <script> const outer = document.getElementById('outer'); const middle = document.getElementById('middle'); const inner = document.getElementById('inner'); const logEvent = (event) => { console.log(event.target.id); }; outer.addEventListener('click', logEvent, true); middle.addEventListener('click', logEvent, true); inner.addEventListener('click', logEvent, true); </script> </body> </html>
5. Event Delegation: Enhancing Performance
Event delegation is a powerful concept that improves performance, especially when dealing with a large number of elements. Instead of attaching event handlers to each individual element, you attach a single event handler to a common ancestor and let events bubble up from the target element. You then determine which element triggered the event and act accordingly.
html <!DOCTYPE html> <html> <head> <title>Event Delegation Example</title> </head> <body> <ul id="myList"> <li>Item 1</li> <li>Item 2</li> <li>Item 3</li> <!-- Add more list items here --> </ul> <script> const myList = document.getElementById('myList'); myList.addEventListener('click', (event) => { if (event.target.tagName === 'LI') { alert(`You clicked on: ${event.target.textContent}`); } }); </script> </body> </html>
6. Building Interactive Elements
6.1 Creating Interactive Buttons
Buttons are a primary means of interactivity on web pages. With event handlers, you can easily add functionality to your buttons.
html <!DOCTYPE html> <html> <head> <title>Interactive Button Example</title> </head> <body> <button id="myButton">Click Me!</button> <script> const button = document.getElementById('myButton'); const handleClick = () => { button.textContent = 'Clicked!'; button.style.backgroundColor = 'green'; }; button.addEventListener('click', handleClick); </script> </body> </html>
6.2 Interactive Forms
Enhancing forms with event handlers can lead to a smoother user experience.
html <!DOCTYPE html> <html> <head> <title>Interactive Form Example</title> </head> <body> <form id="myForm"> <input type="text" id="nameInput" placeholder="Enter your name"> <button type="submit">Submit</button> </form> <script> const form = document.getElementById('myForm'); const nameInput = document.getElementById('nameInput'); const handleSubmit = (event) => { event.preventDefault(); alert(`Hello, ${nameInput.value}! Form submitted successfully.`); form.reset(); }; form.addEventListener('submit', handleSubmit); </script> </body> </html>
6.3 Interactive Image Galleries
Building interactive image galleries can be achieved by utilizing event handlers to change images on user input.
html <!DOCTYPE html> <html> <head> <title>Interactive Image Gallery Example</title> </head> <body> <img src="image1.jpg" id="galleryImage" alt="Image Gallery"> <button id="prevButton">Previous</button> <button id="nextButton">Next</button> <script> const galleryImage = document.getElementById('galleryImage'); const prevButton = document.getElementById('prevButton'); const nextButton = document.getElementById('nextButton'); const images = ['image1.jpg', 'image2.jpg', 'image3.jpg']; let currentIndex = 0; const updateImage = () => { galleryImage.src = images[currentIndex]; }; prevButton.addEventListener('click', () => { currentIndex = (currentIndex - 1 + images.length) % images.length; updateImage(); }); nextButton.addEventListener('click', () => { currentIndex = (currentIndex + 1) % images.length; updateImage(); }); </script> </body> </html>
7. Best Practices and Tips
7.1 Unbinding Event Handlers
Remember to remove event handlers when they are no longer needed to prevent memory leaks and unnecessary event processing.
html <!DOCTYPE html> <html> <head> <title>Unbinding Event Handler Example</title> </head> <body> <button id="myButton">Click Me!</button> <button id="unbindButton">Unbind Event</button> <script> const button = document.getElementById('myButton'); const unbindButton = document.getElementById('unbindButton'); const handleClick = () => { alert('Button clicked!'); }; button.addEventListener('click', handleClick); unbindButton.addEventListener('click', () => { button.removeEventListener('click', handleClick); alert('Event handler unbound!'); }); </script> </body> </html>
7.2 Using Event Delegation Wisely
While event delegation can be efficient, be cautious when using it for events that occur frequently or for elements with deeply nested structures. It may lead to unnecessary event processing.
7.3 Optimizing Event Handling for Performance
When dealing with performance-critical applications, consider throttling or debouncing events to limit the number of times event handlers are triggered.
8. Conclusion
JavaScript event handlers are indispensable tools for creating engaging and interactive web pages. By understanding event types, registering event handlers, and leveraging event propagation, we can build powerful and dynamic user experiences. Moreover, event delegation and best practices ensure our applications are optimized for performance and maintainability. Armed with this knowledge, you are now ready to embark on a journey to create interactive functionality that will captivate your users and elevate your web development skills. Happy coding!
Table of Contents
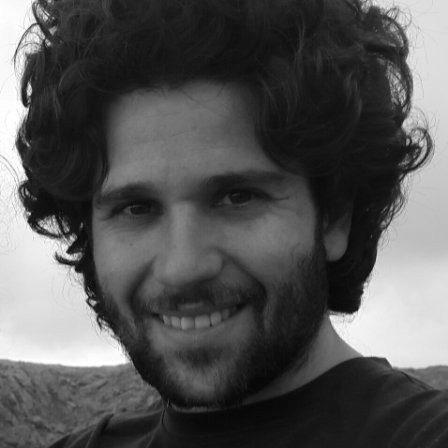
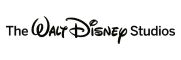