Leveraging JavaScript Function Hoisting for Cleaner Code
In the world of JavaScript, writing clean, efficient, and readable code is an art every developer aspires to master. One of the lesser-known techniques that can significantly improve code readability and organization is function hoisting. Understanding how function hoisting works and leveraging it strategically can lead to cleaner code and more maintainable projects.
Table of Contents
In this blog post, we will explore the concept of function hoisting, its benefits, and how you can use it to your advantage. We will cover various examples and scenarios where hoisting can be applied to enhance code readability and optimize variable declarations.
What is Function Hoisting?
Function hoisting is a unique behavior in JavaScript that allows functions to be declared anywhere in the scope and still be accessible from anywhere within that scope. Unlike variables, which are hoisted but not initialized, functions are hoisted and initialized during the compilation phase. This means that you can use a function before it is declared in your code.
Consider the following example:
javascript sayHello(); // Output: "Hello, there!" function sayHello() { console.log("Hello, there!"); }
In this example, we call the sayHello() function before declaring it, yet it works perfectly fine. That’s because during the compilation phase, JavaScript moves the function declaration to the top of the scope, making it accessible from any point within that scope.
The Benefits of Function Hoisting
Function hoisting offers several advantages, including:
1. Enhanced Readability and Structure
By using function hoisting, you can declare your functions near where they are logically used, without worrying about the order. This can greatly improve the readability of your code and make it easier for you and other developers to understand the flow of the program.
2. Optimal Variable Declarations
In JavaScript, variables declared with the var keyword are hoisted to the top of their scope and are initialized with the value undefined. By leveraging function hoisting, you can place your function declarations above your variable declarations, resulting in a more organized and cleaner codebase.
3. Avoiding Reference Errors
One common issue in JavaScript is accidentally trying to call a function before it is declared, which leads to reference errors. Function hoisting eliminates this problem, ensuring that all your functions are available and ready for use, regardless of their actual placement in the code.
4. Encouraging Modularity
Function hoisting encourages you to break down your code into smaller, reusable functions. You can declare these functions anywhere within their scope, promoting modularity and making your codebase more maintainable and scalable.
Scenarios and Examples
Let’s explore some common scenarios and examples where function hoisting can be effectively utilized to achieve cleaner code and improve code structure.
1. Function Expressions vs. Function Declarations
In JavaScript, you can define functions using function expressions and function declarations. When using function declarations, hoisting comes into play, but function expressions behave differently.
Consider the following example:
javascript // Function Declaration (Hoisted) sayHello(); // Output: "Hello, there!" function sayHello() { console.log("Hello, there!"); } // Function Expression (Not Hoisted) sayHi(); // Throws a ReferenceError var sayHi = function() { console.log("Hi!"); };
In the example above, the function declaration sayHello() works as expected because of hoisting. However, the function expression sayHi() results in a ReferenceError since the variable sayHi is hoisted but initialized as undefined, and it’s not yet assigned a function.
To avoid issues with function expressions, always declare them before use, or prefer using function declarations for more readable and organized code.
2. Function Hoisting within Blocks
In JavaScript, hoisting occurs within function scopes and global scopes. However, hoisting within block scopes (e.g., inside if or for statements) works differently.
Consider the following example:
javascript function example() { console.log(a); // Output: undefined if (true) { var a = 10; } console.log(a); // Output: 10 } example();
In this example, the variable a is hoisted within the function scope. However, due to hoisting, the first console.log(a) outputs undefined since a is hoisted but not yet assigned a value. The assignment var a = 10; happens afterward.
To avoid confusion and maintain cleaner code, it is recommended to declare variables at the beginning of their scope, even though they are hoisted.
3. Leveraging Hoisting for Utility Functions
Function hoisting can be particularly useful when declaring utility functions that are used across your application. By hoisting these functions, you can place them anywhere within the scope, closer to their relevant usages.
Consider the following example:
javascript function generateUniqueId() { // Logic to generate a unique ID } function processData(data) { if (dataIsValid(data)) { data.id = generateUniqueId(); // Process the data further } } function dataIsValid(data) { // Logic to validate data } // Rest of the code that uses the processData function
In this example, generateUniqueId() and dataIsValid() are hoisted, making them accessible anywhere within their scope. Placing them at the top of the scope, even before their usages, promotes readability and helps other developers understand their importance in the context of the application.
Conclusion
Function hoisting is a powerful feature of JavaScript that allows functions to be declared anywhere within a scope and still be accessible throughout that scope. Leveraging function hoisting can significantly enhance the readability and maintainability of your codebase. By declaring functions closer to their relevant usages, you can improve code structure and organization, making your code cleaner and more efficient.
Remember to prefer function declarations over function expressions to take advantage of hoisting fully. Additionally, when working within block scopes, declare variables at the beginning of the scope to avoid confusion due to hoisting.
By mastering the art of function hoisting, you can take your JavaScript coding skills to the next level and become a more effective and efficient developer. Happy coding!
Table of Contents
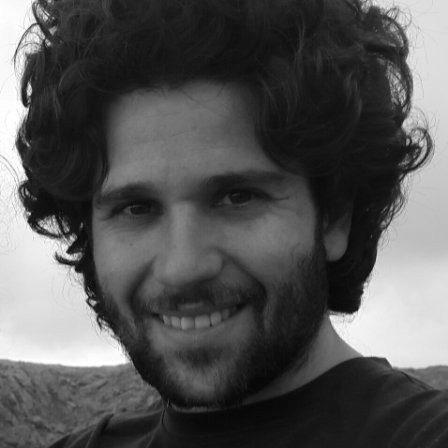
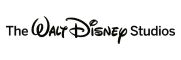