Understanding JavaScript Function Context: this Keyword Explained
When it comes to JavaScript, the this keyword is both essential and occasionally confounding. It’s a powerful feature that allows you to access the context within which a function is executed. However, it can also lead to confusion due to its dynamic nature. In this article, we’ll delve into the world of JavaScript function context and unravel the mysteries behind the this keyword.
Table of Contents
1. What is the ‘this’ Keyword?
In JavaScript, the this keyword refers to the object that a function is currently bound to, or the object that is currently being worked on within the function. It provides a way to access the properties and methods of the current object. The value of this changes based on how a function is called, making it a versatile tool with various use cases.
2. Default Binding
When a function is invoked without any context, the this keyword defaults to the global object in non-strict mode. In a browser environment, the global object is usually the window object. Let’s take a look at an example:
javascript function greet() { console.log(`Hello, ${this.name}!`); } name = "John"; greet(); // Output: Hello, John!
In this case, the function greet is called without an explicit context, so this refers to the global object where the name property is defined.
3. Object Method Invocation
When a function is called as a method of an object, this points to the object itself. This is a common way to access object properties and methods within a function:
javascript const person = { name: "Alice", greet: function() { console.log(`Hello, ${this.name}!`); } }; person.greet(); // Output: Hello, Alice!
Within the greet method, this refers to the person object, allowing us to access its name property.
4. Explicit Binding
JavaScript provides methods like call, apply, and bind that allow you to explicitly set the value of this for a function. This can be particularly useful when you want to control the context in which a function is executed:
javascript function introduce(lang) { console.log(`I speak ${lang} and my name is ${this.name}.`); } const person1 = { name: "Eva" }; const person2 = { name: "Michael" }; introduce.call(person1, "French"); // Output: I speak French and my name is Eva. introduce.apply(person2, ["Spanish"]); // Output: I speak Spanish and my name is Michael. const greetEva = introduce.bind(person1, "German"); greetEva(); // Output: I speak German and my name is Eva.
In the above code, call and apply directly invoke the introduce function with the specified context. Meanwhile, bind returns a new function with the context bound to person1.
5. Arrow Functions and ‘this’
Arrow functions behave differently when it comes to the this keyword. Unlike regular functions, arrow functions do not have their own this value. Instead, they inherit the this value from their enclosing function or context:
javascript const car = { brand: "Tesla", getInfo: function() { const printBrand = () => { console.log(`Brand: ${this.brand}`); }; printBrand(); } }; car.getInfo(); // Output: Brand: Tesla
Even though printBrand is an arrow function, it still correctly captures the this value from the getInfo method of the car object.
6. Global Context and ‘this’
In the global scope, outside of any function or object, the value of this depends on whether you are running in strict mode or not. In non-strict mode, this refers to the global object (e.g., window in a browser). In strict mode, it defaults to undefined:
javascript "use strict"; console.log(this); // Output: undefined
7. Event Handlers and ‘this’
When a function is used as an event handler, the value of this within that function refers to the element that triggered the event. This can sometimes lead to unexpected behavior, especially when working with nested elements:
javascript const button = document.querySelector("button"); button.addEventListener("click", function() { console.log(this); // Output: [object HTMLButtonElement] });
8. ‘this’ Inside Nested Functions
The behavior of this can become confusing when nested functions are involved. Each nested function has its own this value, which may not necessarily refer to the same object as the outer function’s this:
javascript const outer = { name: "Outer", inner: { name: "Inner", showName: function() { console.log(this.name); } } }; outer.inner.showName(); // Output: Inner
In this case, this within the showName function refers to the inner object, not the outer object.
9. ‘this’ in Constructor Functions
When using constructor functions to create objects, the this keyword refers to the newly created instance of the object:
javascript function Animal(name) { this.name = name; this.speak = function() { console.log(`${this.name} makes a sound.`); }; } const dog = new Animal("Dog"); dog.speak(); // Output: Dog makes a sound.
10. ‘this’ in Callback Functions
Callback functions passed to higher-order functions can also have unpredictable this values. To ensure the correct context, it’s common to store the desired this value in a separate variable:
javascript function Timer() { this.seconds = 0; setInterval(function() { this.seconds++; console.log(this.seconds + " seconds passed."); }.bind(this), 1000); } const timer = new Timer();
Conclusion
Mastering the behavior of the this keyword is crucial for writing effective and maintainable JavaScript code. Understanding how this behaves in different contexts and knowing how to manipulate it when needed empowers you to harness the full potential of this powerful feature. By keeping these concepts in mind and practicing with real-world scenarios, you’ll be well-equipped to navigate the intricate landscape of JavaScript function context and the enigmatic this keyword.
Table of Contents
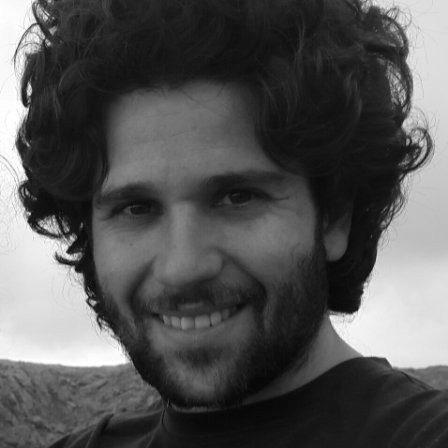
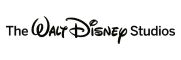