From Basics to Advanced: A Complete Guide to Master JavaScript Functions
JavaScript functions are one of the most essential components of the language, a fact well-known by companies looking to hire JavaScript developers. These functions allow us to build complex applications and simplify our code by avoiding repetition and enhancing readability. This guide is designed to take you from the basics of JavaScript functions to more advanced concepts, much like the journey one would take when training to become a top-tier candidate for organizations seeking to hire JavaScript developers. By the end, you will have a comprehensive understanding of JavaScript functions, ready to use in real-world programming tasks and proficient enough to confidently apply when companies are looking to hire JavaScript developers.
Introduction to JavaScript Functions
A JavaScript function is a block of code designed to perform a particular task. It is executed when it has been invoked (called). A function can be defined using the function keyword, followed by a name, parentheses `()`—which may include function parameters—and the code block to be executed, enclosed within curly brackets `{}`.
```javascript function greet() { console.log("Hello, world!"); } greet(); // Calls the function, prints: "Hello, world!" ```
The `greet` function takes no arguments, and when called, it simply prints a greeting to the console. Functions can also take arguments and use them to perform operations:
```javascript function greet(name) { console.log("Hello, " + name + "!"); } greet("Alice"); // Calls the function, prints: "Hello, Alice!" ```
In this case, the `greet` function takes one argument: `name`. When we call the function, we provide the argument, which the function then uses to print a customized greeting.
Returning Values
While the above functions perform an action, they do not provide any result that can be used later in the code. This is where the `return` statement comes into play. The `return` statement ends function execution and specifies a value to be returned to the function caller.
```javascript function add(num1, num2) { return num1 + num2; } let sum = add(5, 3); console.log(sum); // Prints: 8 ```
Function Expressions
In JavaScript, functions can also be defined using expressions. A function expression can be stored in a variable. Once the function is stored in a variable, the variable can be used as a function. Functions stored in variables do not need function names. They are always invoked (called) using the variable name.
```javascript let greet = function() { console.log("Hello, world!"); } greet(); // Calls the function, prints: "Hello, world!" ```
Arrow Functions
ES6 introduced a new way of writing concise functions, called arrow functions. They provide a shorter syntax than function expressions and do not rebind `this`.
```javascript let greet = () => { console.log("Hello, world!"); } greet(); // Calls the function, prints: "Hello, world!" ```
Arrow functions also simplify functions that take arguments or return a value:
```javascript // A function that takes arguments let add = (num1, num2) => { return num1 + num2; } console.log(add(5, 3)); // Prints: 8 // Even shorter way for a function that returns a value let multiply = (num1, num2) => num1 * num2; console.log(multiply(5, 3)); // Prints: 15 ```
Higher-Order Functions
Higher-order functions are functions that take other functions as arguments, return a function as a result, or both. They are a significant part of functional programming in JavaScript and provide a powerful way to abstract and manage complexity in your code.
Here’s an example of a higher-order function that takes a function as an argument:
```javascript function greet(name, formatter) { return "Hello, " + formatter(name); } function upperCaseName(name) { return name.toUpperCase(); } console.log(greet("Alice", upperCaseName)); // Prints: "Hello, ALICE" ```
In this example, `greet` is a higher-order function that takes a function `formatter` as an argument. `upperCaseName` is passed as an argument, which converts the name to uppercase.
Callback Functions
A callback function is a function passed into another function as an argument, which is then invoked inside the outer function. Callbacks are frequently used in JavaScript for event handlers and timers.
Here’s an example using the `setTimeout` function, which executes a callback function after a specified delay:
```javascript function greet() { console.log("Hello, world!"); } setTimeout(greet, 2000); // Calls greet after 2 seconds ```
Closures
A closure in JavaScript is a function that has access to the parent scope, even after the parent function has closed. This is a powerful feature that enables data privacy and function factories.
Here’s an example of a closure:
```javascript function outer() { let x = 10; function inner() { console.log(x); // Has access to x from outer function } return inner; } let innerFunc = outer(); innerFunc(); // Prints: 10 ```
Conclusion
Mastering JavaScript functions is a crucial step in becoming a proficient JavaScript developer, a quality that is essential when looking to hire JavaScript developers. From defining simple functions to understanding advanced concepts like higher-order functions and closures, this guide provides a comprehensive look at JavaScript functions. As you keep practicing and experimenting with these concepts, you’ll see how they can make your code more efficient, readable, and maintainable, which are important qualities for a potential JavaScript developer hire. Happy coding!
Table of Contents
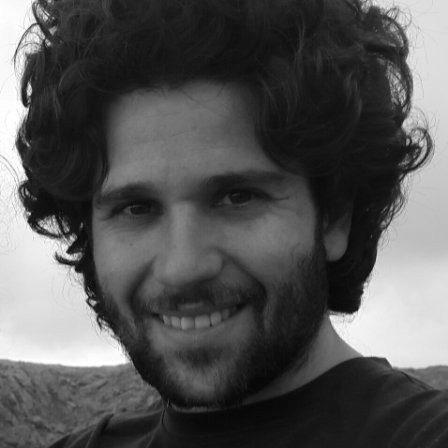
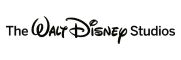