Embracing the Functional Style: A Comprehensive Guide to Functional Programming with JavaScript
Functional programming is a programming paradigm that emphasizes the use of functions and mathematical concepts. It is rooted in the idea that functions should be first-class citizens, allowing them to be passed as arguments, returned from other functions, and assigned to variables. By applying functional programming principles, you can write more readable, maintainable, and less error-prone code.
JavaScript, initially designed for client-side web development, has blossomed into a versatile, full-stack programming language. It incorporates aspects of many programming paradigms, including functional programming. This flexibility is one reason why JavaScript has remained so popular among developers and why companies frequently choose to hire JavaScript developers.
This blog post aims to introduce the fundamental concepts of functional programming and demonstrate how you can apply these principles using JavaScript. Furthermore, it can serve as a guide for those looking to hire JavaScript developers, offering insight into the skill set that such developers should possess to successfully implement functional programming principles.
Concepts of Functional Programming
Before diving into examples, let’s highlight some key concepts:
- Pure Functions: These are functions where the return value is solely dependent on its input values, without any side effects.
- First-Class Functions: In JavaScript, functions are first-class objects. This means that they can be passed as arguments, returned by other functions, and assigned to variables.
- Higher-Order Functions: These are functions that take one or more functions as arguments, return a function, or both.
- Immutability: This concept requires that data or states are not changed once created. Instead, new data is returned.
- Function Composition: This is the process of combining two or more functions to create a new function.
Using Functional Programming in JavaScript
Let’s discuss how to implement these concepts in JavaScript.
1. Pure Functions
Pure functions return the same output for the same input without causing side effects. Here’s an example:
```javascript function squareNumber(num) { return num * num; } ```
In this function, the output is purely dependent on the input. It doesn’t change or affect anything outside of the function.
2. First-Class Functions
JavaScript treats functions as first-class citizens, which allows us to assign functions to variables, pass them as arguments to other functions, or return them as values from other functions.
```javascript let greet = function(name) { return `Hello, ${name}!`; } function sayHello(greet, name) { console.log(greet(name)); } sayHello(greet, "John"); // Outputs: Hello, John! ```
In the above example, the function ‘greet’ is assigned to a variable and is also passed as an argument to the ‘sayHello’ function.
3. Higher-Order Functions
Higher-order functions either take functions as arguments, return functions, or do both. JavaScript array methods like ‘filter’, ‘map’, and ‘reduce’ are examples of higher-order functions.
Here’s an example using the ‘map’ method:
```javascript let numbers = [1, 2, 3, 4, 5]; let squaredNumbers = numbers.map(num => num * num); console.log(squaredNumbers); // Outputs: [1, 4, 9, 16, 25] ```
In the example above, ‘map’ is a higher-order function that takes a function (num => num * num) as an argument.
4. Immutability
Immutability is an essential concept in functional programming. When data is immutable, its state cannot change after it’s created. If you want to change an immutable object, you don’t modify it. Instead, you create a new object with the new value.
In JavaScript, we usually use ‘const’ to declare variables that cannot be re-assigned.
```javascript const PI = 3.14; ```
In the above example, the value of PI cannot be changed once it’s declared.
5. Function Composition
Function composition is the process of combining two or more functions to produce a new function.
Here’s an example:
```javascript function add(a, b) { return a + b; } function square(num) { return num * num; } let squareOfSum = square(add(2, 3)); console.log(squareOfSum); // Outputs: 25 ```
In the above example, the ‘add’ function is first executed and then its result is passed to the ‘square’ function.
Conclusion
Functional programming brings a different perspective to software development by emphasizing immutability, pure functions, and function composition. With JavaScript’s support for functional programming concepts, developers can write cleaner, more maintainable code.
Hiring JavaScript developers who are adept in functional programming can provide a significant boost to your software development process. These developers can leverage their knowledge of functional concepts to build robust applications, making them a valuable asset for any team.
Although it may take time for your hired JavaScript developers to become comfortable with functional programming principles, the effort will undoubtedly pay off in their JavaScript coding. So next time you find yourself wrestling with complex state management or tangled code, consider a functional programming approach, and harness the power of JavaScript functions. This also underscores the importance of hiring JavaScript developers who are well-versed with such advanced programming concepts.
Table of Contents
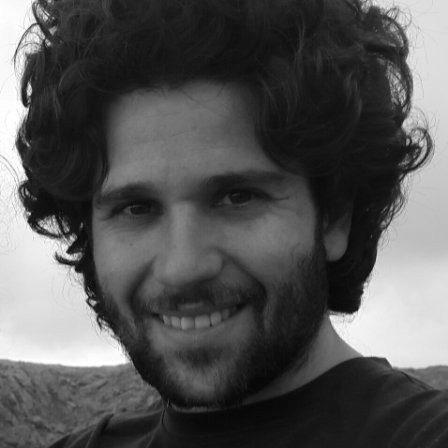
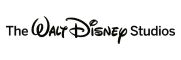