Harnessing the Flexibility of JavaScript First-Class Functions
JavaScript, the versatile and widely-used programming language, has been the backbone of the web for decades. Its powerful features, one of which is first-class functions, have allowed developers to write more elegant and flexible code. Understanding and harnessing the potential of first-class functions is essential for any JavaScript developer aiming to excel in their craft.
Table of Contents
In this blog, we will explore the concept of first-class functions, their significance in JavaScript, and how they enable functional programming paradigms. We’ll dive into real-world examples to demonstrate how first-class functions can be used to write clean, reusable, and maintainable code.
What are First-Class Functions?
In JavaScript, functions are considered “first-class citizens,” which means they can be treated like any other data type, such as numbers or strings. First-class functions have the following properties:
Assigned to variables:
You can assign functions to variables, making them easier to pass around and use in various contexts.
javascript const add = function (a, b) { return a + b; }; const multiply = function (a, b) { return a * b; };
Passed as arguments:
Functions can be passed as arguments to other functions, allowing for dynamic behavior and higher-order functions.
javascript function calculate(operation, a, b) { return operation(a, b); } const result1 = calculate(add, 5, 3); // Returns 8 const result2 = calculate(multiply, 5, 3); // Returns 15
Returned from other functions:
Functions can return other functions, which enables function composition and advanced programming techniques.
javascript function createAdder(a) { return function (b) { return a + b; }; } const addFive = createAdder(5); const result = addFive(3); // Returns 8
The Power of Higher-Order Functions
Higher-order functions are functions that take one or more functions as arguments or return a function. JavaScript’s ability to work with higher-order functions, thanks to first-class functions, is a powerful tool in a developer’s arsenal. Let’s explore some common use cases.
Callbacks
Callbacks are functions passed as arguments to other functions and are executed after the completion of a specific task. They are widely used in asynchronous programming, event handling, and more.
javascript function fetchDataFromServer(callback) { // Simulating asynchronous data fetching setTimeout(() => { const data = { name: "John", age: 30 }; callback(data); }, 1000); } function processData(data) { console.log("Processing data:", data); } fetchDataFromServer(processData);
Array Methods
Higher-order functions are heavily used with arrays, providing concise ways to manipulate and process data.
javascript const numbers = [1, 2, 3, 4, 5]; const squaredNumbers = numbers.map((num) => num ** 2); console.log(squaredNumbers); // [1, 4, 9, 16, 25] const sum = numbers.reduce((acc, num) => acc + num, 0); console.log(sum); // 15 const evenNumbers = numbers.filter((num) => num % 2 === 0); console.log(evenNumbers); // [2, 4]
Currying
Currying is a technique where a function is transformed into a sequence of functions, each taking a single argument. It allows partial application and enhances code reusability.
javascript function multiplyThreeNumbers(a, b, c) { return a * b * c; } const curriedMultiply = (a) => (b) => (c) => a * b * c; const multiplyByTwo = curriedMultiply(2); const multiplyByThree = multiplyByTwo(3); const result = multiplyByThree(4); // Returns 24
Functional Programming Paradigm
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions. JavaScript’s support for first-class functions makes it an excellent language for embracing functional programming concepts.
Immutability
Functional programming encourages immutability, where data is not modified directly. Instead, new data is created, and the old data remains unchanged.
javascript // Non-functional approach let balance = 1000; function deposit(amount) { balance += amount; // Modifies the original balance } deposit(100); console.log(balance); // 1100 // Functional approach const initialBalance = 1000; function deposit(balance, amount) { return balance + amount; // Creates a new balance without modifying the original } const newBalance = deposit(initialBalance, 100); console.log(newBalance); // 1100
Pure Functions
Pure functions are functions that always produce the same output for the same input and have no side effects. They play a crucial role in functional programming, making code easier to test, reason about, and parallelize.
javascript // Impure function let taxRate = 0.2; function calculateTax(amount) { return amount * (1 + taxRate); // Depends on external taxRate variable } console.log(calculateTax(100)); // Returns 120 taxRate = 0.3; console.log(calculateTax(100)); // Now returns 130 // Pure function function calculateTax(amount, taxRate) { return amount * (1 + taxRate); // Pure function with no external dependencies } console.log(calculateTax(100, 0.2)); // Returns 120 console.log(calculateTax(100, 0.3)); // Returns 130
Function Composition
Function composition involves combining functions to create new functions, promoting code reuse and modularization.
javascript function add(a, b) { return a + b; } function square(num) { return num ** 2; } const addAndSquare = (a, b) => square(add(a, b)); console.log(addAndSquare(2, 3)); // Returns 25
Conclusion
JavaScript’s first-class functions provide developers with a powerful toolset to create flexible and expressive code. By understanding higher-order functions and functional programming paradigms, you can enhance your code quality, improve maintainability, and build more scalable applications.
In this blog, we have only scratched the surface of what you can achieve with first-class functions. As you continue your journey with JavaScript, embrace functional programming principles, and leverage the full potential of first-class functions to become a more proficient and efficient JavaScript developer. Happy coding!
Table of Contents
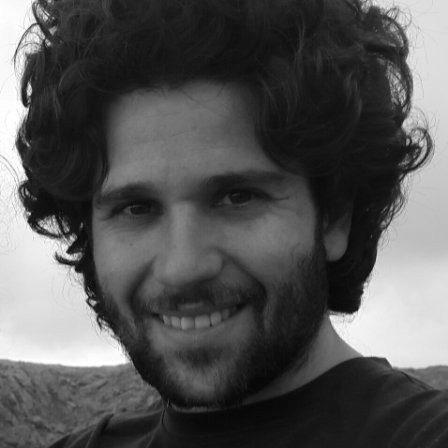
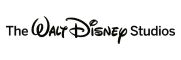