JavaScript Function Factory: Creating Dynamic Functions
JavaScript’s dynamic nature makes it an incredibly versatile language, allowing developers to create flexible and reusable code. One powerful feature is the ability to generate functions dynamically using a pattern known as a “function factory.” This article explores how to leverage function factories in JavaScript to create dynamic functions, providing practical examples and insights into best practices.
Understanding JavaScript Function Factories
A function factory in JavaScript is a function that returns other functions. This pattern is particularly useful for creating multiple similar functions that share common logic but differ in specific behavior. By using function factories, you can reduce redundancy and increase the modularity of your code.
Creating Basic Function Factories
Let’s start with a simple example of a function factory. Imagine you need to create a series of functions that greet users in different languages. Instead of writing each function separately, you can use a function factory to generate them dynamically.
Example: Creating a Greeting Function Factory
```javascript function createGreeting(language) { return function(name) { if (language === 'en') { console.log(`Hello, ${name}!`); } else if (language === 'es') { console.log(`¡Hola, ${name}!`); } else if (language === 'fr') { console.log(`Bonjour, ${name}!`); } else { console.log(`Hello, ${name}!`); } }; } const greetInEnglish = createGreeting('en'); const greetInSpanish = createGreeting('es'); const greetInFrench = createGreeting('fr'); greetInEnglish('John'); // Output: Hello, John! greetInSpanish('Maria'); // Output: ¡Hola, Maria! greetInFrench('Jean'); // Output: Bonjour, Jean! ```
This example demonstrates how a single factory function, `createGreeting`, can generate multiple greeting functions tailored to different languages.
Advanced Use Cases for Function Factories
Function factories can be employed in more complex scenarios, such as creating functions that handle specific business logic or generating event handlers with customized behavior.
Example: Generating Event Handlers
Suppose you’re working on a form that needs to validate different types of inputs. You can create a function factory to generate validation functions for each input type.
```javascript function createValidator(type) { return function(value) { if (type === 'email') { return /\S+@\S+\.\S+/.test(value); } else if (type === 'phone') { return /^\d{10}$/.test(value); } else if (type === 'zip') { return /^\d{5}(-\d{4})?$/.test(value); } else { return false; } }; } const validateEmail = createValidator('email'); const validatePhone = createValidator('phone'); const validateZip = createValidator('zip'); console.log(validateEmail('test@example.com')); // Output: true console.log(validatePhone('1234567890')); // Output: true console.log(validateZip('12345')); // Output: true ```
In this example, `createValidator` produces validation functions for different input types, making the validation process more flexible and reusable.
Handling Context and Closures
Function factories leverage JavaScript closures, where the returned function retains access to the variables defined in the outer function. This characteristic allows the factory to “remember” the parameters passed during its creation, providing powerful context-specific behavior.
Example: Function Factory with Closure
```javascript function createCounter(start) { let count = start; return function() { count += 1; console.log(`Current count: ${count}`); }; } const counterFromTen = createCounter(10); counterFromTen(); // Output: Current count: 11 counterFromTen(); // Output: Current count: 12 const counterFromZero = createCounter(0); counterFromZero(); // Output: Current count: 1 counterFromZero(); // Output: Current count: 2 ```
In this example, each `createCounter` invocation returns a unique counter function with its own internal state, thanks to the closure.
Best Practices for Using Function Factories
While function factories offer great flexibility, it’s essential to use them judiciously to maintain code readability and performance. Here are some best practices:
- Use Descriptive Names: Ensure that your factory functions and the functions they produce have clear, descriptive names to make the code easier to understand.
- Limit Complexity: Avoid over-complicating factories with too many conditional branches or side effects. Aim for simplicity and clarity.
- Document Behavior: Clearly document the expected input and output of your factory functions, especially when dealing with complex scenarios.
- Test Thoroughly: Since function factories can generate numerous functions with varying behavior, it’s crucial to test them thoroughly to ensure they work as intended.
Conclusion
JavaScript function factories are a powerful tool for creating dynamic and reusable functions, enabling more flexible and maintainable code. By understanding how to create and use these factories effectively, you can streamline your development process and enhance the adaptability of your applications.
Further Reading:
Table of Contents
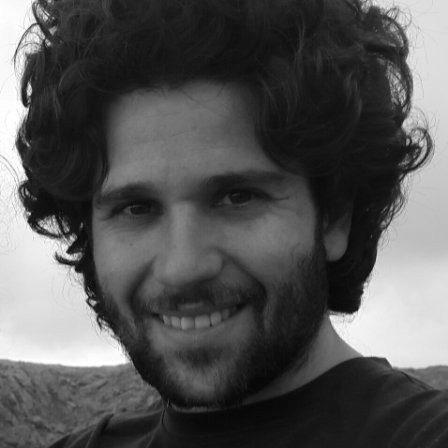
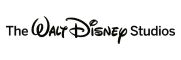