JavaScript Explained: The Distinctions and Use Cases of Functions vs. Methods
In the world of JavaScript, functions and methods are two of the most vital aspects. If you’ve written or read JavaScript code, you’ve undoubtedly encountered both functions and methods, perhaps without even knowing the difference. This knowledge becomes all the more critical when you’re looking to hire JavaScript developers, as understanding these fundamental concepts is key to writing efficient and robust code.
While functions and methods may seem identical and are sometimes used interchangeably, they have subtle differences. This blog post aims to delve into the distinction between JavaScript functions and methods with numerous examples, providing valuable insights for anyone looking to deepen their JavaScript knowledge or intending to hire JavaScript developers with a solid grasp of these crucial aspects.
1. Understanding Functions in JavaScript
Functions are one of the fundamental building blocks in JavaScript. A function is a set of statements that performs a specific task or calculates a value.
1.1 Syntax for a Basic Function
A basic function in JavaScript can be defined as follows:
```javascript function name(parameter1, parameter2, parameter3) { // code to be executed } ```
The function keyword starts the definition, followed by the function name. We then have the parameters within the parentheses (parameter1, parameter2, …). A function can have as many or as few parameters as needed. Finally, the function’s code is written between the curly braces.
Example of a JavaScript Function
Let’s consider a simple JavaScript function named `greet`:
```javascript function greet(name) { return 'Hello, ' + name + '!'; } console.log(greet('John')); // Outputs: Hello, John! ```
In the example above, the function `greet` is declared with one parameter `name`. When the function is called, it returns the greeting string.
2. Understanding Methods in JavaScript
In JavaScript, almost everything is an object: strings, numbers, arrays, dates, and so on. Each object has a set of properties and methods. A method in JavaScript is a function that’s a property of an object.
2.1 Syntax for a Basic Method
Here’s how a method is typically defined in an object:
```javascript let obj = { propertyName: function(parameter1, parameter2) { // code to be executed } } ```
In this case, `propertyName` is the method of the object `obj`. We define the function as a property of the object.
Example of a JavaScript Method
Here’s a simple JavaScript method:
```javascript let person = { firstName: 'John', lastName: 'Doe', fullName: function() { return this.firstName + ' ' + this.lastName; } } console.log(person.fullName()); // Outputs: John Doe ```
In the example above, `fullName` is a method of the `person` object. `this` is a keyword in JavaScript that refers to the object that the method is a part of.
3. The Crucial Difference Between Functions and Methods
The primary difference between a function and a method in JavaScript is the way they are called and how they access data.
A function is independent, meaning that it can be called anytime and anywhere in your program. It also does not have automatic access to other parts of your code outside its scope.
On the other hand, a method is tied to the object that it’s a part of. A method can access other properties of that object using the `this` keyword.
This means that while both functions and methods perform actions, methods are always associated with a specific object.
4. The Blurred Line: Functions as Object Methods
The distinction between functions and methods in JavaScript can sometimes get blurred because functions can be methods when they’re properties of an object.
Here’s an example:
```javascript let greet = { sayHello: function(name) { return 'Hello, ' + name + '!'; } } console.log(greet.sayHello('John')); // Outputs: Hello, John! ```
In the above example, the function `sayHello` is a property of the `greet` object, making it a method.
5. Using `this` in Functions vs. Methods
Another key difference between JavaScript functions and methods lies in the use of `this`.
In a method, `this` refers to the owner object. It’s a way for the method to access other properties of the object. For example:
```javascript let person = { firstName: 'John', lastName: 'Doe', fullName: function() { return this.firstName + ' ' + this.lastName; } } console.log(person.fullName()); // Outputs: John Doe ```
In the `fullName` method, `this.firstName` and `this.lastName` refer to the `firstName` and `lastName` properties of the `person` object.
In a standalone function, however, `this` does not refer to the global object (in browsers, this is the `window` object) but is `undefined` in strict mode. For example:
```javascript 'use strict'; function showThis() { console.log(this); } showThis(); // Outputs: undefined ```
In the function `showThis`, `this` does not refer to any object, so it is `undefined`.
Final Thoughts
Understanding the difference between functions and methods in JavaScript is a crucial skill for both novice and seasoned developers. It significantly improves your code’s organization, readability, and performance. This knowledge is especially important when you’re looking to hire JavaScript developers, as their grasp of these concepts is a strong indicator of their proficiency and attention to detail.
Functions and methods are both indispensable in JavaScript, but knowing when and how to use each will empower developers to write more efficient and effective code. In essence, a function is a set of statements designed to perform a particular task, whereas a method is a function associated with an object and can access the object’s properties.
In the dynamic world of JavaScript, it’s interesting to note that functions can also become methods when they are properties of an object. So, the next time you’re coding in JavaScript, or when you’re about to hire JavaScript developers, keep these differences in mind. It will not only enhance your code’s efficiency but also help you evaluate the depth of understanding of potential developers.
Table of Contents
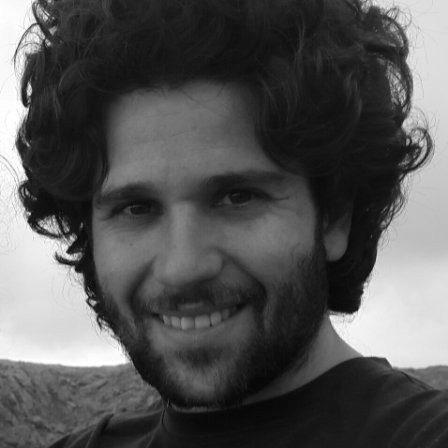
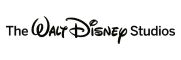